俄罗斯方块代码java
时间: 2023-08-30 22:11:01 浏览: 40
以下是一个简单的俄罗斯方块游戏的Java代码示例:
```java
import java.awt.Color;
import java.awt.Dimension;
import java.awt.Graphics;
import java.awt.Point;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.KeyEvent;
import java.awt.event.KeyListener;
import java.util.ArrayList;
import java.util.Random;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.Timer;
public class Tetris extends JPanel implements ActionListener, KeyListener {
private static final long serialVersionUID = 1L;
private static final int WIDTH = 300;
private static final int HEIGHT = 600;
private static final int BLOCK_SIZE = 30;
private Timer timer;
private ArrayList<Point> blocks;
private Point currentBlock;
private int[][] board;
private boolean isGameOver;
private boolean isPaused;
private Random random;
private int score;
public Tetris() {
setPreferredSize(new Dimension(WIDTH, HEIGHT));
setBackground(Color.BLACK);
setFocusable(true);
addKeyListener(this);
timer = new Timer(500, this);
blocks = new ArrayList<Point>();
board = new int[HEIGHT/BLOCK_SIZE][WIDTH/BLOCK_SIZE];
random = new Random();
startGame();
}
public void startGame() {
isGameOver = false;
isPaused = false;
score = 0;
blocks.clear();
board = new int[HEIGHT/BLOCK_SIZE][WIDTH/BLOCK_SIZE];
newBlock();
timer.start();
}
public void newBlock() {
int blockType = random.nextInt(7);
switch (blockType) {
case 0:
currentBlock = new Point(3, 0);
blocks.add(currentBlock);
blocks.add(new Point(4, 0));
blocks.add(new Point(5, 0));
blocks.add(new Point(5, 1));
break;
case 1:
currentBlock = new Point(4, 0);
blocks.add(currentBlock);
blocks.add(new Point(3, 1));
blocks.add(new Point(4, 1));
blocks.add(new Point(5, 1));
break;
case 2:
currentBlock = new Point(4, 0);
blocks.add(currentBlock);
blocks.add(new Point(5, 0));
blocks.add(new Point(3, 1));
blocks.add(new Point(4, 1));
break;
case 3:
currentBlock = new Point(4, 0);
blocks.add(currentBlock);
blocks.add(new Point(3, 0));
blocks.add(new Point(5, 1));
blocks.add(new Point(4, 1));
break;
case 4:
currentBlock = new Point(4, 0);
blocks.add(currentBlock);
blocks.add(new Point(3, 1));
blocks.add(new Point(4, 1));
blocks.add(new Point(5, 1));
break;
case 5:
currentBlock = new Point(4, 0);
blocks.add(currentBlock);
blocks.add(new Point(3, 0));
blocks.add(new Point(4, 1));
blocks.add(new Point(5, 1));
break;
case 6:
currentBlock = new Point(4, 0);
blocks.add(currentBlock);
blocks.add(new Point(3, 1));
blocks.add(new Point(4, 1));
blocks.add(new Point(4, 2));
break;
}
}
public void paintComponent(Graphics g) {
super.paintComponent(g);
for (Point block : blocks) {
drawBlock(g, block.x * BLOCK_SIZE, block.y * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE, Color.WHITE);
}
for (int y = 0; y < board.length; y++) {
for (int x = 0; x < board[y].length; x++) {
if (board[y][x] == 1) {
drawBlock(g, x * BLOCK_SIZE, y * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE, Color.WHITE);
}
}
}
if (isGameOver) {
g.setColor(Color.RED);
g.drawString("GAME OVER", WIDTH/2 - 40, HEIGHT/2);
}
if (isPaused) {
g.setColor(Color.YELLOW);
g.drawString("PAUSED", WIDTH/2 - 30, HEIGHT/2);
}
g.setColor(Color.WHITE);
g.drawString("Score: " + score, 10, 20);
}
