Vue组件间通信方式及最佳实践
发布时间: 2023-12-20 12:27:03 阅读量: 15 订阅数: 12 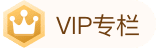
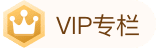
# 第一章:Vue组件通信概述
## 1.1 Vue组件通信的重要性
Vue是一款流行的JavaScript框架,采用组件化开发,因此组件间通信至关重要。在实际开发中,不同组件之间需要频繁地传递数据、触发事件以及共享状态。深入理解Vue组件通信的方式和最佳实践对于构建健壮的Vue应用至关重要。
## 1.2 父子组件通信
在Vue中,父子组件的通信是最常见的情况之一。父组件通过props向子组件传递数据,子组件通过$emit来触发事件通知父组件。
```javascript
// ParentComponent.vue
<template>
<child-component :message="parentMessage" @notify="handleNotify"></child-component>
</template>
<script>
import ChildComponent from './ChildComponent.vue';
export default {
components: {
ChildComponent
},
data() {
return {
parentMessage: 'Message from parent'
};
},
methods: {
handleNotify(message) {
console.log('Received message from child:', message);
}
}
}
</script>
// ChildComponent.vue
<template>
<div>
<button @click="notifyParent">Send Message to Parent</button>
</div>
</template>
<script>
export default {
props: ['message'],
methods: {
notifyParent() {
this.$emit('notify', 'Message from child');
}
}
}
</script>
```
## 1.3 兄弟组件通信
兄弟组件之间的通信需要借助共同的父组件或者通过事件总线(Event Bus)来实现数据传递和状态管理。在兄弟组件之间进行通信时,需要注意数据的单向流动,避免直接修改兄弟组件的状态。
```javascript
// ParentComponent.vue
<template>
<div>
<first-sibling @change="handleChange"></first-sibling>
<second-sibling :value="sharedValue"></second-sibling>
</div>
</template>
<script>
export default {
data() {
return {
sharedValue: ''
};
},
methods: {
handleChange(value) {
this.sharedValue = value;
}
}
}
</script>
// FirstSibling.vue
<template>
<input v-model="inputValue" @input="updateSibling">
</template>
<script>
export default {
data() {
return {
inputValue: ''
};
},
methods: {
updateSibling() {
this.$emit('change', this.inputValue);
}
}
}
</script>
// SecondSibling.vue
<template>
<div>
<p>{{ value }}</p>
</div>
</template>
<script>
export default {
props: ['value']
}
</script>
```
## 1.4 跨级组件通信
当组件层级较深时,可能需要在不直接相关的组件之间进行通信。这时可以使用事件总线(Event Bus)或者Vuex来实现跨级组件的通信。
```javascript
// EventBus.js
import Vue from 'vue';
export const EventBus = new Vue();
// SenderComponent.vue
<template>
<button @click="sendMessage">Send Message</button>
</template>
<script>
import { EventBus } from './EventBus.js';
export default {
methods: {
sendMessage() {
EventBus.$emit('message', 'Hello from SenderComponent');
}
}
}
</script>
// ReceiverComponent.vue
<template>
<div>
<p>{{ message }}</p>
</div>
</template>
<script>
import { EventBus } from './EventBus.js';
export default {
data() {
return {
message: ''
};
},
created() {
EventBus.$on('message', (value) => {
this.message = value;
});
}
}
</script>
```
## 1.5 非父子组件通信
有些情况下,组件之间没有明显的层级关系,此时也可以借助事件总线(Event Bus)、Vuex或者通过共同祖先组件来实现通信。
## 2. 第二章:Props和$emit的使用
在Vue组件通信中,Props和$emit是非常常用的方式,用于实现父子组件之间的通信。本章将详细介绍Props和$emit的使用方法和最佳实践。
### 2.1 Props属性传递
在Vue组件中,我们可以使用Props属性来向子组件传递数据。父组件可以通过Props向子组件传递任意类型的数据,包括基本类型、对象、数组等。子组件可以通过定义Props来接收这些数据,并在组件内部进行处理和渲染。
```javascript
// ParentComponent.vue
<template>
<ChildComponent :message="parentMessage" />
</template>
<script>
import ChildComponent from './ChildComponent.vue';
export default {
data() {
return {
parentMessage: 'Hello from parent component'
};
},
components: {
ChildComponent
}
};
</script>
// ChildComponent.vue
<template>
<div>
{{ message }}
</div>
</template>
<script>
