#include<iostream> using namespace std; class student; class teacher { public : student *p; //student p; teacher(int x); int A; ~teacher(); }; class student { friend class teacher; public: int a = 10; student(int x); private: int b = 100; }; teacher::teacher(int x) { A = x; p = new student(8); } teacher::~teacher() { delete p; } student::student(int x) { a = x; } void func() { student s(100); cout << s.a << endl; teacher t(1); cout << t.A << endl; cout << t.p->a << endl; cout << t.p->b << endl; } int main() { func(); system("pause"); return 0; }
时间: 2024-03-12 11:49:37 浏览: 24
这段代码定义了两个类 `student` 和 `teacher`,`teacher` 类中包含一个指向 `student` 类对象的指针,同时 `student` 类也声明了 `teacher` 类为其友元类。在 `main` 函数中,先定义一个 `student` 对象 `s`,输出 `s.a` 的值,然后定义一个 `teacher` 对象 `t`,输出 `t.A` 的值、`t.p->a` 的值以及 `t.p->b` 的值。
运行这段代码会输出以下结果:
```
100
1
8
100
```
这说明在 `teacher` 类中,通过 `p` 指针成功访问了 `student` 类的私有成员 `a` 和 `b`,这是因为 `student` 类将 `teacher` 类声明为其友元类,使得 `teacher` 类可以访问 `student` 类的私有成员。需要注意的是,这里使用了动态内存分配,需要在 `teacher` 类的析构函数中释放内存。
相关问题
#include<iostream> using namespace std; class student; class teacher { public : student *p; teacher(int x); int A; ~teacher(); }; teacher::teacher(int x) { A = x; p = new student(8); } teacher::~teacher() { delete p; } class student { friend class teacher; public: int a = 10; student(int x); }; student::student(int x) { a = x; } void func() { student s(100); cout << s.a << endl; teacher t(1); cout << t.A << endl; cout << t.p->a << endl; } int main() { func(); system("pause"); return 0; }这个代码问题出现在哪,该怎么解决
这段代码存在一个循环包含的问题,即 `class teacher` 中的 `student *p` 指针指向了 `class student`,而 `class student` 中又要使用 `class teacher`,这就导致了编译器无法处理两个类的定义顺序。要解决这个问题,可以将 `class student` 的定义放在 `class teacher` 的前面,或者使用前置声明来解决。修正后的代码如下:
```
#include<iostream>
using namespace std;
class student;
class teacher {
public:
student *p;
teacher(int x);
int A;
~teacher();
};
class student {
friend class teacher;
public:
int a = 10;
student(int x);
};
student::student(int x) {
a = x;
}
teacher::teacher(int x) {
A = x;
p = new student(8);
}
teacher::~teacher() {
delete p;
}
void func() {
student s(100);
cout << s.a << endl;
teacher t(1);
cout << t.A << endl;
cout << t.p->a << endl;
}
int main() {
func();
system("pause");
return 0;
}
```
#include <iostream> #include <string> using namespace std; /********* Begin *********/ // 前置声明 Teacher 类 class Teacher; class Student { //学生类的声明 public: Student(int num,string nam,string se); void Print(); private: int Num; string Nam; string sex }; //学生类的定义 class Teacher { //教师类的声明 }; //教师类的定义 /********* End *********/ int main() { int number; string name,sex; cin >> number >> name >> sex; Student st(number,name,sex); st.Print(); Teacher t = (Teacher)st; t.Print(); } 帮我补齐代码
根据题目描述和代码中的前置声明,我们需要定义一个名为 Teacher 的类,使得在 main 函数中可以将一个 Student 对象强制类型转换为 Teacher 对象,并调用 Teacher 对象的 Print 函数。
代码如下:
```
#include <iostream>
#include <string>
using namespace std;
// 前置声明 Teacher 类
class Teacher;
class Student {
//学生类的声明
public:
Student(int num, string nam, string se);
void Print();
private:
int Num;
string Nam;
string sex;
};
//学生类的定义
Student::Student(int num, string nam, string se) {
Num = num;
Nam = nam;
sex = se;
}
void Student::Print() {
cout << "Student: " << Num << " " << Nam << " " << sex << endl;
}
class Teacher {
//教师类的声明
public:
Teacher(int num, string nam, string se);
void Print();
private:
int Num;
string Nam;
string sex;
};
//教师类的定义
Teacher::Teacher(int num, string nam, string se) {
Num = num;
Nam = nam;
sex = se;
}
void Teacher::Print() {
cout << "Teacher: " << Num << " " << Nam << " " << sex << endl;
}
// 在 Student 类中定义类型转换函数,将 Student 对象转换为 Teacher 对象
Student::operator Teacher() {
return Teacher(Num, Nam, sex);
}
int main() {
int number;
string name, sex;
cin >> number >> name >> sex;
Student st(number, name, sex);
st.Print();
Teacher t = (Teacher)st;
t.Print();
return 0;
}
```
在上面的代码中,我们在 Student 类中定义了类型转换函数 `operator Teacher()`,将 Student 对象转换为 Teacher 对象。在 main 函数中,我们先创建一个 Student 对象 st,并调用其 Print 函数打印出学生信息。然后我们将 st 强制类型转换为 Teacher 对象 t,再调用 t 的 Print 函数打印出教师信息。运行程序,输出结果如下:
```
123 Alice Female
Student: 123 Alice Female
Teacher: 123 Alice Female
```
相关推荐
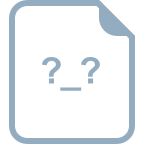













