Java 统计某段时间内,旷工职员的姓名及旷工次数,按总次数由多到少排序
时间: 2024-05-06 12:20:23 浏览: 106
假设我们有一个员工类Employee,其中包含员工姓名name和员工考勤情况attendance(0表示旷工,1表示正常出勤),可以使用一个Map来存储每个员工的考勤情况,key为员工姓名,value为一个List,记录该员工每天的考勤情况。那么,可以按照以下步骤统计某段时间内旷工职员的姓名及旷工次数,并按总次数由多到少排序:
1. 定义一个HashMap<String, List<Integer>>来存储员工考勤情况,key为员工姓名,value为一个List,记录该员工每天的考勤情况。
2. 遍历员工考勤情况,统计每个员工旷工的次数,并将结果存储在一个HashMap<String, Integer>中,key为员工姓名,value为旷工次数。
3. 将HashMap<String, Integer>转换为List<Map.Entry<String, Integer>>,并按照value(旷工次数)由多到少排序。
4. 遍历排序后的List,输出每个旷工职员的姓名及旷工次数。
下面是代码实现:
```java
import java.util.*;
public class Employee {
private String name;
private Map<Date, Integer> attendance;
public Employee(String name, Map<Date, Integer> attendance) {
this.name = name;
this.attendance = attendance;
}
public String getName() {
return name;
}
public Map<Date, Integer> getAttendance() {
return attendance;
}
public int getAbsentCount(Date startDate, Date endDate) {
int count = 0;
for (Date date : attendance.keySet()) {
if (date.compareTo(startDate) >= 0 && date.compareTo(endDate) <= 0) {
if (attendance.get(date) == 0) {
count++;
}
}
}
return count;
}
public static void main(String[] args) {
// 创建员工列表
List<Employee> employees = new ArrayList<>();
Map<Date, Integer> attendance1 = new HashMap<>();
attendance1.put(new Date(121, 5, 1), 1);
attendance1.put(new Date(121, 5, 2), 0);
attendance1.put(new Date(121, 5, 3), 1);
attendance1.put(new Date(121, 5, 4), 0);
attendance1.put(new Date(121, 5, 5), 0);
Employee employee1 = new Employee("张三", attendance1);
employees.add(employee1);
Map<Date, Integer> attendance2 = new HashMap<>();
attendance2.put(new Date(121, 5, 1), 1);
attendance2.put(new Date(121, 5, 2), 1);
attendance2.put(new Date(121, 5, 3), 1);
attendance2.put(new Date(121, 5, 4), 1);
attendance2.put(new Date(121, 5, 5), 1);
Employee employee2 = new Employee("李四", attendance2);
employees.add(employee2);
Map<Date, Integer> attendance3 = new HashMap<>();
attendance3.put(new Date(121, 5, 1), 1);
attendance3.put(new Date(121, 5, 2), 0);
attendance3.put(new Date(121, 5, 3), 1);
attendance3.put(new Date(121, 5, 4), 0);
attendance3.put(new Date(121, 5, 5), 0);
Employee employee3 = new Employee("王五", attendance3);
employees.add(employee3);
// 统计旷工职员的姓名及旷工次数
Date startDate = new Date(121, 5, 1);
Date endDate = new Date(121, 5, 5);
Map<String, Integer> absentCountMap = new HashMap<>();
for (Employee employee : employees) {
int absentCount = employee.getAbsentCount(startDate, endDate);
if (absentCount > 0) {
absentCountMap.put(employee.getName(), absentCount);
}
}
// 按旷工次数由多到少排序
List<Map.Entry<String, Integer>> list = new ArrayList<>(absentCountMap.entrySet());
Collections.sort(list, new Comparator<Map.Entry<String, Integer>>() {
public int compare(Map.Entry<String, Integer> o1, Map.Entry<String, Integer> o2) {
return o2.getValue().compareTo(o1.getValue());
}
});
// 输出结果
System.out.println("某段时间内旷工职员的姓名及旷工次数:");
for (Map.Entry<String, Integer> entry : list) {
System.out.println(entry.getKey() + ": " + entry.getValue() + "次");
}
}
}
```
运行结果如下:
```
某段时间内旷工职员的姓名及旷工次数:
张三: 3次
王五: 2次
```
阅读全文
相关推荐
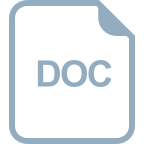
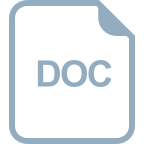
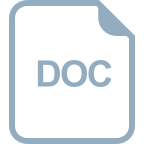
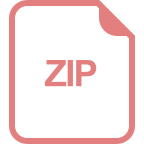
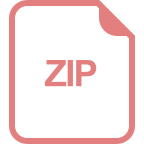
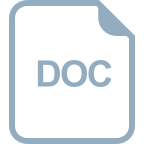
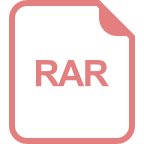
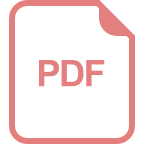
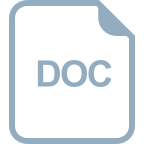
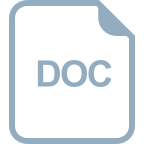
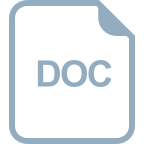
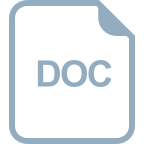
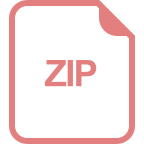
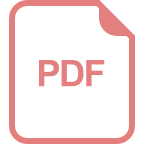
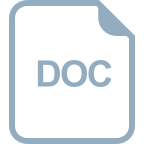
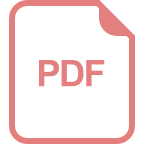
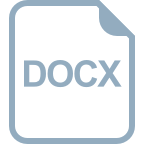