用html vue写一个购物车界面
时间: 2024-06-09 11:04:41 浏览: 10
好的,以下是一个简单的购物车界面示例:
```html
<template>
<div>
<h1>购物车</h1>
<table>
<thead>
<tr>
<th>商品名称</th>
<th>价格</th>
<th>数量</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<tr v-for="(item, index) in shoppingCart" :key="index">
<td>{{ item.name }}</td>
<td>{{ item.price }}</td>
<td>
<button @click="decreaseQuantity(index)">-</button>
{{ item.quantity }}
<button @click="increaseQuantity(index)">+</button>
</td>
<td>
<button @click="removeItem(index)">删除</button>
</td>
</tr>
</tbody>
</table>
<p>总价:{{ totalPrice }}</p>
</div>
</template>
<script>
export default {
data() {
return {
shoppingCart: [
{ name: '商品1', price: 10, quantity: 1 },
{ name: '商品2', price: 20, quantity: 2 },
{ name: '商品3', price: 30, quantity: 1 },
]
}
},
computed: {
totalPrice() {
return this.shoppingCart.reduce((sum, item) => sum + item.price * item.quantity, 0)
}
},
methods: {
increaseQuantity(index) {
this.shoppingCart[index].quantity++
},
decreaseQuantity(index) {
if (this.shoppingCart[index].quantity > 1) {
this.shoppingCart[index].quantity--
}
},
removeItem(index) {
this.shoppingCart.splice(index, 1)
}
}
}
</script>
```
该示例使用了 Vue.js 框架,包括一个表格来显示购物车中的商品和相关信息,以及一些按钮来增加、减少或删除商品。还使用了一个计算属性来计算购物车中所有商品的总价。
相关推荐
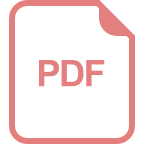
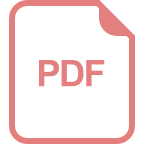
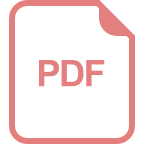














