C# Dictionary反射用Key获取Value
时间: 2024-05-06 14:16:03 浏览: 10
可以使用反射获取Dictionary中指定Key对应的Value。以下是一个示例代码:
```csharp
using System;
using System.Collections.Generic;
using System.Reflection;
class Program
{
static void Main()
{
Dictionary<string, int> dict = new Dictionary<string, int>()
{
{ "one", 1 },
{ "two", 2 },
{ "three", 3 }
};
string key = "two";
// 使用反射获取Dictionary类的私有字段
FieldInfo field = typeof(Dictionary<string, int>).GetField("count", BindingFlags.Instance | BindingFlags.NonPublic);
int count = (int)field.GetValue(dict);
// 遍历Dictionary中的每个元素,查找指定Key对应的Value
for (int i = 0; i < count; i++)
{
KeyValuePair<string, int> pair = dict.ElementAt(i);
if (pair.Key == key)
{
int value = pair.Value;
Console.WriteLine($"The value of '{key}' is {value}");
break;
}
}
Console.ReadKey();
}
}
```
在该示例中,首先定义了一个Dictionary<string, int>类型的变量dict,并初始化了三个元素。然后,定义了一个字符串类型的变量key,用于存储要查找的Key。
接下来,使用反射获取Dictionary类的私有字段count。这是因为Dictionary类没有提供任何公共属性或方法来访问其元素数目。所以,需要使用反射获取它的私有字段。
接着,遍历Dictionary中的每个元素,查找指定Key对应的Value。如果找到了,就打印出该Value,并使用break语句退出循环。
注意,这种方法的效率并不高,因为它需要遍历整个Dictionary。如果Dictionary中的元素数量很大,那么查找所需的时间会很长。如果需要频繁地根据Key查找Value,建议使用其他数据结构,例如HashSet或SortedDictionary。
相关推荐
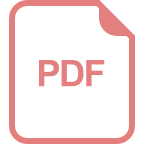
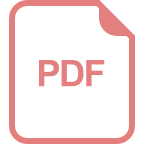
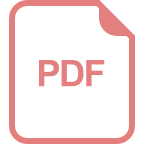














