系统中的文本显示类(TextView)和图片显示类(PictureView)都继承了组件类(Component),分别显示文本和图片内容,现需要构造带有滚动条、或者带有黑色边框、或者既有滚动条又有黑色边框的文本显示控件和图片显示控件,但希望最多只增加三个类,
时间: 2023-12-10 20:38:51 浏览: 544
可以采用装饰器模式来实现这个需求。具体地,我们可以定义一个装饰器类(Decorator),用于装饰文本显示类和图片显示类,实现滚动条和黑色边框的功能。具体实现如下:
- 定义组件类(Component),其中包含了显示组件的基本方法display()。
```java
public abstract class Component {
public abstract void display();
}
```
- 定义文本显示类(TextView),继承自组件类,实现文本内容的显示。
```java
public class TextView extends Component {
private String content;
public TextView(String content) {
this.content = content;
}
public void display() {
System.out.println(content);
}
}
```
- 定义图片显示类(PictureView),继承自组件类,实现图片内容的显示。
```java
public class PictureView extends Component {
private String fileName;
public PictureView(String fileName) {
this.fileName = fileName;
}
public void display() {
System.out.println("Displaying picture " + fileName);
}
}
```
- 定义装饰器类(Decorator),继承自组件类,用于实现装饰功能。
```java
public class Decorator extends Component {
private Component component;
public Decorator(Component component) {
this.component = component;
}
public void display() {
component.display();
}
}
```
- 定义滚动条装饰器类(ScrollBarDecorator),继承自装饰器类,实现滚动条的功能。
```java
public class ScrollBarDecorator extends Decorator {
public ScrollBarDecorator(Component component) {
super(component);
}
public void display() {
super.display();
System.out.println("Adding scrollbar...");
}
}
```
- 定义黑色边框装饰器类(BlackBorderDecorator),继承自装饰器类,实现黑色边框的功能。
```java
public class BlackBorderDecorator extends Decorator {
public BlackBorderDecorator(Component component) {
super(component);
}
public void display() {
super.display();
System.out.println("Adding black border...");
}
}
```
- 定义滚动条和黑色边框装饰器类(ScrollBarAndBlackBorderDecorator),继承自装饰器类,实现既有滚动条又有黑色边框的功能。
```java
public class ScrollBarAndBlackBorderDecorator extends Decorator {
public ScrollBarAndBlackBorderDecorator(Component component) {
super(component);
}
public void display() {
super.display();
System.out.println("Adding scrollbar and black border...");
}
}
```
使用示例:
```java
Component textView = new TextView("This is a text view.");
Component pictureView = new PictureView("picture.jpg");
Component textViewWithScrollBar = new ScrollBarDecorator(textView);
Component textViewWithBlackBorder = new BlackBorderDecorator(textView);
Component textViewWithScrollBarAndBlackBorder = new ScrollBarAndBlackBorderDecorator(textView);
Component pictureViewWithScrollBar = new ScrollBarDecorator(pictureView);
Component pictureViewWithBlackBorder = new BlackBorderDecorator(pictureView);
Component pictureViewWithScrollBarAndBlackBorder = new ScrollBarAndBlackBorderDecorator(pictureView);
textViewWithScrollBar.display();
textViewWithBlackBorder.display();
textViewWithScrollBarAndBlackBorder.display();
pictureViewWithScrollBar.display();
pictureViewWithBlackBorder.display();
pictureViewWithScrollBarAndBlackBorder.display();
```
输出结果:
```
This is a text view.
Adding scrollbar...
This is a text view.
Adding black border...
This is a text view.
Adding scrollbar and black border...
Displaying picture picture.jpg
Adding scrollbar...
Displaying picture picture.jpg
Adding black border...
Displaying picture picture.jpg
Adding scrollbar and black border...
```
阅读全文
相关推荐
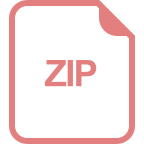



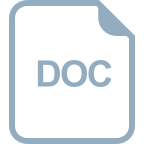
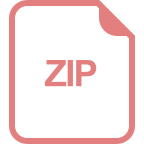
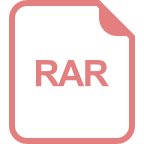
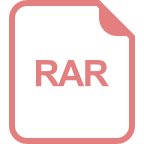
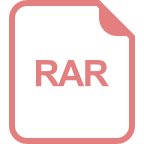
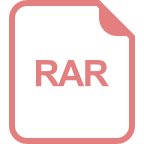
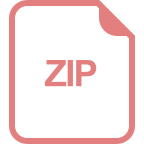
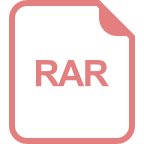
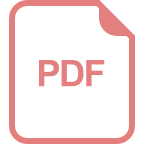
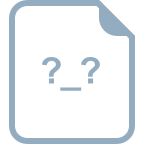
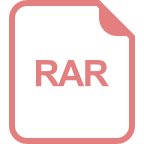