pyqt5,对tableview中的某几列数据进行筛选,要求能选出满足条件的行,要求能对多列设置筛选条件,要求能进行数学筛选,利用QSortFilterProxyModel,提供条件设置窗口及开始按钮,提供代码示例
时间: 2024-05-13 21:19:47 浏览: 6
以下是一个简单的PyQt5应用程序,用于对表格视图中的多个列进行筛选。它使用QSortFilterProxyModel类来实现筛选,并提供了一个条件设置窗口和一个开始按钮。
在这个示例中,我们假设有一个包含学生姓名,年龄和成绩的表格视图。用户可以选择要筛选的列,设置一个或多个条件,并点击开始按钮以执行筛选。筛选后,只有满足所有条件的行会被显示。
```python
from PyQt5.QtWidgets import QApplication, QMainWindow, QTableView, QDialog, QVBoxLayout, QHBoxLayout, QLabel, QLineEdit, QComboBox, QPushButton
from PyQt5.QtCore import Qt, QSortFilterProxyModel, QModelIndex
from PyQt5.QtGui import QStandardItemModel, QStandardItem
class FilterDialog(QDialog):
def __init__(self, parent=None):
super().__init__(parent)
self.setWindowTitle("设置筛选条件")
self.setFixedSize(300, 200)
layout = QVBoxLayout()
column_label = QLabel("选择列:")
self.column_combo = QComboBox()
self.column_combo.addItems(["姓名", "年龄", "成绩"])
layout.addWidget(column_label)
layout.addWidget(self.column_combo)
condition_label = QLabel("设置条件:")
self.condition_edit = QLineEdit()
layout.addWidget(condition_label)
layout.addWidget(self.condition_edit)
button_layout = QHBoxLayout()
self.ok_button = QPushButton("确定")
self.cancel_button = QPushButton("取消")
button_layout.addWidget(self.ok_button)
button_layout.addWidget(self.cancel_button)
layout.addLayout(button_layout)
self.setLayout(layout)
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle("表格筛选器")
self.setGeometry(100, 100, 800, 600)
self.model = QStandardItemModel()
self.model.setHorizontalHeaderLabels(["姓名", "年龄", "成绩"])
self.proxy_model = QSortFilterProxyModel()
self.proxy_model.setSourceModel(self.model)
self.table_view = QTableView()
self.table_view.setModel(self.proxy_model)
self.setCentralWidget(self.table_view)
self.filter_dialog = FilterDialog(self)
self.filter_button = QPushButton("开始筛选", self)
self.filter_button.clicked.connect(self.show_filter_dialog)
self.statusBar().addWidget(self.filter_button)
def show_filter_dialog(self):
result = self.filter_dialog.exec_()
if result == QDialog.Accepted:
column_index = self.filter_dialog.column_combo.currentIndex()
condition = self.filter_dialog.condition_edit.text()
self.add_filter(column_index, condition)
def add_filter(self, column_index, condition):
self.proxy_model.setFilterKeyColumn(column_index)
self.proxy_model.setFilterRegExp(condition)
if __name__ == '__main__':
app = QApplication([])
window = MainWindow()
window.show()
app.exec_()
```
这个示例程序中,我们创建了一个MainWindow类来管理主窗口和表格视图。我们还创建了一个FilterDialog类来提供一个条件设置窗口。在主窗口中,我们创建了一个QSortFilterProxyModel对象来处理筛选逻辑,并将其设置为表格视图的模型。我们还创建了一个按钮来触发条件设置窗口的显示,并在用户点击“确定”按钮时添加筛选条件。
在add_filter方法中,我们使用setFilterKeyColumn方法来设置要筛选的列,使用setFilterRegExp方法来设置筛选条件。您可以根据需要更改这些方法来实现您自己的筛选逻辑。注意,setFilterRegExp方法使用正则表达式来匹配筛选条件。
希望这个示例能帮助您开始使用PyQt5进行表格视图筛选。
相关推荐
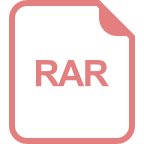
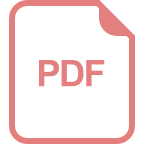
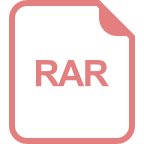














