用C++写一个钟摆小游戏
时间: 2023-12-07 17:49:47 浏览: 25
好的,以下是一个简单的钟摆小游戏的 C++ 代码,希望对您有所帮助:
```cpp
#include <iostream>
#include <cmath>
#include <chrono>
#include <thread>
using namespace std;
const double PI = 3.14159265358979323846; // 圆周率
const int WIDTH = 80; // 游戏窗口宽度
const int HEIGHT = 20; // 游戏窗口高度
const int BAR_LENGTH = 10; // 钟摆杆长
const int BALL_RADIUS = 2; // 钟摆球半径
void draw(int bar_pos, int ball_pos)
{
for (int i = 0; i < HEIGHT; i++)
{
for (int j = 0; j < WIDTH; j++)
{
if (i == 0 || i == HEIGHT - 1 || j == 0 || j == WIDTH - 1)
{
cout << "#"; // 绘制边框
}
else if (j == bar_pos && i >= HEIGHT - BAR_LENGTH)
{
cout << "|"; // 绘制钟摆杆
}
else if (i == HEIGHT - BALL_RADIUS - 1 && abs(j - ball_pos) <= BALL_RADIUS)
{
cout << "O"; // 绘制钟摆球
}
else
{
cout << " "; // 绘制空白
}
}
cout << endl;
}
}
int main()
{
double theta = PI / 4; // 初始角度
double omega = 0; // 初始角速度
const double g = 9.8; // 重力加速度
const double dt = 0.1; // 时间步长
const int bar_pos = WIDTH / 2; // 初始钟摆杆位置
int ball_pos = bar_pos + BAR_LENGTH - BALL_RADIUS - 1; // 初始钟摆球位置
while (true)
{
int x = round(BAR_LENGTH * sin(theta)); // 计算钟摆杆水平位置
ball_pos = bar_pos + x; // 计算钟摆球位置
draw(bar_pos, ball_pos); // 绘制游戏界面
theta += omega * dt; // 更新角度
omega -= g / BAR_LENGTH * sin(theta) * dt; // 更新角速度
this_thread::sleep_for(chrono::milliseconds(100)); // 等待一段时间
system("cls"); // 清屏
}
return 0;
}
```
代码实现的原理是用周期为 $\frac{2\pi}{\sqrt{\frac{g}{L}}}$ 的简谐运动模型模拟钟摆运动,每个时间步长计算角度和角速度,根据钟摆杆长度和角度计算钟摆球位置,然后绘制游戏界面并等待一段时间再清屏,循环执行直到程序结束。
相关推荐
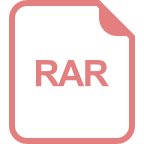



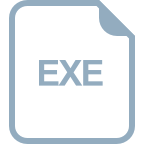
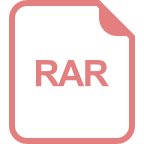
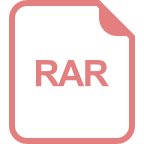
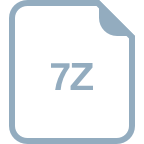
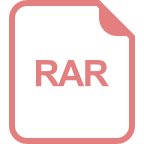
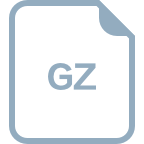