帮我用java写一个人事管理系统
时间: 2024-05-29 17:03:18 浏览: 104
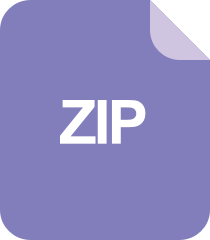
java编写的人事管理系统
好的,人事管理系统是一个比较大的项目,我可以给你提供一些基本的框架,你可以根据需要进行修改和完善。
首先,我们需要设计数据库。人事管理系统需要记录员工的个人信息、工作经历、教育背景、工资等信息。我们可以设计以下表:
- 员工表(employee):记录员工的基本信息,包括员工ID、姓名、性别、出生日期、联系电话、邮箱等。
- 工作经历表(work_experience):记录员工的工作经历,包括公司名称、职位、入职时间、离职时间等。
- 教育背景表(education_background):记录员工的教育背景,包括学校名称、学历、专业、入学时间、毕业时间等。
- 工资表(salary):记录员工的工资信息,包括基本工资、绩效奖金、岗位津贴、社保等。
接下来,我们可以使用Java语言和SQL语句来实现这些功能。以下是一个简单的示例:
```java
import java.sql.*;
public class HRSystem {
// 数据库连接信息
private String url = "jdbc:mysql://localhost:3306/hr_system";
private String username = "root";
private String password = "123456";
// 数据库连接对象
private Connection conn = null;
public HRSystem() {
try {
// 加载驱动
Class.forName("com.mysql.jdbc.Driver");
// 获取连接
conn = DriverManager.getConnection(url, username, password);
} catch (ClassNotFoundException e) {
e.printStackTrace();
} catch (SQLException e) {
e.printStackTrace();
}
}
// 添加员工信息
public boolean addEmployee(Employee employee) {
String sql = "INSERT INTO employee (id, name, gender, birthdate, phone, email) VALUES (?, ?, ?, ?, ?, ?)";
try {
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setString(1, employee.getId());
pstmt.setString(2, employee.getName());
pstmt.setString(3, employee.getGender());
pstmt.setDate(4, new java.sql.Date(employee.getBirthdate().getTime()));
pstmt.setString(5, employee.getPhone());
pstmt.setString(6, employee.getEmail());
int count = pstmt.executeUpdate();
if (count > 0) {
return true;
}
} catch (SQLException e) {
e.printStackTrace();
}
return false;
}
// 更新员工信息
public boolean updateEmployee(Employee employee) {
String sql = "UPDATE employee SET name=?, gender=?, birthdate=?, phone=?, email=? WHERE id=?";
try {
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setString(1, employee.getName());
pstmt.setString(2, employee.getGender());
pstmt.setDate(3, new java.sql.Date(employee.getBirthdate().getTime()));
pstmt.setString(4, employee.getPhone());
pstmt.setString(5, employee.getEmail());
pstmt.setString(6, employee.getId());
int count = pstmt.executeUpdate();
if (count > 0) {
return true;
}
} catch (SQLException e) {
e.printStackTrace();
}
return false;
}
// 删除员工信息
public boolean deleteEmployee(String id) {
String sql = "DELETE FROM employee WHERE id=?";
try {
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setString(1, id);
int count = pstmt.executeUpdate();
if (count > 0) {
return true;
}
} catch (SQLException e) {
e.printStackTrace();
}
return false;
}
// 查询员工信息
public Employee getEmployee(String id) {
String sql = "SELECT * FROM employee WHERE id=?";
try {
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setString(1, id);
ResultSet rs = pstmt.executeQuery();
if (rs.next()) {
Employee employee = new Employee();
employee.setId(rs.getString("id"));
employee.setName(rs.getString("name"));
employee.setGender(rs.getString("gender"));
employee.setBirthdate(rs.getDate("birthdate"));
employee.setPhone(rs.getString("phone"));
employee.setEmail(rs.getString("email"));
return employee;
}
} catch (SQLException e) {
e.printStackTrace();
}
return null;
}
// 关闭数据库连接
public void close() {
try {
conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
```
这是一个简单的人事管理系统框架,你可以根据需要进行修改和完善。同时,还需要实现其他功能,如添加、更新、删除、查询工作经历、教育背景、工资等信息。
阅读全文
相关推荐
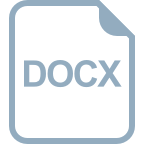
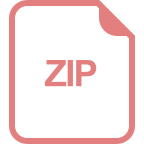
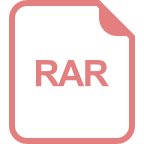
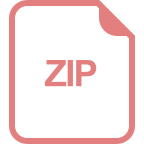
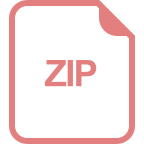
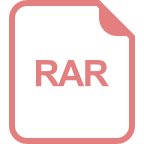
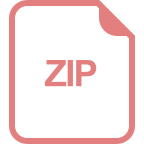
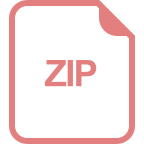