vue3中如何确保在数据加载完毕后再访问该属性
时间: 2024-02-25 12:59:19 浏览: 44
在Vue3中,可以使用`watchEffect`函数来监视响应式数据的变化,并在变化时执行副作用函数。可以在副作用函数中访问该属性,确保数据已经加载完毕。
例如,假设我们有一个异步加载数据的函数`fetchData()`,并且需要访问其返回的数据中的某个属性`foo`,可以使用以下代码确保在数据加载完毕后再访问该属性:
```javascript
import { reactive, watchEffect } from 'vue'
const state = reactive({
data: null,
foo: null
})
watchEffect(async () => {
state.data = await fetchData()
state.foo = state.data.foo
})
```
在上面的代码中,`watchEffect`函数会监视`state`对象的变化,并在变化时执行副作用函数。副作用函数中会调用`fetchData`函数异步加载数据,并将其赋值给`state.data`。然后,`state.foo`会被赋值为`state.data.foo`,确保在数据加载完毕后再访问该属性。
相关问题
vue mounted
### 回答1:
`mounted` 是 Vue 组件生命周期钩子函数之一,它表示组件已经被挂载到页面上并且可以访问到 DOM 元素。在 `mounted` 钩子函数中,你可以执行一些需要访问 DOM 元素的操作,比如使用 jQuery 或者其他 JavaScript 库来操作 DOM。同时,你也可以在这个钩子函数中发起异步请求获取数据并更新组件的状态。
示例代码:
```javascript
export default {
mounted() {
// 使用 jQuery 操作 DOM
$('.my-element').addClass('active');
// 发起异步请求获取数据
axios.get('/api/data')
.then(response => {
this.data = response.data;
})
.catch(error => {
console.log(error);
});
}
}
```
### 回答2:
mounted是Vue的生命周期钩子函数之一,它在Vue实例被创建之后立即被调用。
在mounted钩子函数中,Vue实例已经完成了编译过程,将模板渲染成了最终的HTML,并将其挂载到了DOM上。这说明在mounted钩子函数执行的时候,Vue实例已经可以访问到挂载的DOM元素。
在mounted钩子函数中,我们可以进行一些需要访问DOM的操作,例如操作DOM元素、绑定事件监听等。因为只有在mounted之后,才能确保已经存在需要操作的DOM元素。
在mounted钩子函数中,也可以调用其他Vue实例的方法或访问其属性。这是因为在Vue实例创建之后,各个实例之间已经建立了联系,可以进行相互调用和访问。
总之,mounted钩子函数是Vue实例完成挂载的一个重要时刻,它提供了一个访问DOM和其他Vue实例的时机,可以用于执行一些需要在DOM完全渲染之后才能进行的操作。
### 回答3:
Vue的mounted钩子函数是Vue生命周期中的一个阶段,它在Vue实例被挂载到DOM之后立即调用。
在mounted函数中,Vue实例已经完成了初始化过程,它的el属性已经指向了Vue实例所挂载的元素,可以通过this.$el来访问这个元素。
一般情况下,我们可以在mounted函数中进行一些需要操作DOM的初始化工作,比如绑定事件、初始化插件、发送网络请求等。
当Vue实例被挂载到DOM之后,mounted函数会被触发,这意味着此时DOM已经完全渲染完毕,可以进行各种操作,而不会因为DOM元素不存在而导致错误。
举个例子,假设我们要在页面加载完成后显示一个弹窗,我们可以在mounted函数中调用一个弹窗插件的初始化方法,并传入相关配置项即可。
另外,mounted函数还可以用来监听DOM事件,比如点击事件、滚动事件等。我们可以在mounted函数中通过addEventListener来添加事件监听器,然后在相应的回调函数中处理事件。
需要注意的是,mounted函数只会在Vue实例的初始渲染过程中被调用一次,而不会在每次数据更新时都被调用。如果想在数据更新后执行一些操作,可以使用updated钩子函数。
总结起来,Vue的mounted函数是用来在Vue实例被挂载到DOM之后进行一些初始化工作的钩子函数,它在Vue生命周期中的位置是在created之后,beforeMounted之前。
vue Cannot read properties of undefined (reading 'replace')
在 Vue 中出现 "Cannot read properties of undefined (reading 'replace')" 错误通常表示您尝试在一个未定义的变量上使用 `replace` 方法。这可能是因为您的数据未正确初始化或未正确传递到 Vue 组件中。
以下是一些可能导致此错误的常见情况和解决方法:
1. 数据未正确初始化:确保在使用 `replace` 方法之前,将相关数据正确初始化。例如,如果您尝试在一个未定义的变量上使用 `replace` 方法,可以在 Vue 组件的 `data` 属性中初始化该变量。
```javascript
data() {
return {
myVariable: ''
}
},
```
2. 异步数据加载:如果您的数据是通过异步请求获取的,可能会导致在数据加载之前访问该数据而出现错误。在使用异步数据之前,可以使用 `v-if` 指令或条件渲染来确保数据已经加载完毕。
```html
<template>
<div v-if="myData">
{{ myData.replace('old', 'new') }}
</div>
</template>
```
3. 父子组件通信问题:如果您的数据是从父组件传递给子组件的,确保在子组件中正确接收和处理该数据。可以使用 `props` 属性来传递数据,并使用 `v-if` 指令或条件渲染来确保父组件已经传递了正确的数据。
```html
<!-- 父组件 -->
<template>
<child-component :my-prop="myData"></child-component>
</template>
<!-- 子组件 -->
<template>
<div v-if="myProp">
{{ myProp.replace('old', 'new') }}
</div>
</template>
<script>
export default {
props: {
myProp: {
type: String,
default: ''
}
}
}
</script>
```
请根据您的具体情况检查并修复问题。如果问题仍然存在,可以提供更多的代码和上下文信息,以便我们能够更好地帮助您解决问题。
相关推荐
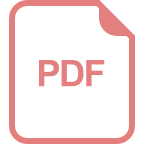
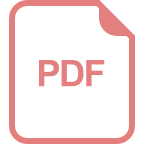











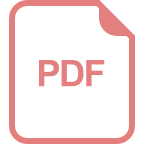