vue3父子组件的实现方式
时间: 2023-10-19 18:16:26 浏览: 48
在 Vue3 中,可以通过以下两种方式实现父子组件通信:
1. Props + Emit
父组件可以通过 props 给子组件传递数据,子组件可以通过 emit 触发自定义事件向父组件传递数据。
父组件代码:
```html
<template>
<child-component :message="parentMessage" @update:message="handleUpdateMessage"></child-component>
</template>
<script>
import ChildComponent from './ChildComponent.vue';
export default {
components: {
ChildComponent,
},
data() {
return {
parentMessage: 'Hello from Parent',
};
},
methods: {
handleUpdateMessage(message) {
this.parentMessage = message;
},
},
};
</script>
```
子组件代码:
```html
<template>
<div>
<p>{{ message }}</p>
<button @click="handleClick">Update Message</button>
</div>
</template>
<script>
export default {
props: {
message: String,
},
methods: {
handleClick() {
this.$emit('update:message', 'Hello from Child');
},
},
};
</script>
```
2. Provide + Inject
父组件可以通过 provide 提供数据,子组件可以通过 inject 注入数据。
父组件代码:
```html
<template>
<child-component></child-component>
</template>
<script>
import ChildComponent from './ChildComponent.vue';
export default {
components: {
ChildComponent,
},
provide() {
return {
parentMessage: 'Hello from Parent',
};
},
};
</script>
```
子组件代码:
```html
<template>
<div>
<p>{{ message }}</p>
<button @click="handleClick">Update Message</button>
</div>
</template>
<script>
export default {
inject: ['parentMessage'],
data() {
return {
message: this.parentMessage,
};
},
methods: {
handleClick() {
this.message = 'Hello from Child';
},
},
};
</script>
```
以上两种方式都可以实现父子组件通信,具体选择哪种方式取决于实际需求和个人喜好。
相关推荐
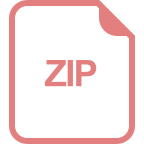
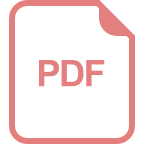
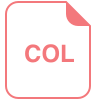
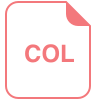
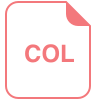
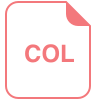
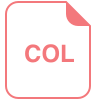









