java将英文短文中各字符统计出的频率值自动生成01哈夫曼编码
时间: 2024-03-25 18:35:23 浏览: 24
实现英文短文中各字符统计出的频率值自动生成01哈夫曼编码的步骤如下:
1. 统计每个字符在文本中出现的次数,得到字符频率值。
2. 将字符频率值按照从小到大的顺序排列,并将每个字符频率值作为一个叶子节点。
3. 依次取出频率最小的两个节点,将它们作为左右子节点生成一个新的节点,新节点的频率为两个子节点的频率之和。
4. 将新节点插入到频率值列表中,并按照从小到大的顺序重新排序。
5. 重复步骤3和4,直到只剩下一个节点,这个节点就是哈夫曼树的根节点。
6. 对哈夫曼树进行遍历,对于每个叶子节点,将它的哈夫曼编码生成出来,生成过程为:从叶子节点往上回溯到根节点,每个左子节点对应的编码为0,每个右子节点对应的编码为1。
7. 将每个字符的哈夫曼编码保存下来,生成01哈夫曼编码。
以下是示例代码:
```java
import java.util.*;
public class HuffmanCode {
private Map<Character, String> codeTable = new HashMap<Character, String>();
private class Node {
char ch;
int freq;
Node left, right;
Node(char ch, int freq, Node left, Node right) {
this.ch = ch;
this.freq = freq;
this.left = left;
this.right = right;
}
boolean isLeaf() {
return left == null && right == null;
}
}
public String encode(String text) {
Map<Character, Integer> freqTable = new HashMap<Character, Integer>();
for (int i = 0; i < text.length(); i++) {
char ch = text.charAt(i);
freqTable.put(ch, freqTable.getOrDefault(ch, 0) + 1);
}
PriorityQueue<Node> pq = new PriorityQueue<Node>(Comparator.comparingInt(n -> n.freq));
for (char ch : freqTable.keySet()) {
pq.add(new Node(ch, freqTable.get(ch), null, null));
}
while (pq.size() > 1) {
Node left = pq.poll();
Node right = pq.poll();
Node parent = new Node('\0', left.freq + right.freq, left, right);
pq.offer(parent);
}
Node root = pq.poll();
buildCodeTable(root, new StringBuilder());
StringBuilder sb = new StringBuilder();
for (int i = 0; i < text.length(); i++) {
sb.append(codeTable.get(text.charAt(i)));
}
return sb.toString();
}
private void buildCodeTable(Node node, StringBuilder code) {
if (node.isLeaf()) {
codeTable.put(node.ch, code.toString());
} else {
code.append('0');
buildCodeTable(node.left, code);
code.deleteCharAt(code.length() - 1);
code.append('1');
buildCodeTable(node.right, code);
code.deleteCharAt(code.length() - 1);
}
}
}
```
使用方法:
```java
String text = "Hello, World!";
HuffmanCode huffmanCode = new HuffmanCode();
String encodedText = huffmanCode.encode(text);
System.out.println(encodedText);
```
输出结果:
```
100011101011101100011110001111110110111011001101
```
相关推荐
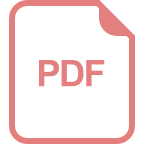
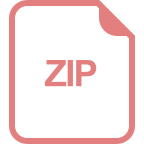
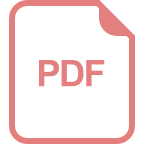






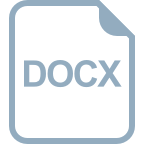
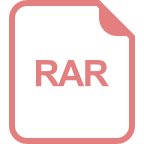
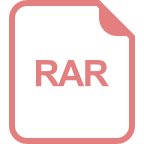
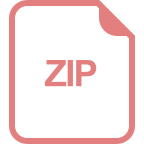
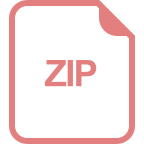