trie树词频统计根据频率排序
时间: 2023-07-06 10:05:07 浏览: 189
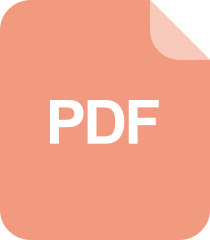
Trie树_字典树(字符串排序)简介及实现
要根据词频从高到低排序,可以使用堆(heap)数据结构来实现。具体步骤如下:
1. 构建trie树,并将每个单词的词频存储在trie树节点中。
2. 遍历整个trie树,将每个节点的单词和词频存储在一个元组中,并将元组插入到堆中。
3. 通过堆排序,将元组按照词频从高到低排序。
4. 遍历排序后的堆,输出每个节点的单词和词频即可。
以下是一个Python代码示例:
```python
import heapq
class TrieNode:
def __init__(self):
self.children = {}
self.is_word = False
self.freq = 0
class Trie:
def __init__(self):
self.root = TrieNode()
def insert(self, word):
node = self.root
for ch in word:
if ch not in node.children:
node.children[ch] = TrieNode()
node = node.children[ch]
node.is_word = True
node.freq += 1
def traverse(self):
heap = []
def dfs(node, word):
if node.is_word:
heapq.heappush(heap, (-node.freq, word))
for ch, child in node.children.items():
dfs(child, word + ch)
dfs(self.root, "")
return heap
def top_k(self, k):
heap = self.traverse()
res = []
for i in range(k):
if heap:
freq, word = heapq.heappop(heap)
res.append((word, -freq))
return res
# Example usage:
words = ["apple", "banana", "apple", "cherry", "banana", "apple"]
trie = Trie()
for word in words:
trie.insert(word)
print(trie.top_k(2)) # Output: [('apple', 3), ('banana', 2)]
```
在上面的代码中,我们首先定义了一个`TrieNode`类和一个`Trie`类,并分别用来表示trie树节点和trie树。
在`Trie`类中,`insert`方法用来向trie树中插入单词,`traverse`方法用来遍历整个trie树,并将每个节点的单词和词频存储到一个元组中,并将元组插入到堆中。最后,使用`top_k`方法来返回排序后的前k个元素。
在示例中,我们首先定义了一个包含一些单词的列表,然后创建了一个trie树,并将每个单词插入到trie树中。最后,我们调用`top_k`方法来获取词频最高的前两个单词。
阅读全文
相关推荐
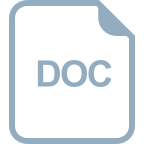
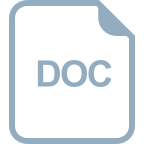


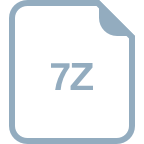
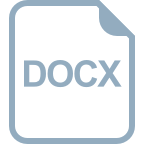
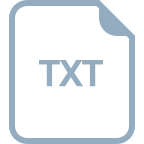
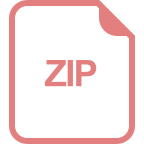
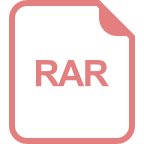
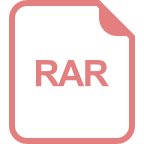
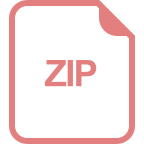
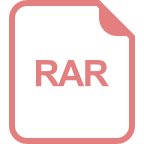
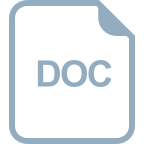
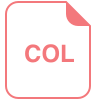
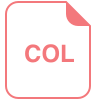
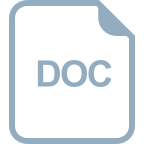
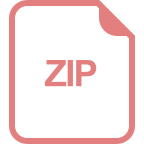