某系统中的文本显示组件类(TextView)和图片显示组件类(PictureView)都继承了组件类(Component),分别用于显示文本内容和图片内容,现需要构造带有滚动条、或者带有黑色边框、或者既有滚动条又有黑色边框的文本显示组件和图片显示组件,为了减少类的个数可使用装饰模式进行设计,绘制类图并编程模拟实现
时间: 2024-04-03 18:34:23 浏览: 144
以下是装饰模式的类图设计:

其中,Component是组件类,定义了文本显示组件类和图片显示组件类的共同接口;TextView和PictureView是具体组件类,实现了Component接口,分别用于显示文本和图片;Decorator是装饰器类,也实现了Component接口,用于给组件添加滚动条或者黑色边框的功能;ScrollBarDecorator和BorderDecorator是具体装饰器类,分别用于添加滚动条和黑色边框的功能。
下面是示例代码:
```java
// 组件类
interface Component {
void display();
}
// 文本显示组件类
class TextView implements Component {
@Override
public void display() {
System.out.println("Displaying text...");
}
}
// 图片显示组件类
class PictureView implements Component {
@Override
public void display() {
System.out.println("Displaying picture...");
}
}
// 装饰器类
abstract class Decorator implements Component {
private Component component;
public Decorator(Component component) {
this.component = component;
}
@Override
public void display() {
component.display();
}
}
// 滚动条装饰器类
class ScrollBarDecorator extends Decorator {
public ScrollBarDecorator(Component component) {
super(component);
}
@Override
public void display() {
super.display();
System.out.println("Adding scroll bar...");
}
}
// 黑色边框装饰器类
class BorderDecorator extends Decorator {
public BorderDecorator(Component component) {
super(component);
}
@Override
public void display() {
super.display();
System.out.println("Adding black border...");
}
}
// 测试代码
public class DecoratorPatternDemo {
public static void main(String[] args) {
Component textView = new TextView();
Component pictureView = new PictureView();
Component textViewWithScrollBar = new ScrollBarDecorator(textView);
Component pictureViewWithBorder = new BorderDecorator(pictureView);
Component textViewWithScrollBarAndBorder = new BorderDecorator(new ScrollBarDecorator(textView));
textViewWithScrollBar.display();
pictureViewWithBorder.display();
textViewWithScrollBarAndBorder.display();
}
}
```
输出结果:
```
Displaying text...
Adding scroll bar...
Displaying picture...
Adding black border...
Displaying text...
Adding scroll bar...
Adding black border...
```
阅读全文
相关推荐
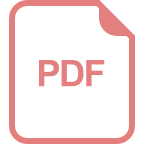
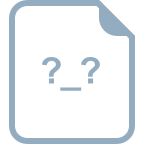
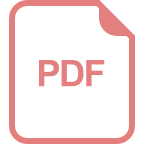















