编写一个用c++的基于激光雷达点云的可行驶区域检测,用pcl库,并说明如何使用,给我代码
时间: 2024-05-22 16:13:10 浏览: 119
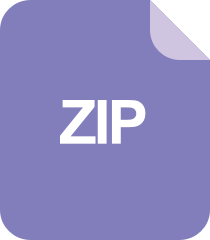
C ++实现,使用LIDAR扫描或点云检测,跟踪和分类多个对象-C/C++开发

以下是一个基于PCL库的激光雷达点云可行驶区域检测的示例代码:
#include <iostream>
#include <pcl/io/pcd_io.h>
#include <pcl/point_types.h>
#include <pcl/filters/voxel_grid.h>
#include <pcl/filters/passthrough.h>
#include <pcl/segmentation/region_growing.h>
#include <pcl/visualization/cloud_viewer.h>
typedef pcl::PointXYZ PointT;
typedef pcl::PointCloud<PointT> PointCloudT;
int main (int argc, char** argv)
{
// Load point cloud data from PCD file
PointCloudT::Ptr cloud (new PointCloudT);
if (pcl::io::loadPCDFile<PointT> ("input.pcd", *cloud) == -1)
{
std::cerr << "Failed to read point cloud data." << std::endl;
return (-1);
}
// Downsample point cloud
pcl::VoxelGrid<PointT> voxel_filter;
voxel_filter.setInputCloud (cloud);
voxel_filter.setLeafSize (0.01f, 0.01f, 0.01f);
PointCloudT::Ptr filtered_cloud (new PointCloudT);
voxel_filter.filter (*filtered_cloud);
// Filter out points outside of specified range
pcl::PassThrough<PointT> pass_filter;
pass_filter.setInputCloud (filtered_cloud);
pass_filter.setFilterFieldName ("z");
pass_filter.setFilterLimits (0.0, 1.0);
PointCloudT::Ptr range_filtered_cloud (new PointCloudT);
pass_filter.filter (*range_filtered_cloud);
// Segment regions of the point cloud
pcl::RegionGrowing<PointT, pcl::Normal> region_growing;
region_growing.setInputCloud (range_filtered_cloud);
region_growing.setMinClusterSize (100);
region_growing.setMaxClusterSize (100000);
pcl::search::Search<PointT>::Ptr tree = boost::shared_ptr<pcl::search::Search<PointT> > (new pcl::search::KdTree<PointT>);
region_growing.setSearchMethod (tree);
pcl::PointCloud<pcl::Normal>::Ptr normals (new pcl::PointCloud<pcl::Normal>);
pcl::NormalEstimation<PointT, pcl::Normal> normal_estimator;
normal_estimator.setInputCloud (range_filtered_cloud);
normal_estimator.setSearchMethod (tree);
normal_estimator.setRadiusSearch (0.03);
normal_estimator.compute (*normals);
region_growing.setInputNormals (normals);
region_growing.setSmoothnessThreshold (5.0 / 180.0 * M_PI);
region_growing.setCurvatureThreshold (1.0);
std::vector<pcl::PointIndices> clusters;
region_growing.extract (clusters);
// Visualize the segmented regions
pcl::visualization::CloudViewer viewer ("Segmented Regions");
PointCloudT::Ptr colored_cloud (new PointCloudT);
pcl::copyPointCloud (*range_filtered_cloud, *colored_cloud);
int j = 0;
for (std::vector<pcl::PointIndices>::const_iterator it = clusters.begin (); it != clusters.end (); ++it)
{
pcl::PointCloud<PointT>::Ptr cloud_cluster (new pcl::PointCloud<PointT>);
for (std::vector<int>::const_iterator pit = it->indices.begin (); pit != it->indices.end (); ++pit)
{
cloud_cluster->points.push_back (colored_cloud->points[*pit]);
}
cloud_cluster->width = cloud_cluster->points.size ();
cloud_cluster->height = 1;
cloud_cluster->is_dense = true;
// Color the cluster with a different RGB value
int r = rand() % 255;
int g = rand() % 255;
int b = rand() % 255;
for (std::vector<int>::const_iterator pit = it->indices.begin (); pit != it->indices.end (); ++pit)
{
colored_cloud->points[*pit].r = r;
colored_cloud->points[*pit].g = g;
colored_cloud->points[*pit].b = b;
}
j++;
}
viewer.showCloud (colored_cloud);
return (0);
}
该示例代码的实现过程如下:
1. 加载点云数据:使用pcl::io::loadPCDFile()函数从PCD文件中读取点云数据。
2. 下采样点云:使用pcl::VoxelGrid滤波器将点云降采样以减少计算量。
3. 过滤点云范围:使用pcl::PassThrough滤波器过滤掉点云数据中指定范围外的点。
4. 分割点云区域:使用pcl::RegionGrowing分割点云数据中的可行驶区域。
5. 可视化分割结果:使用pcl::visualization::CloudViewer可视化分割结果。
使用该示例代码需要注意以下几点:
1. 确保已安装PCL库并正确配置了编译环境。
2. 将示例代码中的“input.pcd”替换为实际的点云数据文件名。
3. 可根据需要调整各个滤波器和分割器的参数以达到更好的效果。
4. 示例代码中的可视化仅适用于单个分割结果,如需可视化多个分割结果可采用其他方法。
阅读全文
相关推荐















