vue2 有多个已经封装的接口 使用promise.all 结合async await访问多个接口
时间: 2024-02-18 14:00:13 浏览: 87
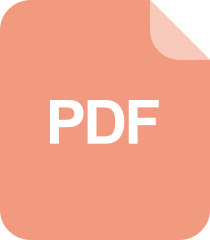
vue-axios同时请求多个接口 等所有接口全部加载完成再处理操作

可以使用`Promise.all`结合`async/await`来访问多个已经封装的接口。以下是一个示例代码:
```javascript
async function fetchData() {
const [result1, result2, result3] = await Promise.all([
api.get('/data1'),
api.get('/data2'),
api.get('/data3')
]);
// 对返回值进行操作
console.log(result1, result2, result3);
}
```
在上述示例中,我们使用`Promise.all`来等待三个异步操作完成,这三个异步操作都是通过调用`api`对象中的`get`方法来进行访问的。最终我们可以得到三个异步操作的返回值,并对其进行操作。需要注意的是,这里使用了数组解构来获取每个异步操作的返回值。
当然,前提是你已经封装好了`api`对象,其中包含了`get`方法,用于访问接口并返回一个`Promise`对象。
阅读全文
相关推荐
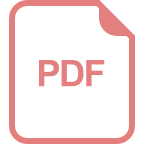

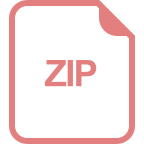
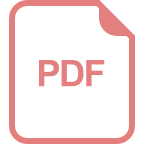
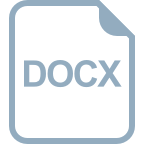
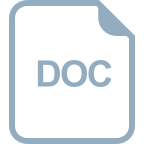










