c++中怎么把字符串转换为整数
时间: 2023-05-12 14:06:45 浏览: 94
可以使用 atoi 函数将字符串转换为整数。例如:
```c
#include <stdio.h>
#include <stdlib.h>
int main() {
char str[] = "123";
int num = atoi(str);
printf("%d\n", num);
return 0;
}
```
输出结果为:
```
123
```
相关问题
c++将字符串转换成整数
在C++中,可以使用`std::stoi`函数将字符串转换成整数。该函数接受一个字符串参数,并返回对应的整数值。例如:
```cpp
#include <iostream>
#include <string>
int main() {
std::string str = "123";
int num = std::stoi(str);
std::cout << num << std::endl; // 输出 123
return 0;
}
```
需要注意的是,如果字符串中包含非数字字符,或者超出了整数类型的取值范围,`std::stoi`函数会抛出`std::invalid_argument`或`std::out_of_range`异常。因此,在使用该函数时,需要加入异常处理机制。
c++中怎么把整数转换成字符串
在C++中,可以使用标准库函数`std::to_string()`将整数转换为字符串。
具体用法如下:
```cpp
#include <string>
int num = 123;
std::string str = std::to_string(num);
```
这样就可以将整数`num`转换为字符串`str`。需要注意的是,`to_string()`函数只能转换基本类型的数字,例如`int`、`float`等等,对于自定义类型的数字需要定义`to_string()`函数。
另外,如果你使用的是 C++11 或以上的版本,也可以使用`std::to_chars()`函数将整数转换为字符串。用法如下:
```cpp
#include <charconv>
int num = 123;
char str[10];
std::to_chars(str, str + 10, num);
```
这样就可以将整数`num`转换为字符数组`str`。需要注意的是,`to_chars()`函数需要传入一个字符数组的指针和长度,且长度至少应该为转换后的字符串长度加一(用于存储字符串结束符`\0`)。
相关推荐
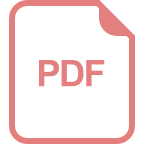
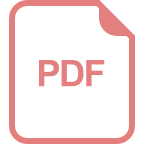












