C++执行python外部程序
时间: 2023-10-19 09:22:08 浏览: 90
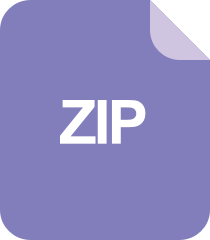
C++调用Python
在C++中执行Python外部程序可以使用系统调用函数`system()`或者使用进程管理函数`fork()`和`exec()`的组合。
使用`system()`函数可以通过以下方式执行Python程序:
```cpp
#include <cstdlib>
std::string command = "python path/to/your/script.py";
system(command.c_str());
```
这里将Python脚本的路径和名称存储在一个字符串变量中,然后将其作为参数传递给`system()`函数。
使用`fork()`和`exec()`的组合可以实现更高级的进程控制。以下是一个示例代码:
```cpp
#include <unistd.h>
#include <sys/wait.h>
#include <iostream>
int main() {
pid_t pid = fork();
if (pid == -1) {
std::cerr << "Failed to fork process" << std::endl;
exit(1);
} else if (pid == 0) {
// Child process
execlp("python", "python", "path/to/your/script.py", nullptr);
exit(0);
} else {
// Parent process
int status;
waitpid(pid, &status, 0);
if (WIFEXITED(status) && (WEXITSTATUS(status) == 0)) {
std::cout << "Python script executed successfully" << std::endl;
} else {
std::cerr << "Failed to execute Python script" << std::endl;
}
}
return 0;
}
```
在这个示例中,使用`fork()`函数创建一个新的进程,然后调用`execlp()`函数执行Python程序。`execlp()`函数的第一个参数是程序的名称,第二个参数是程序的路径和名称,后面的参数是程序的命令行参数。`waitpid()`函数等待子进程结束,并检查进程的退出状态。如果进程成功退出,则输出一条成功消息。否则,输出一条错误消息。
阅读全文
相关推荐

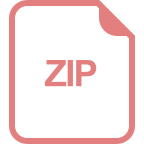
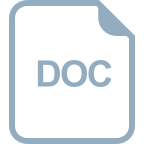
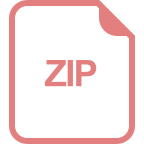
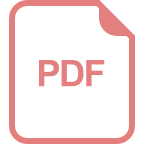
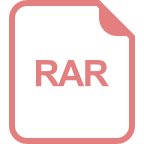
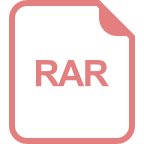
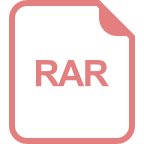
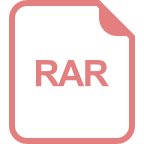
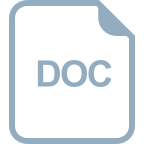
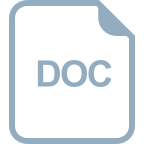
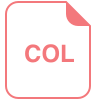
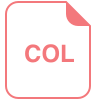


