MongoDB c++ driver是同步还是异步操作,写下同步和异步增删改查接口的C++代码
时间: 2024-03-12 13:44:49 浏览: 241
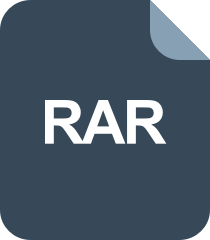
java连接Mongodb进行增删改查_java连接Mongodb进行增删改查_curiousjop_depthklb_Mong

MongoDB C++ driver支持同步和异步操作。下面分别给出同步和异步增删改查接口的C++代码示例:
同步操作:
```c++
#include <mongocxx/client.hpp>
#include <mongocxx/instance.hpp>
#include <mongocxx/options/find.hpp>
#include <mongocxx/stdx.hpp>
#include <bsoncxx/json.hpp>
mongocxx::instance instance{}; // 实例化驱动
mongocxx::client client{mongocxx::uri{}}; // 连接MongoDB数据库
mongocxx::database db = client["test"]; // 选择数据库
mongocxx::collection coll = db["test_collection"]; // 选择集合
bsoncxx::builder::stream::document document{};
document << "name" << "John Doe" << "age" << 42;
coll.insert_one(document.view()); // 插入一条文档
auto builder = bsoncxx::builder::stream::document{};
auto query = builder << "name" << "John Doe" << bsoncxx::builder::stream::finalize;
auto update = builder << "$set" << bsoncxx::builder::stream::open_document << "age" << 43 << bsoncxx::builder::stream::close_document << bsoncxx::builder::stream::finalize;
coll.update_one(query.view(), update.view()); // 更新一条文档
auto delete_result = coll.delete_one(query.view()); // 删除一条文档
```
异步操作:
```c++
#include <mongocxx/client.hpp>
#include <mongocxx/instance.hpp>
#include <mongocxx/pool.hpp>
#include <mongocxx/options/find.hpp>
#include <mongocxx/stdx.hpp>
#include <bsoncxx/json.hpp>
mongocxx::instance instance{}; // 实例化驱动
mongocxx::pool pool{mongocxx::uri{}}; // 连接池
mongocxx::database db = pool.acquire().database("test"); // 从连接池中获取连接并选择数据库
mongocxx::collection coll = db["test_collection"]; // 选择集合
auto insert_result = coll.insert_one(
bsoncxx::builder::stream::document{} << "name" << "John Doe" << "age" << 42 << bsoncxx::builder::stream::finalize,
mongocxx::options::insert{}); // 插入一条文档
auto update_result = coll.update_one(
bsoncxx::builder::stream::document{} << "name" << "John Doe" << bsoncxx::builder::stream::finalize,
bsoncxx::builder::stream::document{} << "$set" << bsoncxx::builder::stream::open_document << "age" << 43 << bsoncxx::builder::stream::close_document << bsoncxx::builder::stream::finalize,
mongocxx::options::update{}); // 更新一条文档
auto delete_result = coll.delete_one(
bsoncxx::builder::stream::document{} << "name" << "John Doe" << bsoncxx::builder::stream::finalize,
mongocxx::options::delete{}); // 删除一条文档
```
需要注意的是,异步操作需要使用连接池,而同步操作不需要。此外,异步操作需要使用回调函数处理结果,但这里没有给出回调函数的示例。
阅读全文
相关推荐
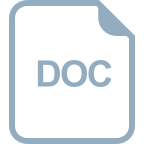
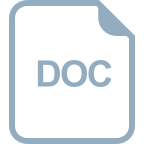
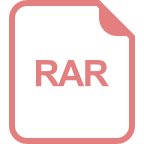
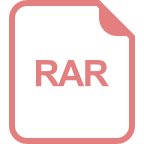
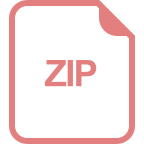
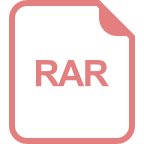
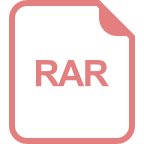
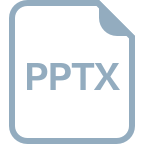
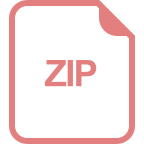
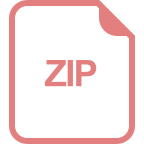
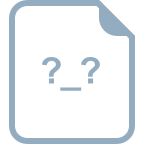
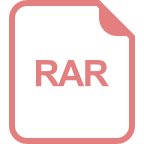
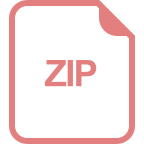
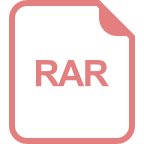
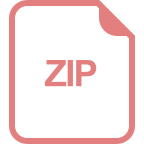
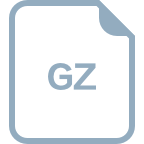
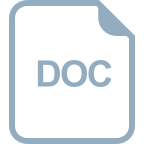
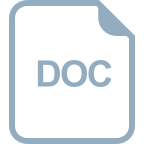