用c语言写出stm32串口dma驱动程序框架
时间: 2023-05-25 17:05:11 浏览: 73
#include "stm32f10x.h"
#define USART_DR_ADDRESS ((uint32_t)USART1_BASE + 0x04)
void USART1_DMA_Init(void)
{
/* Enable DMA1 clock */
RCC_AHBPeriphClockCmd(RCC_AHBPeriph_DMA1, ENABLE);
/* Initialize DMA1 channel 5, which is connected to USART1 Tx */
DMA_InitTypeDef DMA_InitStruct;
DMA_StructInit(&DMA_InitStruct);
DMA_InitStruct.DMA_PeripheralBaseAddr = USART_DR_ADDRESS;
DMA_InitStruct.DMA_MemoryBaseAddr = (uint32_t)NULL;
DMA_InitStruct.DMA_DIR = DMA_DIR_PeripheralDST;
DMA_InitStruct.DMA_BufferSize = 0;
DMA_InitStruct.DMA_PeripheralInc = DMA_PeripheralInc_Disable;
DMA_InitStruct.DMA_MemoryInc = DMA_MemoryInc_Enable;
DMA_InitStruct.DMA_PeripheralDataSize = DMA_PeripheralDataSize_Byte;
DMA_InitStruct.DMA_MemoryDataSize = DMA_MemoryDataSize_Byte;
DMA_InitStruct.DMA_Mode = DMA_Mode_Normal;
DMA_InitStruct.DMA_Priority = DMA_Priority_Medium;
DMA_InitStruct.DMA_M2M = DMA_M2M_Disable;
DMA_Init(DMA1_Channel5, &DMA_InitStruct);
DMA_ITConfig(DMA1_Channel5, DMA_IT_TC, ENABLE);
/* Enable USART1 clock */
RCC_APB2PeriphClockCmd(RCC_APB2Periph_USART1, ENABLE);
/* Initialize USART1 */
USART_InitTypeDef USART_InitStruct;
USART_StructInit(&USART_InitStruct);
USART_InitStruct.USART_BaudRate = 9600;
USART_Init(USART1, &USART_InitStruct);
/* Enable USART1 DMA request, so that USART1 data will be sent through DMA */
USART_DMACmd(USART1, USART_DMAReq_Tx, ENABLE);
/* Enable USART1 */
USART_Cmd(USART1, ENABLE);
/* Enable DMA1 channel 5 interrupt, so that we know that the data has been sent */
NVIC_InitTypeDef NVIC_InitStruct;
NVIC_InitStruct.NVIC_IRQChannel = DMA1_Channel5_IRQn;
NVIC_InitStruct.NVIC_IRQChannelPreemptionPriority = 0;
NVIC_InitStruct.NVIC_IRQChannelSubPriority = 0;
NVIC_InitStruct.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init(&NVIC_InitStruct);
}
void USART1_SendString(char *str)
{
/* First, get the length of the string */
int len = strlen(str);
/* Set the buffer size for DMA1 channel 5 */
DMA_SetCurrDataCounter(DMA1_Channel5, len);
/* Set the memory address in DMA1 channel 5 */
DMA1_Channel5->CMAR = (uint32_t)str;
/* Start the transfer */
DMA_Cmd(DMA1_Channel5, ENABLE);
}
void DMA1_Channel5_IRQHandler(void)
{
/* Clear the interrupt flag */
DMA_ClearITPendingBit(DMA1_IT_TC5);
/* Disable DMA1 channel 5 */
DMA_Cmd(DMA1_Channel5, DISABLE);
}
相关推荐
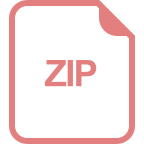
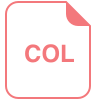
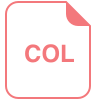
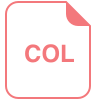
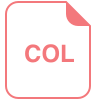
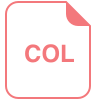









