决策树分箱实现 python
时间: 2023-06-29 11:06:35 浏览: 55
决策树分箱是一种常用的数据预处理方法,可以将连续型特征离散化为分类型特征,以便于机器学习算法的使用。下面是一个简单的 Python 实现:
```python
import pandas as pd
from sklearn.tree import DecisionTreeClassifier
def binning(data, feature, target, max_depth):
# 将 feature 和 target 从数据中提取出来
X = data[[feature]]
y = data[target]
# 使用决策树分类器进行分箱
dt = DecisionTreeClassifier(max_depth=max_depth)
dt.fit(X, y)
# 提取出决策树中的阈值点
thresholds = sorted(dt.tree_.threshold[dt.tree_.threshold != -2])
# 将阈值点插入到数据中,得到分箱后的数据
result = pd.cut(X[feature], [-float("inf")] + thresholds + [float("inf")], labels=False)
return result
# 示例
data = pd.DataFrame({"age": [20, 25, 30, 35, 40, 45, 50, 55, 60],
"income": [10, 20, 30, 40, 50, 60, 70, 80, 90],
"label": [0, 0, 0, 0, 1, 1, 1, 1, 1]})
result = binning(data, "age", "label", 2)
print(result)
```
这个实现中,我们使用了 scikit-learn 库中的 `DecisionTreeClassifier` 类来进行决策树分类。我们将目标变量 `label` 作为分类标签,将特征变量 `age` 作为分类特征,通过限制最大深度 `max_depth`,控制决策树的复杂度。最后,我们将得到的阈值点插入到数据中,得到分箱后的结果。
相关推荐
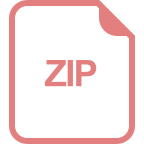
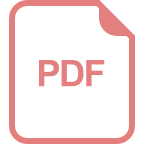



