query(valuation.code,valuation.circulating_market_cap).filter(valuation.code.in_(test_list)).order_by(valuation.circulating_market_cap.asc())得到什么数据
时间: 2024-02-17 22:01:41 浏览: 115
这段代码可以得到一个 SQLAlchemy 查询对象,该对象包含了符合条件的数据。
具体来说,该查询会返回 `valuation` 表中 `code` 值在 `test_list` 中的行,并按照 `circulating_market_cap` 列的值升序排序。查询对象中包含了 `code` 和 `circulating_market_cap` 两个列的值。如果需要获取查询结果,可以使用查询对象的 `all()` 方法或者迭代器进行遍历。
以下是一个示例代码,展示如何使用查询对象获取数据:
```python
result = query(valuation.code, valuation.circulating_market_cap).filter(valuation.code.in_(test_list)).order_by(valuation.circulating_market_cap.asc()).all()
# 遍历结果
for row in result:
code, market_cap = row
print(f"Code: {code}, Market Cap: {market_cap}")
```
在这个示例中,我们使用了查询对象的 `all()` 方法获取了查询结果,并使用一个 for 循环对结果进行遍历。在每次循环中,我们从结果中获取了 `code` 和 `circulating_market_cap` 两个列的值,并将其打印输出。
相关问题
这是我的一个表://定义:每只指数属于行业A,是因为这只指数的第一大成分行业是A。 //每个行业指数列表生成的SQL代码。(以石油石化为例) SELECT t_fund_index_valuation.`index_code`, t_fund_index_valuation.`index_name`, t_fund_index_valuation.`truly_valuation`, t_fund_index_valuation.`market_value`, t_fund_index_valuation.`stock_industry_name_1`, t_fund_index_valuation.`stock_industry_weight_1`, t_fund_index_valuation.`stock_industry_name_2`, t_fund_index_valuation.`stock_industry_weight_2`, t_fund_index_valuation.`stock_industry_name_3`, t_fund_index_valuation.`stock_industry_weight_3`, CONCAT( t_fund_index_valuation.`stock_industry_name_1`, ' ', t_fund_index_valuation.`stock_industry_weight_1`, ' %+', t_fund_index_valuation.`stock_industry_name_2`, ' ', t_fund_index_valuation.`stock_industry_weight_2`, ' %+', t_fund_index_valuation.`stock_industry_name_3`, ' ', t_fund_index_valuation.`stock_industry_weight_3`, ' %' ) AS combine FROM t_fund_index_valuation 如果我只希望展示stock_industry_name_1等于“食品饮料”的行,怎么写
如果您只希望展示 `stock_industry_name_1` 等于 "食品饮料" 的行,您可以在查询中添加一个 `WHERE` 子句来过滤结果。以下是相应的查询代码:
```sql
SELECT
t_fund_index_valuation.`index_code`,
t_fund_index_valuation.`index_name`,
t_fund_index_valuation.`truly_valuation`,
t_fund_index_valuation.`market_value`,
t_fund_index_valuation.`stock_industry_name_1`,
t_fund_index_valuation.`stock_industry_weight_1`,
t_fund_index_valuation.`stock_industry_name_2`,
t_fund_index_valuation.`stock_industry_weight_2`,
t_fund_index_valuation.`stock_industry_name_3`,
t_fund_index_valuation.`stock_industry_weight_3`,
CONCAT(
t_fund_index_valuation.`stock_industry_name_1`, ' ',
t_fund_index_valuation.`stock_industry_weight_1`, ' %+',
t_fund_index_valuation.`stock_industry_name_2`, ' ',
t_fund_index_valuation.`stock_industry_weight_2`, ' %+',
t_fund_index_valuation.`stock_industry_name_3`, ' ',
t_fund_index_valuation.`stock_industry_weight_3`, ' %'
) AS combine
FROM t_fund_index_valuation
WHERE t_fund_index_valuation.`stock_industry_name_1` = '食品饮料'
```
在上述查询中,我们添加了 `WHERE` 子句,并将条件设置为 `t_fund_index_valuation.stock_industry_name_1 = '食品饮料'`,这样只有 `stock_industry_name_1` 等于 "食品饮料" 的行才会被查询出来。请注意,我假设您使用的是 MySQL 数据库,如果是其他数据库系统,请根据具体的数据库语法进行相应的修改。
修改这串代码import jqdatasdk as jq from datetime import datetime # 设置聚宽账户信息 jq.auth('username', 'password') jq.set_token('API token') # 设置选股条件 price_limit = 20 # 股票价格上限 pe_limit = 50 # 市盈率上限 pb_limit = 5 # 市净率上限 # 获取当前时间 now = datetime.now().strftime('%H:%M:%S') # 判断是否处于集合竞价时段 if now >= '09:20:00' and now <= '09:25:00': # 获取股票池 stocks = jq.get_all_securities(types=['stock']).index.tolist() # 筛选符合条件的股票 q = jq.query(jq.valuation.code, jq.valuation.pe_ratio, jq.valuation.pb_ratio).filter( jq.valuation.pe_ratio < pe_limit, jq.valuation.pb_ratio < pb_limit) df = jq.get_fundamentals(q, date=None) stocks = df['code'].tolist() # 过滤股票价格 prices = jq.get_price(stocks, end_date=datetime.now(), frequency='minute', fields=['close']) stocks = prices[prices['close'] <= price_limit].index.tolist() # 获取持仓信息 positions = jq.get_position('stock') # 调整持仓 for stock in positions: if stock in stocks: # 如果当前持仓的股票仍符合条件,则继续持有 pass else: # 如果当前持仓的股票不符合条件,则卖出 jq.order(stock, amount=0, side='sell') # 集合竞价买入 for stock in stocks: jq.order(stock, amount=100, side='buy', style='limit', price=price_limit)
这段代码看起来已经很完整了,不知道您需要修改哪些部分。如果您需要修改选股条件或者买卖数量,可以修改以下代码:
```python
# 设置选股条件
price_limit = 20 # 股票价格上限
pe_limit = 50 # 市盈率上限
pb_limit = 5 # 市净率上限
# 集合竞价买入
for stock in stocks:
jq.order(stock, amount=100, side='buy', style='limit', price=price_limit)
```
其中,price_limit、pe_limit 和 pb_limit 分别对应股票价格、市盈率和市净率的上限值,可以根据自己的需求进行修改。amount 参数用于指定买卖股票的数量,可以根据自己的资金状况和风险偏好进行调整。
另外,如果您需要增加其他操作或者条件判断,也可以在代码中进行修改。
阅读全文
相关推荐
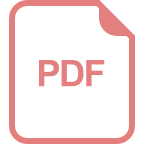
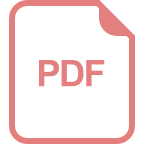
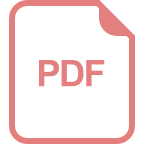














