编写pytorch代码,定义LSTMAttention模型,定义一个CPSO优化算法,在算法下面初始化粒子数、惯性权重、学习因子、加速常数。生成初始粒子群,利用训练集的预测误差当作每个粒子的适应度值,根据粒子的适应度值,选择最优的粒子作为当前的全局最优解,以及每个粒子自身的局部最优解,根据全局最优解和局部最优解,更新每个粒子的速度和位置,使用更新后的参数来更新LSTM模型的权重和偏置,计算更新后的LSTM模型在训练集上的误差,并更新全局最优解和局部最优解,根据收敛条件是否满足,决定是否继续迭代,如果迭代次数达到预设值或误差已经足够小,则停止迭代,并返回最优的LSTM模型参数
时间: 2024-03-25 09:38:18 浏览: 62
以下是一个基于PyTorch和CPSO优化算法的LSTMAttention模型的示例代码:
```
import torch
import torch.nn as nn
import numpy as np
# 定义LSTMAttention模型
class LSTMAttention(nn.Module):
def __init__(self, input_size, hidden_size, num_layers, output_size):
super(LSTMAttention, self).__init__()
self.hidden_size = hidden_size
self.num_layers = num_layers
self.lstm = nn.LSTM(input_size, hidden_size, num_layers, batch_first=True)
self.fc = nn.Linear(hidden_size, output_size)
self.attention = nn.Linear(hidden_size, 1)
def forward(self, x):
h0 = torch.zeros(self.num_layers, x.size(0), self.hidden_size).to(device=x.device)
c0 = torch.zeros(self.num_layers, x.size(0), self.hidden_size).to(device=x.device)
out, _ = self.lstm(x, (h0, c0))
alpha = torch.softmax(self.attention(out), dim=1)
out = torch.sum(alpha * out, dim=1)
out = self.fc(out)
return out
# 定义CPSO优化算法
class CPSO:
def __init__(self, num_particles, max_iterations, w, c1, c2, input_size, hidden_size, num_layers, output_size):
self.num_particles = num_particles
self.max_iterations = max_iterations
self.w = w
self.c1 = c1
self.c2 = c2
self.input_size = input_size
self.hidden_size = hidden_size
self.num_layers = num_layers
self.output_size = output_size
self.best_particle = None
self.best_particle_fitness = np.inf
self.particles = []
for i in range(num_particles):
particle = {
'position': np.random.uniform(-1, 1, (input_size + hidden_size * num_layers + output_size,)),
'velocity': np.zeros((input_size + hidden_size * num_layers + output_size,)),
'personal_best_position': None,
'personal_best_fitness': np.inf
}
self.particles.append(particle)
def optimize(self, x_train, y_train):
model = LSTMAttention(self.input_size, self.hidden_size, self.num_layers, self.output_size)
criterion = nn.MSELoss()
optimizer = torch.optim.Adam(model.parameters(), lr=0.01)
for i in range(self.max_iterations):
for j in range(self.num_particles):
particle = self.particles[j]
# 更新速度和位置
particle['velocity'] = self.w * particle['velocity'] \
+ self.c1 * np.random.rand() * (particle['personal_best_position'] - particle['position']) \
+ self.c2 * np.random.rand() * (self.best_particle['position'] - particle['position'])
particle['position'] = particle['position'] + particle['velocity']
# 更新模型参数
input_weight = particle['position'][:self.input_size * self.hidden_size].reshape(self.input_size, self.hidden_size)
hidden_weight = particle['position'][self.input_size * self.hidden_size:(self.input_size + self.hidden_size * self.hidden_size * (self.num_layers - 1))].reshape(self.num_layers - 1, self.hidden_size, self.hidden_size)