public void drawBlock(Graphics g, int x, int y, int width, int height, Color color) {
g.setColor(color);
g.fillRect(x, y, width, height);
g.setColor(Color.BLACK);
g.drawRect(x, y, width, height);
}
public void actionPerformed(ActionEvent e) {
if (!isPaused) {
if (isGameOver) {
timer.stop();
} else {
moveDown();
}
}
repaint();
}
public void moveDown() {
if (canMove(currentBlock.x, currentBlock.y + 1)) {
currentBlock.y++;
} else {
addToBoard();
checkLines();
newBlock();
if (!canMove(currentBlock.x, currentBlock.y)) {
isGameOver = true;
}
}
}
public void addToBoard() {
for (Point block : blocks) {
board[block.y][block.x] = 1;
}
}
public void checkLines() {
int lines = 0;
for (int y = board.length - 1; y >= 0; y--) {
boolean isLine = true;
for (int x = 0; x < board[y].length; x++) {
if (board[y][x] == 0) {
isLine = false;
break;
}
}
if (isLine) {
lines++;
for (int i = y; i > 0; i--) {
System.arraycopy(board[i - 1], 0, board[i], 0, board[i].length);
}
for (int i = 0; i < board[0].length; i++) {
board[0][i] = 0;
}
}
}
score += lines * 100;
}
public boolean canMove(int x, int y) {
for (Point block : blocks) {
int newX = block.x + x;
int newY = block.y + y;
if (newX < 0 || newX >= WIDTH/BLOCK_SIZE || newY >= HEIGHT/BLOCK_SIZE || board[newY][newX] == 1) {
return false;
}
}
return true;
}
public void keyPressed(KeyEvent e) {
if (!isPaused && !isGameOver) {
switch (e.getKeyCode()) {
case KeyEvent.VK_LEFT:
if (canMove(currentBlock.x - 1, currentBlock.y)) {
currentBlock.x--;
}
break;
case KeyEvent.VK_RIGHT:
if (canMove(currentBlock.x + 1, currentBlock.y)) {
currentBlock.x++;
}
break;
case KeyEvent.VK_DOWN:
if (canMove(currentBlock.x, currentBlock.y + 1)) {
currentBlock.y++;
}
break;
case KeyEvent.VK_UP:
rotate();
break;
case KeyEvent.VK_P:
isPaused = true;
break;
}
} else if (isPaused) {
if (e.getKeyCode() == KeyEvent.VK_P) {
isPaused = false;
}
} else {
if (e.getKeyCode() == KeyEvent.VK_ENTER) {
startGame();
}
}
repaint();
}
public void rotate() {
if (currentBlock != null) {
ArrayList<Point> newBlocks = new ArrayList<Point>();
for (Point block : blocks) {
int newX = block.y - currentBlock.y + currentBlock.x;
int newY = currentBlock.x - block.x + currentBlock.y;
newBlocks.add(new Point(newX, newY));
}
if (canRotate(newBlocks)) {
blocks.clear();
blocks.addAll(newBlocks);
}
}
}
public boolean canRotate(ArrayList<Point> newBlocks) {
for (Point block : newBlocks) {
if (block.x < 0 || block.x >= WIDTH/BLOCK_SIZE || block.y >= HEIGHT/BLOCK_SIZE || board[block.y][block.x] == 1) {
return false;
}
}
return true;
}
public void keyReleased(KeyEvent e) {}
public void keyTyped(KeyEvent e) {}
public static void main(String[] args) {
JFrame frame = new JFrame("Tetris");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setResizable(false);
Tetris tetris = new Tetris();
frame.add(tetris);
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
}
}
```
该代码使用Java Swing库创建了一个简单的俄罗斯方块游戏。它实现了基本的游戏逻辑和动画,并支持左右移动、旋转、加速下落、暂停和重新开始等功能。
相关推荐
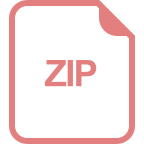
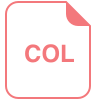
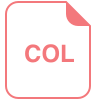
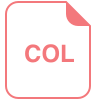
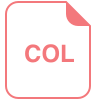
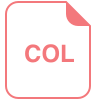








