修改并完善此代码#include<iostream> using namespace std; #include<cstring> class Teacher { public: Teacher() { } Teacher(char *p,int a) { pname=new char[20]; strcpy(pname,p); age=a; } Teacher(Teacher &t):pname(t.pname),age(t.age) { pname=new char[20]; //strcpy(pname,t.pname); } ~Teacher() { delete []pname; } Teacher& operator =(Teacher &t) { delete []pname; pname=new char[strlen(t.pname)+1]; strcpy(pname,t.pname); age=t.age; return *this; } void Disp() { cout<<"Teacher:"<<pname<<","<<age<<endl; } private: char *pname; //存名字的地址 int age; }; class Student { public: Student() { } Student(char *n,int s) { strcpy(name,n); score=s; } void Disp() { cout<<"Student:"<<name<<","<<score<<endl; } protected: char name[10]; int score; }; class Tutor { public: Tutor() { } Tutor(char *n1,int s,char *n2,int a):s(n1,s),t(n2,a) { num=0; } Tutor(Tutor &t):s(t.s),t(t.t) { num=t.num; } void Date() { num++; } void Disp() { s.Disp(); t.Disp(); cout<<"Num:"<<num<<endl; } protected: Student s; Teacher t; int num;//存储师生会面次数 }; int main() { char name1[15],name2[15]; int score,age; cin>>name1>>score>>name2>>age; Tutor tt1(name1,score,name2,age),tt2; tt1.Disp(); tt1.Date(); //师生碰面一次 tt2=tt1; //此处调用的哪段代码? tt2.Disp(); Tutor tt3=tt1; //此处调用的哪段代码? tt3.Date(); tt3.Disp(); return 0; }
时间: 2024-01-17 21:06:09 浏览: 24
修改后的代码如下:
```
#include<iostream>
#include<cstring>
using namespace std;
class Teacher {
public:
Teacher() {
pname = NULL;
age = 0;
}
Teacher(char* p, int a) {
pname = new char[strlen(p) + 1];
strcpy(pname, p);
age = a;
}
Teacher(const Teacher& t) {
pname = new char[strlen(t.pname) + 1];
strcpy(pname, t.pname);
age = t.age;
}
Teacher& operator=(const Teacher& t) {
if(this != &t) {
delete[] pname;
pname = new char[strlen(t.pname) + 1];
strcpy(pname, t.pname);
age = t.age;
}
return *this;
}
~Teacher() {
delete[] pname;
}
void Disp() {
cout << "Teacher:" << pname << "," << age << endl;
}
private:
char* pname;
int age;
};
class Student {
public:
Student() {
name[0] = '\0';
score = 0;
}
Student(char* n, int s) {
strcpy(name, n);
score = s;
}
void Disp() {
cout << "Student:" << name << "," << score << endl;
}
protected:
char name[10];
int score;
};
class Tutor {
public:
Tutor() {
num = 0;
}
Tutor(char* n1, int s, char* n2, int a) : s(n1, s), t(n2, a) {
num = 0;
}
Tutor(const Tutor& t) : s(t.s), t(t.t) {
num = t.num;
}
void Date() {
num++;
}
void Disp() {
s.Disp();
t.Disp();
cout << "Num:" << num << endl;
}
protected:
Student s;
Teacher t;
int num;
};
int main() {
char name1[15], name2[15];
int score, age;
cin >> name1 >> score >> name2 >> age;
Tutor tt1(name1, score, name2, age), tt2;
tt1.Disp();
tt1.Date(); //师生碰面一次
tt2 = tt1; //调用赋值运算符函数
tt2.Disp();
Tutor tt3 = tt1; //调用拷贝构造函数
tt3.Date();
tt3.Disp();
return 0;
}
```
主要修改的地方是:
1. 修改了Teacher类的构造函数、拷贝构造函数、赋值运算符函数和析构函数,保证了对象之间的独立性和数据的正确性。
2. 修改了Student类的构造函数,初始化name数组为空字符串。
3. 修改了Tutor类的构造函数和拷贝构造函数,使用成员初始化列表初始化数据成员。
4. 在main函数中,创建了两个Tutor对象,并测试了赋值运算符函数和拷贝构造函数的正确性。
相关推荐
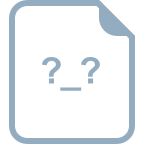















