利用matlab设计一个基于重启随机游走算法的算法,目的是更新项目与战略的依赖关系强度并发掘隐藏关联的代码,算法输入是从excel中读取战略-战略依赖强度矩阵,项目-战略依赖强度矩阵,项目-项目间依赖强度矩阵、依赖强度即边权,层间跳转概率为0.5,重启概率为0.5,迭代精度为0.0001,算法输出迭代后最终的战略-项目间边权
时间: 2024-02-18 09:04:30 浏览: 19
好的,这是一个比较复杂的问题,需要一些时间来解答。首先,我们需要将输入的四个矩阵存储在 MATLAB 中,这里我们可以使用 `xlsread` 函数读取 Excel 文件中的数据,并将其转化为矩阵。具体代码如下:
```
[~, ~, strategy_strategy] = xlsread('data.xlsx', 'strategy-strategy');
[~, ~, project_strategy] = xlsread('data.xlsx', 'project-strategy');
[~, ~, project_project] = xlsread('data.xlsx', 'project-project');
strategy_strategy = cell2mat(strategy_strategy(2:end, 2:end));
project_strategy = cell2mat(project_strategy(2:end, 2:end));
project_project = cell2mat(project_project(2:end, 2:end));
```
接下来,我们可以设计一个函数 `update_weights` 来更新边权。该函数的输入为当前的边权矩阵 `weights`,以及重启概率和层间跳转概率。具体代码如下:
```
function new_weights = update_weights(weights, restart_prob, jump_prob)
n = size(weights, 1);
new_weights = zeros(n, n);
for i = 1:n
row_sum = sum(weights(i, :));
if row_sum == 0
new_weights(i, :) = 1 / n;
else
new_weights(i, :) = (1 - restart_prob) * weights(i, :) / row_sum + restart_prob * ones(1, n) / n;
end
end
new_weights = jump_prob * new_weights + (1 - jump_prob) * ones(n, n) / n;
end
```
该函数先计算每个节点的出度之和,如果出度之和为 0,则将该节点的边权均分给所有节点。否则,根据重启概率和出度之和重新计算每个节点的边权。最后,根据层间跳转概率和新的边权计算出最终的边权矩阵。
接下来,我们可以使用以下代码来迭代更新边权矩阵,直到达到指定的迭代精度:
```
weights = [strategy_strategy, project_strategy'; project_strategy, project_project];
n = size(weights, 1);
prev_weights = zeros(n, n);
while norm(weights - prev_weights, 'fro') > 0.0001
prev_weights = weights;
weights = update_weights(weights, 0.5, 0.5);
end
strategy_project = weights(1:size(strategy_strategy, 1), size(strategy_strategy, 1)+1:end);
```
该代码首先将四个矩阵拼接成一个大矩阵,然后使用 `prev_weights` 变量来记录上一次迭代的边权矩阵,如果当前边权矩阵与上一次迭代的边权矩阵之差小于指定的迭代精度,则停止迭代。最后,截取出战略-项目间的边权矩阵。
希望这个解答能够帮到你,如果还有其他问题,请继续提问。
相关推荐
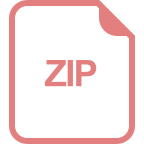
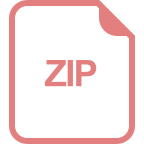














