用vue写一个购物车界面
时间: 2023-07-01 13:12:43 浏览: 53
好的,首先你需要安装Vue.js,然后创建一个Vue实例。接下来我们可以创建一个简单的购物车界面。
HTML代码:
```html
<div id="app">
<h1>购物车</h1>
<table>
<thead>
<tr>
<th>商品</th>
<th>数量</th>
<th>价格</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<tr v-for="(item, index) in cartItems" :key="index">
<td>{{ item.name }}</td>
<td>{{ item.quantity }}</td>
<td>{{ item.price }}</td>
<td><button @click="removeFromCart(index)">删除</button></td>
</tr>
</tbody>
</table>
<p>总价: {{ totalPrice }}</p>
</div>
```
在这个HTML代码中,我们使用了Vue的指令v-for来遍历购物车中的商品,v-on指令来绑定删除按钮的点击事件,并使用了插值表达式{{ }}来显示商品的名称、数量和价格。
在Vue实例中,我们可以定义购物车的数据和一些方法:
```javascript
new Vue({
el: '#app',
data: {
cartItems: [
{ name: '商品1', quantity: 1, price: 100 },
{ name: '商品2', quantity: 2, price: 200 },
{ name: '商品3', quantity: 3, price: 300 }
]
},
computed: {
totalPrice: function() {
return this.cartItems.reduce(function(total, item) {
return total + item.quantity * item.price;
}, 0);
}
},
methods: {
removeFromCart: function(index) {
this.cartItems.splice(index, 1);
}
}
});
```
在这个Vue实例中,我们定义了一个购物车数据cartItems,其中包含三个商品,每个商品有名称、数量和价格。我们还使用了计算属性totalPrice,它会根据购物车中的商品计算出总价。最后,我们定义了一个方法removeFromCart来从购物车中删除一个商品。
这样,我们就完成了一个简单的购物车界面。你可以根据需求进一步完善和优化它。
相关推荐
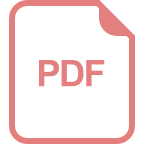
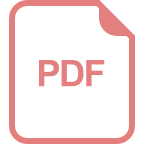
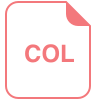
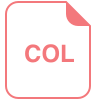
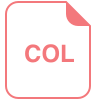
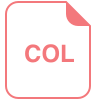
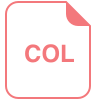









