def bubble_sort(arr)代码注释
时间: 2023-09-17 15:13:59 浏览: 94
以下是对 bubble_sort(arr) 函数的注释:
```python
def bubble_sort(arr):
"""
对传入的列表 arr 进行冒泡排序,从小到大排序。
参数:
arr:待排序的列表
返回值:
无返回值,原列表被排序。
"""
n = len(arr)
# 外层循环控制比较轮数,共需要比较 n-1 轮
for i in range(n-1):
# 内层循环控制每轮比较的位置,由于每轮确定一个最大值,
# 所以只需要比较前 n-i-1 个元素即可
for j in range(n-i-1):
# 如果前一个元素比后一个元素大,交换两个元素
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
```
相关问题
def bubble_sort
Bubble sort is a simple sorting algorithm that repeatedly steps through the list, compares adjacent elements and swaps them if they are in the wrong order. The pass through the list is repeated until the list is sorted. Here's an example of bubble sort in Python:
```
def bubble_sort(arr):
n = len(arr)
# Traverse through all array elements
for i in range(n):
# Last i elements are already in place
for j in range(0, n-i-1):
# Swap if the element found is greater than the next element
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
return arr
```
This function takes an array `arr` as input and returns a sorted array using bubble sort. The outer loop runs `n` times, where `n` is the length of the array. For each iteration of the outer loop, the inner loop runs `n-i-1` times, where `i` is the current iteration of the outer loop. The inner loop compares adjacent elements and swaps them if they are in the wrong order. At the end of each iteration of the outer loop, the last `i` elements are already in place, so the inner loop doesn't need to check them again.
Bubble_sort函数
Bubble_sort函数是一种简单的排序算法,它通过多次遍历列表,比较相邻元素的大小并交换位置,将最大(或最小)的元素逐渐“冒泡”到列表的末尾。这个过程会不断重复,直到整个列表排序完成。
以下是一个使用Bubble_sort函数进行排序的示例代码:
```python
def bubble_sort(arr):
n = len(arr)
for i in range(n-1):
for j in range(0, n-i-1):
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
# 示例用法
arr = [64, 34, 25, 12, 22, 11, 90]
bubble_sort(arr)
print("排序后的列表:", arr)
```
运行以上代码,输出结果为:
```
排序后的列表: [11, 12, 22, 25, 34, 64, 90]
```
阅读全文
相关推荐
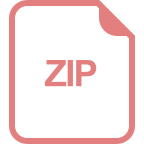
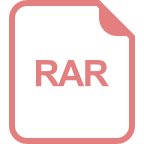
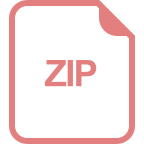













