python Bubble Sort
时间: 2024-05-10 13:21:00 浏览: 138
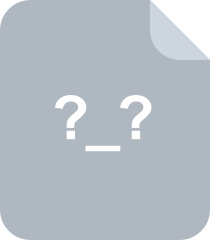
bubble sort
Bubble sort is a simple sorting algorithm that works by repeatedly swapping adjacent elements if they are in the wrong order. The algorithm gets its name from the way smaller elements "bubble" to the top of the list during each pass.
Here is an example implementation of bubble sort in Python:
```
def bubble_sort(arr):
n = len(arr)
# Traverse through all array elements
for i in range(n):
# Last i elements are already in place
for j in range(0, n-i-1):
# Swap if the element found is greater than the next element
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
return arr
```
To use this function, simply pass in an array of integers:
```
arr = [64, 34, 25, 12, 22, 11, 90]
sorted_arr = bubble_sort(arr)
print(sorted_arr) # Output: [11, 12, 22, 25, 34, 64, 90]
```
In this example, we pass in an unsorted array of integers and use the `bubble_sort` function to sort them in ascending order. The sorted array is then printed to the console.
阅读全文
相关推荐
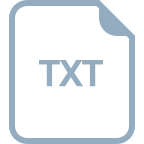

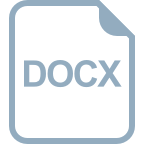




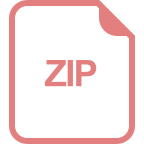
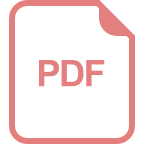
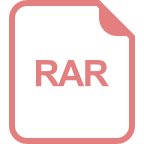






