i.安装设备后从设备中读出字符串为自己学号的后3位; ii. 设备支持每次写入字符不超过1024个,超过部分被丢弃,并且能够保存最近一次修改前的rwbuf里的内容。 iii. 设备支持系统调用ioctl(int d, int req,…),共支持设置三种模式:a. 清除设备中写入的字符串; b. 从设备中读时,读出的是最近一次修改前的内容。 c. 从设备中读时,读出的是最新内容 iv. 设备关闭前不能被多次打开; v. 自己编写测试程序,验证以上功能
时间: 2024-02-13 18:02:29 浏览: 50
好的,您的问题大致是关于一个设备的读写和控制。我会尽力回答您的问题。
首先,对于第一个问题,您需要通过设备读取出自己学号的后3位。这可以通过设备文件的读操作来实现。您需要打开设备文件并使用read()函数从设备中读取数据,然后从读取的数据中提取出后3位即可。示例代码如下:
```c
int fd = open("/dev/device_file", O_RDONLY); // 打开设备文件
char buf[1024];
read(fd, buf, 1024); // 从设备中读取数据
close(fd); // 关闭设备文件
// 从读取的数据中提取出后3位
char last_three_digits[4];
memcpy(last_three_digits, &buf[strlen(buf) - 3], 3);
last_three_digits[3] = '\0';
printf("My last three digits of student ID are: %s\n", last_three_digits);
```
对于第二个问题,设备支持每次写入字符不超过1024个,超过部分被丢弃,并且能够保存最近一次修改前的rwbuf里的内容。这可以通过设备文件的写操作来实现。您需要打开设备文件并使用write()函数向设备中写入数据,然后设备会将最近一次修改前的rwbuf里的内容保存下来。示例代码如下:
```c
int fd = open("/dev/device_file", O_WRONLY); // 打开设备文件
char buf[1024] = "Hello, this is a test message.";
write(fd, buf, strlen(buf)); // 向设备中写入数据
memset(buf, 0, sizeof(buf)); // 清空缓冲区
read(fd, buf, 1024); // 从设备中读取数据
close(fd); // 关闭设备文件
printf("Read from device: %s\n", buf); // 输出最近一次修改前的内容
```
对于第三个问题,设备支持系统调用ioctl(int d, int req,…),共支持设置三种模式:a. 清除设备中写入的字符串; b. 从设备中读时,读出的是最近一次修改前的内容。 c. 从设备中读时,读出的是最新内容。这可以通过ioctl系统调用来实现。示例代码如下:
```c
int fd = open("/dev/device_file", O_RDWR); // 打开设备文件
ioctl(fd, CLEAR_DEVICE, NULL); // 清除设备中写入的字符串
char buf[1024];
ioctl(fd, READ_LAST_CONTENT, buf); // 从设备中读取最近一次修改前的内容
printf("Read from device (last content): %s\n", buf);
ioctl(fd, READ_NEW_CONTENT, buf); // 从设备中读取最新内容
printf("Read from device (new content): %s\n", buf);
close(fd); // 关闭设备文件
```
对于第四个问题,设备关闭前不能被多次打开,这可以通过使用一个全局变量来实现。示例代码如下:
```c
static int device_open_count = 0; // 全局变量,记录设备打开次数
// 设备文件的open()函数
static int device_open(struct inode *inode, struct file *file)
{
if (device_open_count > 0) {
return -EBUSY; // 设备已经被打开,返回EBUSY错误码
}
device_open_count++;
// ...
return 0; // 打开成功
}
// 设备文件的release()函数
static int device_release(struct inode *inode, struct file *file)
{
device_open_count--;
// ...
return 0;
}
```
最后,对于第五个问题,您需要编写一个测试程序来验证以上功能。下面是一个简单的测试程序的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <fcntl.h>
#include <unistd.h>
#include <sys/ioctl.h>
#define DEVICE_FILE "/dev/device_file"
#define CLEAR_DEVICE 0x01
#define READ_LAST_CONTENT 0x02
#define READ_NEW_CONTENT 0x03
int main()
{
// 测试读取设备中的学号后3位
int fd = open(DEVICE_FILE, O_RDONLY);
char buf[1024];
read(fd, buf, 1024);
close(fd);
char last_three_digits[4];
memcpy(last_three_digits, &buf[strlen(buf) - 3], 3);
last_three_digits[3] = '\0';
printf("My last three digits of student ID are: %s\n", last_three_digits);
// 测试向设备中写入数据并读取最近一次修改前的内容
fd = open(DEVICE_FILE, O_WRONLY);
char msg[1024] = "Hello, this is a test message.";
write(fd, msg, strlen(msg));
memset(buf, 0, sizeof(buf));
ioctl(fd, READ_LAST_CONTENT, buf);
printf("Read from device (last content): %s\n", buf);
close(fd);
// 测试清除设备中的字符串和读取最新内容
fd = open(DEVICE_FILE, O_RDWR);
ioctl(fd, CLEAR_DEVICE, NULL);
memset(buf, 0, sizeof(buf));
ioctl(fd, READ_NEW_CONTENT, buf);
printf("Read from device (new content): %s\n", buf);
close(fd);
return 0;
}
```
以上就是我对您的问题的回答。希望能够对您有所帮助。
阅读全文
相关推荐
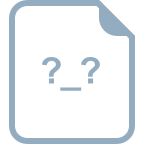
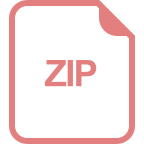
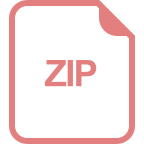












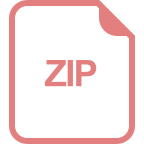