Android怎么将文本和图片保存到Room数据库里,最后在含有recyclerview的界面中显示出来,java代码
时间: 2024-02-25 13:56:55 浏览: 121
好的,下面是Java代码实现的步骤:
首先,你需要定义一个实体类(Entity)来存储文本和图片的数据,如下所示:
```
@Entity(tableName = "note_table")
public class Note {
@PrimaryKey(autoGenerate = true)
public int id;
public String text;
public String imageUri;
}
```
其中,id是主键,text是文本数据,imageUri是图片的URI,可以为空。
接下来,你需要定义一个Dao(数据访问对象)来对数据库进行操作,如下所示:
```
@Dao
public interface NoteDao {
@Insert
void insert(Note note);
@Query("SELECT * FROM note_table")
LiveData<List<Note>> getNotes();
}
```
其中,insert方法用于插入数据,getNotes方法用于获取所有的数据。
接着,在你的ViewModel中,你需要定义一个LiveData来观察数据库中的数据变化,并且在适当的时候更新RecyclerView的数据源,如下所示:
```
public class NoteViewModel extends AndroidViewModel {
private NoteDao noteDao;
private LiveData<List<Note>> notes;
public NoteViewModel(Application application) {
super(application);
AppDatabase db = AppDatabase.getDatabase(application);
noteDao = db.noteDao();
notes = noteDao.getNotes();
}
public LiveData<List<Note>> getNotes() {
return notes;
}
public void insert(Note note) {
AppDatabase.databaseWriteExecutor.execute(() -> {
noteDao.insert(note);
});
}
}
```
最后,在含有RecyclerView的界面中,你需要创建一个Adapter来渲染数据,如下所示:
```
public class NoteAdapter extends RecyclerView.Adapter<NoteViewHolder> {
private List<Note> notes;
public NoteAdapter(List<Note> notes) {
this.notes = notes;
}
@NonNull
@Override
public NoteViewHolder onCreateViewHolder(@NonNull ViewGroup parent, int viewType) {
View view = LayoutInflater.from(parent.getContext()).inflate(R.layout.item_note, parent, false);
return new NoteViewHolder(view);
}
@Override
public void onBindViewHolder(@NonNull NoteViewHolder holder, int position) {
Note note = notes.get(position);
holder.bind(note);
}
@Override
public int getItemCount() {
return notes.size();
}
public void setNotes(List<Note> notes) {
this.notes = notes;
}
}
public class NoteViewHolder extends RecyclerView.ViewHolder {
private TextView text;
private ImageView image;
public NoteViewHolder(@NonNull View itemView) {
super(itemView);
text = itemView.findViewById(R.id.text);
image = itemView.findViewById(R.id.image);
}
public void bind(Note note) {
text.setText(note.text);
if (note.imageUri != null) {
image.setImageURI(Uri.parse(note.imageUri));
image.setVisibility(View.VISIBLE);
} else {
image.setVisibility(View.GONE);
}
}
}
```
其中,NoteAdapter用于渲染数据,NoteViewHolder用于绑定数据和视图。
最后,你可以在Activity或Fragment中使用ViewModel来观察数据变化,如下所示:
```
public class NoteActivity extends AppCompatActivity {
private NoteViewModel noteViewModel;
private NoteAdapter adapter;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_note);
RecyclerView recyclerView = findViewById(R.id.recycler_view);
recyclerView.setLayoutManager(new LinearLayoutManager(this));
adapter = new NoteAdapter(new ArrayList<>());
recyclerView.setAdapter(adapter);
noteViewModel = new ViewModelProvider(this).get(NoteViewModel.class);
noteViewModel.getNotes().observe(this, notes -> {
adapter.setNotes(notes);
adapter.notifyDataSetChanged();
});
}
}
```
这样,当你插入新的数据时,RecyclerView会自动更新显示。
阅读全文
相关推荐
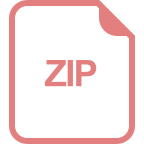

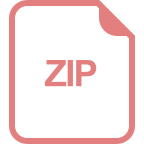
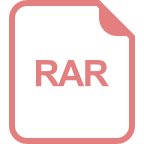
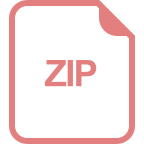
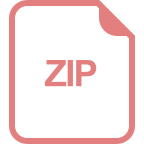
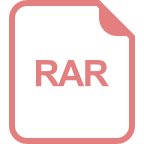
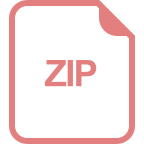
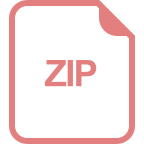
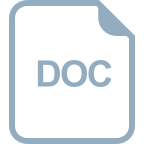
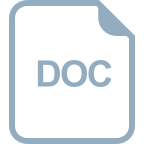
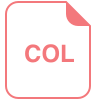


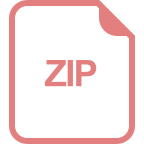