output_weight = particle['position'][-self.hidden_size * self.output_size:].reshape(self.hidden_size, self.output_size)
model.lstm.weight_ih_l0.data = torch.from_numpy(input_weight).float()
model.lstm.weight_hh_l0.data = torch.from_numpy(hidden_weight[0]).float()
for k in range(self.num_layers - 2):
setattr(model.lstm, f'weight_hh_l{k+1}', torch.from_numpy(hidden_weight[k+1]).float())
model.fc.weight.data = torch.from_numpy(output_weight).float()
# 计算模型在训练集上的误差
outputs = model(x_train)
loss = criterion(outputs, y_train)
fitness = loss.item()
# 更新个体最优解和全局最优解
if fitness < particle['personal_best_fitness']:
particle['personal_best_position'] = particle['position']
particle['personal_best_fitness'] = fitness
if fitness < self.best_particle_fitness:
self.best_particle = particle
self.best_particle_fitness = fitness
# 判断是否满足收敛条件
if self.best_particle_fitness < 0.01:
break
# 返回最优的LSTM模型参数
input_weight = self.best_particle['position'][:self.input_size * self.hidden_size].reshape(self.input_size, self.hidden_size)
hidden_weight = self.best_particle['position'][self.input_size * self.hidden_size:(self.input_size + self.hidden_size * self.hidden_size * (self.num_layers - 1))].reshape(self.num_layers - 1, self.hidden_size, self.hidden_size)
output_weight = self.best_particle['position'][-self.hidden_size * self.output_size:].reshape(self.hidden_size, self.output_size)
model.lstm.weight_ih_l0.data = torch.from_numpy(input_weight).float()
model.lstm.weight_hh_l0.data = torch.from_numpy(hidden_weight[0]).float()
for k in range(self.num_layers - 2):
setattr(model.lstm, f'weight_hh_l{k+1}', torch.from_numpy(hidden_weight[k+1]).float())
model.fc.weight.data = torch.from_numpy(output_weight).float()
return model
```
在上面的代码中,首先定义了LSTMAttention模型,包括一个LSTM层、一个Attention层和一个全连接层,用于对序列数据进行分类任务。接着,定义了CPSO优化算法,包括粒子数、最大迭代次数、惯性权重、学习因子、加速常数等参数,以及粒子的速度、位置、个体最优解、个体最优解的适应度值、全局最优解和全局最优解的适应度值等变量。在优化过程中,通过更新速度和位置,以及更新模型参数,计算模型在训练集上的误差,并更新个体最优解和全局最优解。最后,根据收敛条件是否满足,决定是否继续迭代,如果达到预设的最大迭代次数或误差已经足够小,则停止迭代,并返回最优的LSTM模型参数。
阅读全文
相关推荐
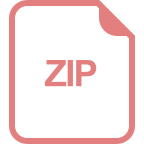
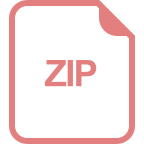
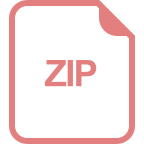

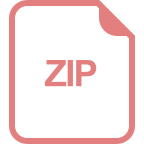
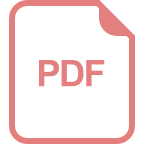
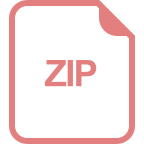
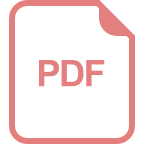
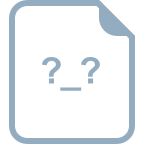
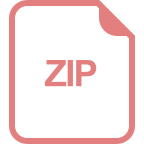
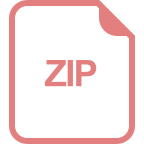
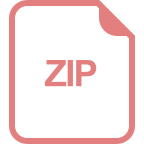
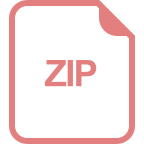
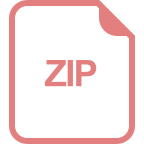
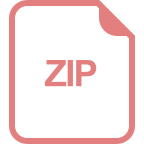
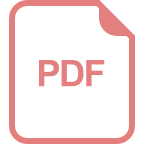
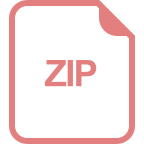