export default {
props: ['message']
};
</script>
```
在上面的例子中,父组件通过Props向子组件传递了`parentMessage`,子组件通过定义Props来接收并渲染这个数据。在实际开发中,Props属性的传递可以帮助我们实现父子组件之间的数据共享和通信。
### 2.2 使用$emit触发事件
除了Props属性传递,Vue组件通信还可以通过$emit触发自定义事件,实现子组件向父组件传递数据的目的。子组件可以通过$emit触发事件,并将需要传递的数据作为参数传递到父组件。
```javascript
// ChildComponent.vue
<template>
<button @click="sendMessageToParent">Send Message to Parent</button>
</template>
<script>
export default {
methods: {
sendMessageToParent() {
this.$emit('messageToParent', 'Hello from child component');
}
}
};
</script>
// ParentComponent.vue
<template>
<div>
<ChildComponent @messageToParent="receiveMessageFromChild" />
</div>
</template>
<script>
import ChildComponent from './ChildComponent.vue';
export default {
methods: {
receiveMessageFromChild(message) {
console.log(message); // Output: Hello from child component
}
},
components: {
ChildComponent
}
};
</script>
```
### 第三章:$parent和$children的使用
在Vue中,$parent和$children是两个指向父级组件和子级组件的引用,可以用于实现父子组件之间的通信。
#### 3.1 使用$parent进行父组件通信
通过$parent可以直接访问父级组件实例,从而调用父组件的方法或访问父组件的数据。这种方式适用于父子组件关系比较简单且层级不深的情况下。
```javascript
// ParentComponent.vue
<template>
<div>
<ChildComponent></ChildComponent>
</div>
</template>
<script>
import ChildComponent from './ChildComponent.vue';
export default {
components: {
ChildComponent
},
methods: {
greetParent() {
console.log('Hello from the parent component!');
}
}
}
</script>
```
```javascript
// ChildComponent.vue
<template>
<div>
<button @click="callParentMethod">Call Parent Method</button>
</div>
</template>
<script>
export default {
methods: {
callParentMethod() {
this.$parent.greetParent();
}
}
}
</script>
```
#### 3.2 使用$children进行子组件通信
通过$children可以直接访问子级组件实例,从而调用子组件的方法或访问子组件的数据。需要注意的是,$children返回的是一个数组,因为一个父组件可能包含多个子组件。
```javascript
// ParentComponent.vue
<template>
<div>
<button @click="callChildMethod">Call Child Method</button>
<ChildComponent ref="child1"></ChildComponent>
<ChildComponent ref="child2"></ChildComponent>
</div>
</template>
<script>
import ChildComponent from './ChildComponent.vue';
export default {
components: {
ChildComponent
},
methods: {
callChildMethod() {
this.$children.forEach(child => {
child.greetChild();
});
}
}
}
</script>
```
```javascript
// ChildComponent.vue
<template>
<div>
<!-- Child component content -->
</div>
</template>
<script>
export default {
methods: {
greetChild() {
console.log('Hello from the child component!');
}
}
}
</script>
```
#### 3.3 $parent和$children的注意事项
使用$parent和$children虽然可以实现父子组件的通信,但需要注意以下几点:
- $parent和$children是Vue的内置属性,但在复杂的组件结构中使用时可能会导致代码耦合度高,不利于组件的维护和重用。
- 使用$parent和$children时要确保父子组件之间的关系是稳定的,否则可能会导致意外的错误,尤其是在组件层级较多的情况下。
总的来说,$parent和$children适用于简单的父子组件通信,但在复杂的组件结构中,建议使用其他更灵活的通信方式,如Props和$emit、VueX或Event Bus。
### 4. 第四章:使用VueX进行组件状态管理
在Vue应用程序中,组件状态管理是一个非常关键的问题。通常情况下,多个组件共享同一个状态或者需要进行状态的同步更新。VueX是一个专门为Vue.js应用程序开发的状态管理模式。
在这一章节中,我们将深入探讨VueX的基本概念、在组件中如何使用VueX以及VueX的最佳实践。
#### 4.1 VueX的基本概念
VueX基于Flux架构,主要包含了state、getters、mutations、actions和modules几个核心概念。
- **State**:即应用程序中需要管理的状态数据,类似于React中的state。在VueX中,所有的状态都存储在一个单一的state对象中。
- **Getters**:从state中派生出一些状态,类似于Vue组件中的计算属性。可以在getters中进行一些复杂的状态计算或过滤操作。
- **Mutations**: mutations是唯一允许修改state的地方,类似于事件,但是它是同步的。每个mutation都有一个字符串的事件类型(type)和一个回调函数(handler)。
