用链栈实现将非负的十进制数转换为2,8,16的进制数
时间: 2023-06-03 07:03:22 浏览: 278
好的,这个问题可以回答。以下是用链栈实现将非负的十进制数转换为2,8,16进制数的步骤:
1. 将十进制数不断除以要转换的进制数(2、8或16),直到商为0为止。
2. 将每一次除法所得的余数依次入栈。
3. 依次出栈每一个余数,得到的序列就是转换后的数。
需要注意的是,对于16进制数,需要将余数大于10的直接转换为对应的字母。(如10对应A,11对应B,以此类推)。
希望这个回答可以帮到你!
相关问题
C语言实现:【问题描述】 写一个算法,将一个非负十进制数转为一个等价的八进制数 要求使用堆栈,且堆栈的基本操作使用链栈实现 【输入形式】 输入一个非负十进制整数 【输出形式】 输出对应八进制数 【样例输入】 10 【样例输出】 12
以下是使用链栈实现十进制转八进制的C语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
int data;
struct Node *next;
} Node;
typedef struct Stack {
Node *top;
} Stack;
void initStack(Stack *s) {
s->top = NULL;
}
void push(Stack *s, int data) {
Node *node = (Node *) malloc(sizeof(Node));
node->data = data;
node->next = s->top;
s->top = node;
}
int pop(Stack *s) {
if (s->top == NULL) {
printf("Stack is empty!\n");
exit(-1);
}
Node *p = s->top;
int data = p->data;
s->top = p->next;
free(p);
return data;
}
int isEmpty(Stack *s) {
return s->top == NULL;
}
void decimalToOctal(int decimal) {
Stack s;
initStack(&s);
while (decimal > 0) {
push(&s, decimal % 8);
decimal /= 8;
}
while (!isEmpty(&s)) {
printf("%d", pop(&s));
}
}
int main() {
int decimal;
scanf("%d", &decimal);
decimalToOctal(decimal);
return 0;
}
```
首先定义了一个链栈,包含节点和栈本身两个结构体。然后实现了初始化、入栈、出栈、判断栈是否为空等基本操作。
在主函数中,先读入一个非负十进制整数,然后调用 decimalToOctal 函数进行转换。该函数将每次 decimal 取模 8 的余数入栈,直到 decimal 为 0 为止。最后弹出栈中的元素,即可得到对应的八进制数。
例如输入 10,按照算法可得到二进制数为 12,输出即为对应的八进制数。
利用链栈的基本操作,设计一个算法将一个非负十进制数转换成一个十六进制数数字串并输出。
算法步骤如下:
1. 初始化一个空的链栈;
2. 对于给定的非负十进制数,不断将其除以16,将每次的余数压入链栈中,直至商为0;
3. 从链栈底部开始依次弹出每个余数,根据十六进制规则转换成相应的数字或字母,并输出,直至链栈为空。
以下是算法的具体实现(使用C语言):
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
int data; // 存储余数
struct Node *next; // 指向下一个节点的指针
} Node;
typedef struct Stack {
Node *bottom; // 链栈底部指针
Node *top; // 链栈顶部指针
} Stack;
// 初始化链栈
void initStack(Stack *s) {
s->bottom = (Node *)malloc(sizeof(Node));
s->top = s->bottom;
s->top->next = NULL;
}
// 判断链栈是否为空
int isEmpty(Stack *s) {
return s->bottom == s->top;
}
// 入栈
void push(Stack *s, int x) {
Node *new_node = (Node *)malloc(sizeof(Node));
new_node->data = x;
new_node->next = s->top;
s->top = new_node;
}
// 出栈
int pop(Stack *s) {
if(isEmpty(s)) {
printf("Stack is empty.\n");
return -1;
}
int x = s->top->data;
Node *to_delete = s->top;
s->top = s->top->next;
free(to_delete);
return x;
}
// 十进制转十六进制
void decimalToHex(Stack *s, int decimal) {
while(decimal > 0) {
int remainder = decimal % 16;
push(s, remainder);
decimal /= 16;
}
while(!isEmpty(s)) {
int remainder = pop(s);
if(remainder >= 0 && remainder <= 9) {
printf("%d", remainder);
} else {
printf("%c", remainder - 10 + 'A');
}
}
}
int main() {
int decimal;
printf("Please input a non-negative decimal integer: ");
scanf("%d", &decimal);
Stack s;
initStack(&s);
printf("The corresponding hexadecimal number is: ");
decimalToHex(&s, decimal);
printf("\n");
return 0;
}
```
阅读全文
相关推荐

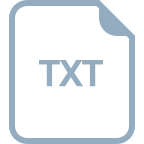
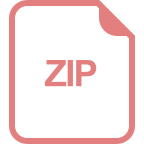
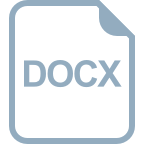
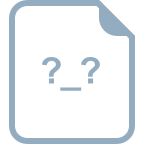
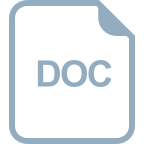
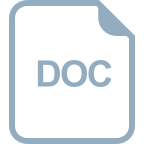
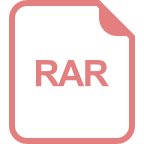
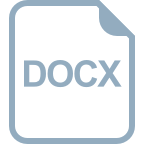