python bubble sort
时间: 2023-04-27 07:02:23 浏览: 588
Python 冒泡排序
冒泡排序是一种简单的排序算法,它重复地遍历要排序的列表,比较每对相邻的项,并在必要时交换它们的位置。通过多次遍历列表,将最大的项逐渐“浮”到列表的顶部,因此称为冒泡排序。
以下是 Python 中实现冒泡排序的示例代码:
```python
def bubble_sort(arr):
n = len(arr)
# 遍历所有数组元素
for i in range(n):
# 最后 i 个元素已经排好序
for j in range(0, n-i-1):
# 如果当前元素大于下一个元素,则交换它们的位置
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
# 测试代码
arr = [64, 34, 25, 12, 22, 11, 90]
bubble_sort(arr)
print("排序后的数组:")
for i in range(len(arr)):
print("%d" %arr[i])
```
输出结果:
```
排序后的数组:
11
12
22
25
34
64
90
```
在上面的代码中,我们使用两个嵌套的循环来遍历列表中的所有元素。外部循环用于遍历列表中的所有元素,而内部循环用于比较相邻的元素并交换它们的位置。在每次内部循环结束时,最大的元素都会“浮”到列表的顶部。重复这个过程,直到整个列表都被排序。
相关问题
python Bubble Sort
Bubble sort is a simple sorting algorithm that works by repeatedly swapping adjacent elements if they are in the wrong order. The algorithm gets its name from the way smaller elements "bubble" to the top of the list during each pass.
Here is an example implementation of bubble sort in Python:
```
def bubble_sort(arr):
n = len(arr)
# Traverse through all array elements
for i in range(n):
# Last i elements are already in place
for j in range(0, n-i-1):
# Swap if the element found is greater than the next element
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
return arr
```
To use this function, simply pass in an array of integers:
```
arr = [64, 34, 25, 12, 22, 11, 90]
sorted_arr = bubble_sort(arr)
print(sorted_arr) # Output: [11, 12, 22, 25, 34, 64, 90]
```
In this example, we pass in an unsorted array of integers and use the `bubble_sort` function to sort them in ascending order. The sorted array is then printed to the console.
bubble sort python
Sure! Here's an example of bubble sort implemented in Python:
```python
def bubble_sort(arr):
n = len(arr)
for i in range(n):
# Last i elements are already in place
for j in range(0, n-i-1):
# Traverse the array from 0 to n-i-1
# Swap if the element found is greater than the next element
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
return arr
# Example usage
arr = [64, 34, 25, 12, 22, 11, 90]
sorted_arr = bubble_sort(arr)
print(sorted_arr)
```
This code will sort the given array using the bubble sort algorithm. The outer loop runs `n` times, where `n` is the length of the array. In each iteration of the outer loop, the inner loop compares adjacent elements and swaps them if they are in the wrong order. This process is repeated until the entire array is sorted. The sorted array is then returned and printed.
阅读全文
相关推荐
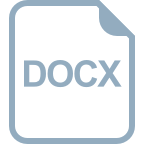
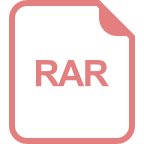



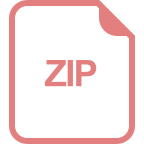
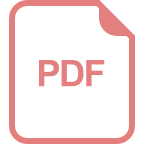
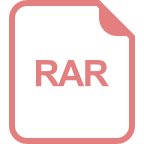






