去重 ,python代码实现
时间: 2023-10-17 14:04:42 浏览: 60
好的,我会用中文回答你的问题并给出 Python 代码的实现。
如果你想去除列表中的重复元素,你可以使用 Python 的内置函数 set()。set() 函数会创建一个无序不重复元素集,可以用来去重。
例如,你可以这样做:
```
# 定义一个列表
my_list = [1, 2, 3, 4, 5, 5, 5, 6, 6, 7, 8, 8]
# 使用 set() 函数去重
my_set = set(my_list)
# 将 set 转换回列表
result = list(my_set)
print(result) # 输出 [1, 2, 3, 4, 5, 6, 7, 8]
```
如果你想保留列表中元素的顺序,你可以使用 collections 模块中的 OrderedDict 类。它能够在保留元素顺序的同时去重。
例如:
```
from collections import OrderedDict
# 定义一个列表
my_list = [1, 2, 3, 4, 5, 5, 5, 6, 6, 7, 8, 8]
# 使用 OrderedDict 类去重
result = list(OrderedDict.fromkeys(my_list))
print(result) # 输出 [1, 2, 3, 4, 5, 6, 7, 8]
```
希望这些信息能帮到你。
相关问题
链表去重代码怎么实现
链表去重通常通过遍历链表并维护一个集合(如哈希集或Set)来实现。以下是Python中基于哈希集合的简单实现:
```python
def remove_duplicates(head):
if not head or not head.next:
return head
seen = set()
current = head
while current:
if current.val in seen:
# 如果当前节点值已经在集合中,删除它
next_node = current.next
current.next = None
current = next_node
else:
seen.add(current.val)
current = current.next
return head
```
在这个代码中,我们从头节点开始,如果遇到的值已经在`seen`集合中,则删除当前节点;否则将该值添加到集合中,并继续遍历。最后返回处理后的头节点。
图片去重 python
可以使用Python中的Pillow库来实现图片去重,具体实现可以参考以下代码:
```
from PIL import Image
import imagehash
# 计算图片的哈希值
def hash_image(image_path):
with Image.open(image_path) as img:
hash = imagehash.average_hash(img)
return hash
# 判断两张图片是否相似
def is_image_similar(image_path1, image_path2, threshold=10):
hash1 = hash_image(image_path1)
hash2 = hash_image(image_path2)
diff = hash1 - hash2
return diff < threshold
# 示例,判断两张图片是否相似
if is_image_similar("image1.jpg", "image2.jpg"):
print("两张图片相似")
else:
print("两张图片不相似")
```
以上代码中,我们使用了Pillow库来加载图片,并使用imagehash库计算图片的哈希值。然后,我们可以通过比较两张图片的哈希值的差异来判断它们是否相似。如果两张图片的哈希值差异小于一个阈值,我们就认为它们是相似的。
阅读全文
相关推荐
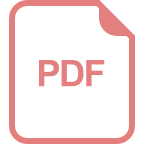
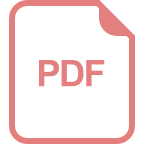
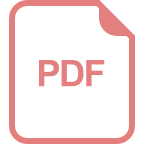













