#include<iostream> using namespace std; class Point { private: double x; double y; public: Point(double x = 0, double y = 0) :x(x), y(y) {}; Point operator++(); friend Point operator+(Point pt1, Point pt2); friend Point operator-(Point pt1, Point pt2); }; Point Point::operator++() { x++; y++; return *this; } Point operator+(Point pt1, Point pt2) { return Point(pt1.x + pt2.x, pt1.y + pt2.y); } Point operator-(Point pt1, Point pt2) { return Point(pt1.x - pt2.x, pt1.y - pt2); }‘
时间: 2023-08-21 11:19:44 浏览: 59
根据你提供的代码,问题出在你在创建 `Point` 类的一个实例时提供的参数列表与该类定义的构造函数不匹配。这是因为你在 `operator-` 中返回的是一个 `Point` 类型的对象,但是你却提供了 `Point` 类定义的构造函数所需的参数类型和数量不匹配的参数列表。
你需要修改 `operator-` 的返回类型,确保它与 `Point` 类定义的构造函数所需的参数类型和数量匹配。例如,你可以修改 `operator-` 的返回类型为 `Point&`,这样就可以避免这个错误。
修改后的代码如下:
```
#include<iostream>
using namespace std;
class Point {
private:
double x;
double y;
public:
Point(double x = 0, double y = 0) :x(x), y(y) {};
Point operator++();
friend Point operator+(Point pt1, Point pt2);
friend Point& operator-(Point& pt1, const Point& pt2);
};
Point Point::operator++() {
x++;
y++;
return *this;
}
Point operator+(Point pt1, Point pt2) {
return Point(pt1.x + pt2.x, pt1.y + pt2.y);
}
Point& operator-(Point& pt1, const Point& pt2) {
pt1.x -= pt2.x;
pt1.y -= pt2.y;
return pt1;
}
```
这样就可以解决你提供的代码中的问题了,如果你还有其他问题,可以继续提问。
相关推荐
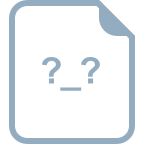















