二叉树中值大于x的结点个数python
时间: 2024-02-18 18:00:55 浏览: 26
可以使用递归方式来遍历二叉树,并统计节点值大于x的节点个数。具体实现如下:
```python
class TreeNode:
def __init__(self, val):
self.val = val
self.left = None
self.right = None
def count_nodes(node, x):
if node is None:
return 0
count = 0
if node.val > x:
count = 1
count += count_nodes(node.left, x)
count += count_nodes(node.right, x)
return count
```
其中`TreeNode`是二叉树节点的定义,`count_nodes`是统计节点个数的函数。传入参数`node`表示当前节点,`x`表示给定的值。如果当前节点为`None`,则返回0。否则,统计当前节点的值是否大于`x`,如果是,则计数器加1。然后递归遍历左右子树,将统计的结果累加到计数器中,并返回计数器的值。
相关问题
二叉树中值大于x的结点个数python,写出主程序
好的,以下是一个简单的二叉树实现和计算节点数量的Python主程序:
```python
class Node:
def __init__(self, value):
self.value = value
self.left = None
self.right = None
def insert(root, value):
if root is None:
return Node(value)
if value < root.value:
root.left = insert(root.left, value)
else:
root.right = insert(root.right, value)
return root
def count_nodes(root, x):
if root is None:
return 0
if root.value > x:
return 1 + count_nodes(root.left, x) + count_nodes(root.right, x)
else:
return count_nodes(root.right, x)
# 测试代码
if __name__ == "__main__":
root = None
values = [5, 3, 7, 1, 6, 8]
for v in values:
root = insert(root, v)
x = 4
count = count_nodes(root, x)
print(f"The number of nodes with value > {x} is {count}")
```
该程序首先定义了一个 `Node` 类表示二叉树节点,包括节点值、左右子节点。然后定义了 `insert` 函数用于向二叉树中插入节点。最后定义了 `count_nodes` 函数用于计算二叉树中值大于 `x` 的节点个数。
在主程序中,首先创建了一个空的根节点 `root`,然后向二叉树中插入一些节点。最后调用 `count_nodes` 函数计算二叉树中值大于 `x` 的节点个数,并输出结果。
请注意,这只是一个简单的二叉树实现和计算节点数量的示例,可能并不适用于所有情况。在实际开发中,可能需要根据具体需求进行修改和优化。
二叉树的结点个数Python
二叉树是一种常见的数据结构,它由结点组成,每个结点最多有两个子结点。二叉树的结点个数可以通过递归的方式进行计算。
在Python中,可以使用类来表示二叉树的结点。每个结点包含一个值和指向左右子结点的指针。以下是一个示例代码:
```python
class TreeNode:
def __init__(self, value):
self.value = value
self.left = None
self.right = None
def count_nodes(root):
if root is None:
return 0
else:
return 1 + count_nodes(root.left) + count_nodes(root.right)
```
在上述代码中,`TreeNode`类表示二叉树的结点,`count_nodes`函数用于计算二叉树的结点个数。该函数使用递归的方式,如果当前结点为空,则返回0;否则,返回左子树的结点个数加上右子树的结点个数再加1。
你可以创建一个二叉树,并调用`count_nodes`函数来计算结点个数。以下是一个示例:
```python
# 创建二叉树
root = TreeNode(1)
root.left = TreeNode(2)
root.right = TreeNode(3)
root.left.left = TreeNode(4)
root.left.right = TreeNode(5)
# 计算结点个数
node_count = count_nodes(root)
print("二叉树的结点个数为:", node_count)
```
希望以上信息对你有帮助!如果你有任何其他问题,请随时提问。
相关推荐
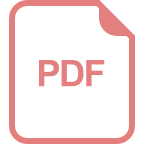












