C语言如何在使用回调函数的情况下,在按下键盘上的上下左右是,实现屏幕上的小人发生左右移动(注意,此时小人在迷宫内)
时间: 2024-02-16 17:59:43 浏览: 97
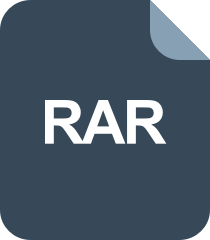
回调函数实现控制上下左右
首先,你需要使用一个回调函数来处理键盘输入。你可以使用 `conio.h` 库中的 `getch()` 函数来获取按键的 ASCII 值。然后,你可以将键盘输入映射到小人在屏幕上的移动。
以下是一个示例代码,实现了在按下上下左右键时小人在屏幕上移动的功能。你需要自己实现小人在迷宫内移动的逻辑。
```c
#include <stdio.h>
#include <conio.h>
#include <windows.h>
// 定义小人的位置
int x = 10;
int y = 10;
// 定义屏幕大小
int screen_width = 80;
int screen_height = 25;
// 定义小人的移动速度
int speed = 1;
// 回调函数,处理键盘输入
void handle_input() {
if (kbhit()) { // 如果有键盘输入
char c = getch(); // 获取按键的 ASCII 值
switch (c) { // 根据按键的值处理不同的移动操作
case 'w': // 上移
if (y > 0) {
y -= speed;
}
break;
case 's': // 下移
if (y < screen_height - 1) {
y += speed;
}
break;
case 'a': // 左移
if (x > 0) {
x -= speed;
}
break;
case 'd': // 右移
if (x < screen_width - 1) {
x += speed;
}
break;
default:
break;
}
}
}
// 主函数,实现绘制小人和屏幕更新
int main() {
while (1) { // 无限循环,直到退出程序
// 绘制小人
system("cls"); // 清空屏幕
for (int i = 0; i < y; i++) {
printf("\n");
}
for (int i = 0; i < x; i++) {
printf(" ");
}
printf("O\n");
for (int i = 0; i < x; i++) {
printf(" ");
}
printf("| |\n");
for (int i = 0; i < x; i++) {
printf(" ");
}
printf("/ \\\n");
// 处理键盘输入
handle_input();
// 等待一段时间,控制小人移动的速度
Sleep(50);
}
return 0;
}
```
在这个示例代码中,我们使用了 `system("cls")` 函数清空屏幕,并使用 `printf` 函数在屏幕上绘制小人。 `kbhit` 函数判断是否有键盘输入,`getch` 函数获取按键的 ASCII 值。根据按键的值,我们更新小人的位置。`Sleep` 函数控制小人移动的速度。你需要根据自己的需求进行调整。
阅读全文
相关推荐
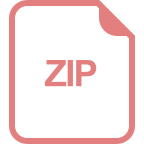
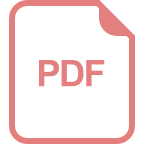
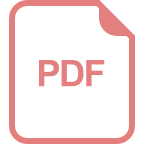
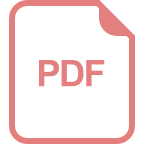
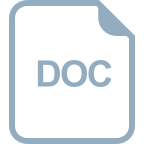
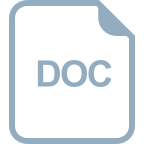
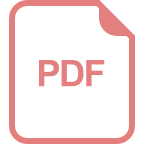
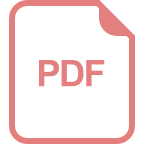
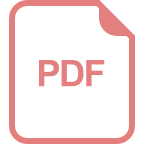
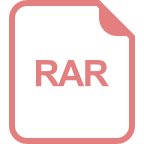
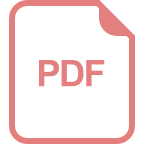
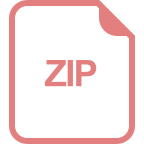