编写pytorch代码,定义FA_CPSO优化算法,在这个算法下面初始化粒子群的位置、速度、种群规模等参数,通过混沌粒子群算法迭代更新粒子群的参数,在参数达到最优时加入萤火虫随机扰动,进一步优化参数,直到得到粒子群最优参数
时间: 2023-07-24 13:50:50 浏览: 99
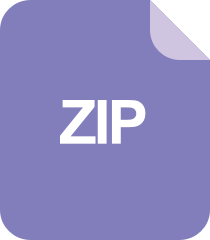
eval_coco.zip

好的,让我来帮您解答这个问题。
首先,需要安装PyTorch和numpy库:
```python
!pip install torch
!pip install numpy
```
然后,定义FA_CPSO优化算法的类:
```python
import torch
import numpy as np
class FA_CPSO:
def __init__(self, f, dim, swarm_size, max_iter, w_min, w_max, c1, c2):
self.f = f
self.dim = dim
self.swarm_size = swarm_size
self.max_iter = max_iter
self.w_min = w_min
self.w_max = w_max
self.c1 = c1
self.c2 = c2
def optimize(self):
# 初始化粒子群的位置和速度
swarm_pos = torch.rand(self.swarm_size, self.dim)
swarm_vel = torch.rand(self.swarm_size, self.dim)
swarm_best_pos = swarm_pos.clone()
swarm_best_score = torch.zeros(self.swarm_size)
for i in range(self.swarm_size):
swarm_best_score[i] = self.f(swarm_best_pos[i])
global_best_pos = swarm_best_pos[torch.argmin(swarm_best_score)]
global_best_score = torch.min(swarm_best_score)
# 迭代更新粒子群的参数
for t in range(self.max_iter):
w = self.w_max - (self.w_max - self.w_min) * t / self.max_iter
c = self.c1 + self.c2
rand = torch.rand(self.swarm_size, self.dim)
swarm_vel = w * swarm_vel + self.c1 * rand * (swarm_best_pos - swarm_pos) + self.c2 * rand * (global_best_pos - swarm_pos)
swarm_pos = swarm_pos + swarm_vel
# 使用混沌粒子群算法更新位置
for i in range(self.swarm_size):
r1 = np.random.random()
r2 = np.random.random()
swarm_pos[i] = r1 * swarm_pos[i] + r2 * global_best_pos
# 更新最优位置和得分
for i in range(self.swarm_size):
score = self.f(swarm_pos[i])
if score < swarm_best_score[i]:
swarm_best_pos[i] = swarm_pos[i]
swarm_best_score[i] = score
if score < global_best_score:
global_best_pos = swarm_pos[i]
global_best_score = score
# 萤火虫随机扰动
for i in range(self.swarm_size):
if torch.rand(1) < 0.1:
swarm_pos[i] = swarm_pos[i] + 0.1 * torch.randn(self.dim)
return global_best_pos, global_best_score
```
其中,`f`是目标函数,`dim`是参数维度,`swarm_size`是粒子群规模,`max_iter`是最大迭代次数,`w_min`和`w_max`是惯性权重的最小值和最大值,`c1`和`c2`是加速系数。`optimize`方法返回全局最优位置和得分。
最后,可以使用以下代码对函数进行测试:
```python
# 定义目标函数
def sphere(x):
return torch.sum(x ** 2)
# 初始化FA_CPSO算法并运行
fa_cpso = FA_CPSO(sphere, 10, 20, 100, 0.4, 0.9, 2.0, 2.0)
best_pos, best_score = fa_cpso.optimize()
print("最优位置:", best_pos)
print("最优得分:", best_score)
```
这里以10维的sphere函数为例,运行20个粒子,最大迭代次数为100次。输出最优位置和得分。
阅读全文
相关推荐
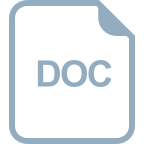
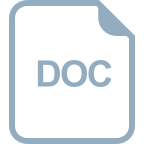
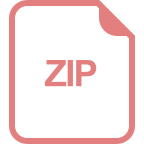
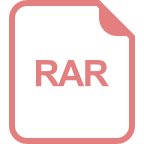
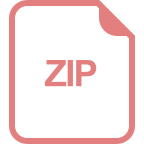
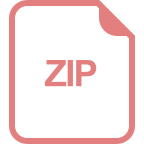
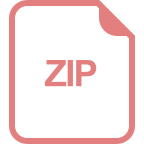
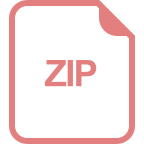
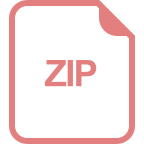
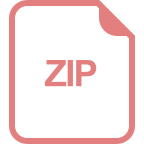
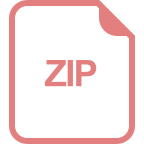
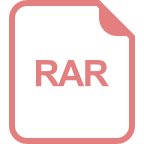
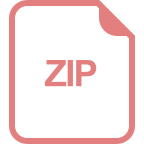
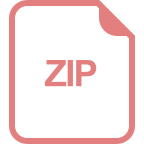
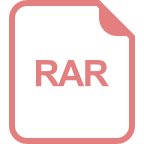
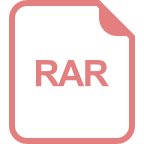
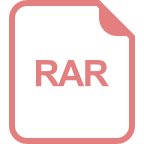