c#dictionary通过key找value
时间: 2023-04-26 08:01:34 浏览: 1792
C# 中的 Dictionary 类有一个 TryGetValue 方法,可以通过 key 来查找 value。该方法接收两个参数,一个是 key,另一个是输出参数,用来保存找到的 value。如果找到了 value,TryGetValue 方法会返回 true,反之会返回 false。
示例:
```
Dictionary<string, int> dict = new Dictionary<string, int>();
dict.Add("apple", 1);
dict.Add("banana", 2);
int value;
if (dict.TryGetValue("apple", out value))
{
Console.WriteLine(value); // 输出: 1
}
```
也可以用下标来访问,如果key不存在会抛出异常
```
Console.WriteLine(dict["apple"]);
```
相关问题
C# dictionary 通过value查找key值
在 C# 中,Dictionary 类提供了通过值查找键的方法。你可以使用 LINQ 查询语法或循环遍历字典来实现这个功能。以下是两种方法的示例:
1. 使用 LINQ 查询语法:
```csharp
var dictionary = new Dictionary<string, int>
{
{ "apple", 1 },
{ "banana", 2 },
{ "orange", 3 }
};
int targetValue = 2;
string key = dictionary.FirstOrDefault(x => x.Value == targetValue).Key;
if (key != null)
{
Console.WriteLine($"Key with value {targetValue} is: {key}");
}
else
{
Console.WriteLine("Key not found for the given value.");
}
```
2. 使用循环遍历字典:
```csharp
var dictionary = new Dictionary<string, int>
{
{ "apple", 1 },
{ "banana", 2 },
{ "orange", 3 }
};
int targetValue = 2;
string key = null;
foreach (var kvp in dictionary)
{
if (kvp.Value == targetValue)
{
key = kvp.Key;
break;
}
}
if (key != null)
{
Console.WriteLine($"Key with value {targetValue} is: {key}");
}
else
{
Console.WriteLine("Key not found for the given value.");
}
```
这些代码示例将在给定一个目标值时,通过值查找字典中对应的键。请注意,如果字典中存在多个具有相同值的键,这些方法将返回第一个匹配的键。
C# Dictionary反射用Key获取Value
可以使用反射获取Dictionary中指定Key对应的Value。以下是一个示例代码:
```csharp
using System;
using System.Collections.Generic;
using System.Reflection;
class Program
{
static void Main()
{
Dictionary<string, int> dict = new Dictionary<string, int>()
{
{ "one", 1 },
{ "two", 2 },
{ "three", 3 }
};
string key = "two";
// 使用反射获取Dictionary类的私有字段
FieldInfo field = typeof(Dictionary<string, int>).GetField("count", BindingFlags.Instance | BindingFlags.NonPublic);
int count = (int)field.GetValue(dict);
// 遍历Dictionary中的每个元素,查找指定Key对应的Value
for (int i = 0; i < count; i++)
{
KeyValuePair<string, int> pair = dict.ElementAt(i);
if (pair.Key == key)
{
int value = pair.Value;
Console.WriteLine($"The value of '{key}' is {value}");
break;
}
}
Console.ReadKey();
}
}
```
在该示例中,首先定义了一个Dictionary<string, int>类型的变量dict,并初始化了三个元素。然后,定义了一个字符串类型的变量key,用于存储要查找的Key。
接下来,使用反射获取Dictionary类的私有字段count。这是因为Dictionary类没有提供任何公共属性或方法来访问其元素数目。所以,需要使用反射获取它的私有字段。
接着,遍历Dictionary中的每个元素,查找指定Key对应的Value。如果找到了,就打印出该Value,并使用break语句退出循环。
注意,这种方法的效率并不高,因为它需要遍历整个Dictionary。如果Dictionary中的元素数量很大,那么查找所需的时间会很长。如果需要频繁地根据Key查找Value,建议使用其他数据结构,例如HashSet或SortedDictionary。
相关推荐
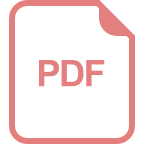
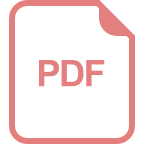
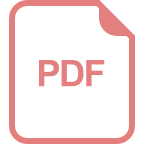












