bubble sort python
时间: 2023-10-19 14:34:17 浏览: 207
Sure! Here's an example of bubble sort implemented in Python:
```python
def bubble_sort(arr):
n = len(arr)
for i in range(n):
# Last i elements are already in place
for j in range(0, n-i-1):
# Traverse the array from 0 to n-i-1
# Swap if the element found is greater than the next element
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
return arr
# Example usage
arr = [64, 34, 25, 12, 22, 11, 90]
sorted_arr = bubble_sort(arr)
print(sorted_arr)
```
This code will sort the given array using the bubble sort algorithm. The outer loop runs `n` times, where `n` is the length of the array. In each iteration of the outer loop, the inner loop compares adjacent elements and swaps them if they are in the wrong order. This process is repeated until the entire array is sorted. The sorted array is then returned and printed.
阅读全文
相关推荐
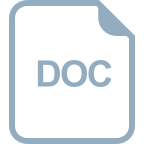
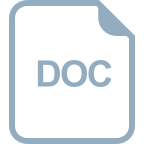
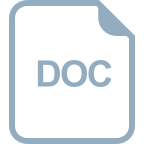
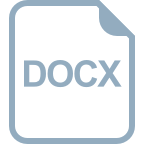
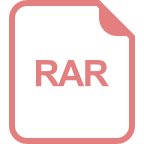
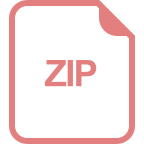





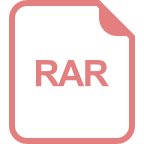
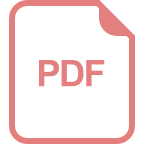
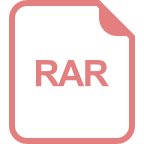
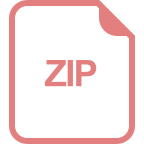



