举个实际的程序例子满足以下条件The CAN interface is used to control motor operation and to set and modify motor configuration parameters. The motor control interface should at a minimum provide a C++ application programming interface (API), with appropriate header files. Motor configuration parameters are preferably set and modified through a command line interface, accessible from a Linux terminal shell, although including motor configuration commands with the motor control API may be acceptable.
时间: 2023-06-12 13:07:05 浏览: 58
以下是一个示例程序,它提供了一个使用CAN接口控制电机操作并设置和修改电机配置参数的C API,并通过命令行界面访问电机配置命令。该程序可以运行在Linux操作系统上。
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
#define CAN_INTERFACE "can0"
// Define motor control API here
void start_motor();
void stop_motor();
void set_motor_speed(int speed);
// Define motor configuration API here
void set_motor_config(char* config_name, char* config_value);
char* get_motor_config(char* config_name);
int main(int argc, char** argv) {
// Initialize CAN interface here
// ...
// Parse command line arguments here
if(argc < 2) {
printf("Usage: %s [command] [arguments]\n", argv[0]);
exit(1);
}
// Handle motor control commands here
if(strcmp(argv[1], "start") == 0) {
start_motor();
}
else if(strcmp(argv[1], "stop") == 0) {
stop_motor();
}
else if(strcmp(argv[1], "speed") == 0) {
if(argc < 3) {
printf("Usage: %s speed [speed value]\n", argv[0]);
exit(1);
}
int speed = atoi(argv[2]);
set_motor_speed(speed);
}
// Handle motor configuration commands here
else if(strcmp(argv[1], "set") == 0) {
if(argc < 4) {
printf("Usage: %s set [config name] [config value]\n", argv[0]);
exit(1);
}
set_motor_config(argv[2], argv[3]);
}
else if(strcmp(argv[1], "get") == 0) {
if(argc < 3) {
printf("Usage: %s get [config name]\n", argv[0]);
exit(1);
}
char* config_value = get_motor_config(argv[2]);
printf("%s\n", config_value);
free(config_value);
}
// Display usage information if command is not recognized
else {
printf("Unrecognized command: %s\n", argv[1]);
exit(1);
}
return 0;
}
void start_motor() {
// Implement motor start command here
// ...
}
void stop_motor() {
// Implement motor stop command here
// ...
}
void set_motor_speed(int speed) {
// Implement motor speed command here
// ...
}
void set_motor_config(char* config_name, char* config_value) {
// Implement motor configuration set command here
// ...
}
char* get_motor_config(char* config_name) {
// Implement motor configuration get command here
char* config_value = (char*)malloc(256*sizeof(char));
// ...
return config_value;
}
```
在此示例程序中,我们定义了一个基本的C API,用于控制电机操作和设置/修改电机配置参数。我们还定义了一个命令行界面,该界面通过解析命令行参数来调用相应的API函数。此外,我们还可以在适当的位置添加CAN接口初始化和其他必要的代码来实现完整的电机控制系统。
相关推荐
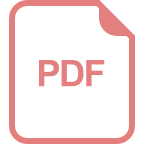
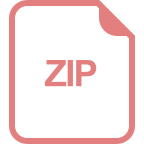