- **Actions**:actions提交的是mutations,而不是直接变更状态,可以包含任意异步操作。actions可以被组件中的methods调用。
- **Modules**:允许将store分割成模块,每个模块拥有自己的state、getters、mutations、actions,比较适用于大型应用。
#### 4.2 在组件中使用VueX
为了在组件中使用VueX,我们首先需要创建一个store对象,并在根组件中注入该store。然后在组件中可以通过`this.$store`访问到该store对象,进而访问到state、getters、mutations和actions。
```javascript
// store.js
import Vue from 'vue'
import Vuex from 'vuex'
Vue.use(Vuex)
const store = new Vuex.Store({
state: {
count: 0
},
mutations: {
increment (state) {
state.count++
}
}
})
export default store
// main.js
import Vue from 'vue'
import App from './App.vue'
import store from './store'
new Vue({
el: '#app',
store,
render: h => h(App)
})
// Counter.vue
<template>
<div>
<p>{{ $store.state.count }}</p>
<button @click="incrementCount">Increment</button>
</div>
</template>
<script>
export default {
methods: {
incrementCount () {
this.$store.commit('increment')
}
}
}
</script>
```
#### 4.3 使用VueX的最佳实践
- 将store和根实例分离:将store对象的创建和注入与根实例分离,可以使得在测试或者在不同环境中更容易地维护和管理。
- 使用mapState、mapGetters、mapMutations和mapActions辅助函数:这些辅助函数可以帮助我们在组件中更方便地访问state、getters、mutations和actions,减少代码冗余。
- 将actions中的异步操作抽离出来:将异步操作放到actions中,并且在组件中只保留对actions的调用,可以使得代码更具有可维护性和可测试性。
VueX的引入可以帮助我们更好地进行组件状态管理,保持组件间的数据同步。但是在应用VueX时,也需要根据实际情况选择合适的应用方式。
### 5. 第五章:Event Bus的使用
在Vue应用中,Event Bus是一种非常便利的方式,用于在不同组件之间进行通信。它可以让我们在任何地方发布事件和监听事件,从而实现组件之间的解耦。然而,在实际开发中,它也存在一些使用上的注意事项和适用场景。
#### 5.1 创建和使用Event Bus
首先,我们需要创建一个Vue实例作为我们的Event Bus:
```javascript
// event-bus.js
import Vue from 'vue';
export const EventBus = new Vue();
```
然后,在需要通信的组件中,我们可以通过Event Bus来发布事件和监听事件:
```javascript
// ComponentA.vue
import { EventBus } from './event-bus.js';
export default {
created() {
EventBus.$on('custom-event', this.handleCustomEvent);
},
methods: {
handleCustomEvent(payload) {
// 处理接收到的事件
},
triggerEvent() {
EventBus.$emit('custom-event', payload);
}
}
}
```
```javascript
// ComponentB.vue
import { EventBus } from './event-bus.js';
export default {
created() {
EventBus.$on('custom-event', this.handleCustomEvent);
},
methods: {
handleCustomEvent(payload) {
// 处理接收到的事件
}
}
}
```
#### 5.2 Event Bus的优点和缺点
##### 优点:
- 简单易用:Event Bus的创建和使用非常简单,可以快速实现组件间的通信。
- 解耦组件:组件不需要直接引用彼此,通过Event Bus进行通信,可以降低组件之间的耦合度。
##### 缺点:
- 全局事件:事件总线是一个全局实例,因此可能导致事件的命名冲突和不可预测的事件流。
- 组件关系不明显:Event Bus的使用会使得组件之间的关系不够明显,可能会增加代码的维护难度。
#### 5.3 Event Bus的适用场景
Event Bus适合于简单的父子组件通信或者跨级组件通信,特别是在中小型项目中可以快速解决一些简单的状态共享和通信问题。但在大型项目中,由于其全局性质和潜在的命名冲突问题,建议谨慎使用,可以考虑使用Vuex进行更为严谨的状态管理。
## 6. 第六章:总结与最佳实践
在本文中,我们深入探讨了Vue组件间通信的多种方式及其最佳实践。通过对Props和$emit、$parent和$children、VueX以及Event Bus的使用进行详细分析,我们可以得出一些最佳实践和总结如下:
### 6.1 Vue组件通信最佳实践总结
- 对于父子组件通信,推荐使用Props属性传递数据和$emit触发事件的方式,这样可以简单直接地进行数据传递和事件的处理。
- 当涉及兄弟组件通信时,推荐使用共同的父组件作为中转站,通过Props和$emit进行数据和事件的传递。
- 跨级组件通信可以通过$parent和$children来实现,但应该避免过多地依赖这种方式,以免造成组件间的耦合过重。
- 对于非父子组件通信,VueX和Event Bus是更为合适的选择,具体选择取决于项目的规模和复杂度。
### 6.2 使用不同通信方式的场景分析
- 当项目较小且组件嵌套不深时,可以优先考虑使用Props和$emit进行父子组件通信,简单易行。
- 对于大型复杂项目,特别是涉及跨级或非父子组件通信时,推荐使用VueX进行组件状态管理,以便统一管理组件间的状态和数据流动。
- 在某些简单的场景中,Event Bus可以作为一种快速解决方案,但需要注意潜在的事件命名冲突和管理不当可能导致的混乱。
### 6.3 在项目中的实际应用建议
- 在实际项目中,应根据具体需求和场景选择合适的组件通信方式,不要死板地套用某种方式,而是灵活应用各种通信方式以最优化整体架构。
- 对于新项目,及时进行需求分析和架构设计,选择合适的组件通信方式并在团队中进行合理的规范约定,将有助于项目整体的可维护性和扩展性。
0
0
相关推荐
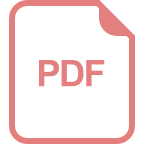
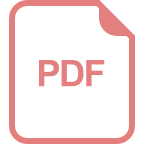





