定义一个学生类,它包含学号(int)、姓名(string)、性别(sex)、分数(float)等数据成员,定义一个对象,重载赋值运算符“=”,使“=”能够实现int、string、float型的数据为该对象赋值;重载运算符“<<”“>>”,使之能够直接输入、输出学生类的对象。
时间: 2024-03-26 10:35:15 浏览: 68
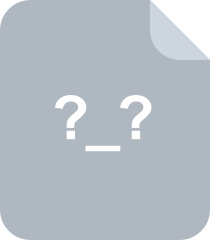
一个 Student类:包括姓名、学号、性别、专业、家庭地址。
好的,下面是定义一个学生类并实现相关功能的示例代码:
```c++
#include <iostream>
#include <string>
using namespace std;
enum Sex { Male, Female }; // 定义枚举类型Sex表示性别
class Student {
public:
Student() = default; // 默认构造函数
Student(int num, string name, Sex sex, float score) :
number(num), name(name), sex(sex), score(score) {} // 带参构造函数
// 重载赋值运算符"="
Student& operator=(const int& num) {
number = num;
return *this;
}
Student& operator=(const string& n) {
name = n;
return *this;
}
Student& operator=(const float& s) {
score = s;
return *this;
}
// 重载输入输出运算符">>"和"<<"
friend istream& operator>>(istream& in, Student& s) {
in >> s.number >> s.name >> s.sex >> s.score;
return in;
}
friend ostream& operator<<(ostream& out, const Student& s) {
out << s.number << " " << s.name << " " << s.sex << " " << s.score << endl;
return out;
}
private:
int number;
string name;
Sex sex;
float score;
};
int main() {
Student s;
int num = 1001;
string name = "Tom";
float score = 89.5;
s = num;
s = name;
s = score;
cout << "请输入学生的学号、姓名、性别和分数:" << endl;
cin >> s;
cout << "学生信息如下:" << endl;
cout << s;
return 0;
}
```
这个程序定义了一个类`Student`,其中包含了学号、姓名、性别和分数四个数据成员。我们重载了赋值运算符“=”,使之能够实现int、string、float型的数据为该对象赋值;重载运算符“<<”“>>”,使之能够直接输入、输出学生类的对象。在`main`函数中,我们对一个`Student`对象`s`进行了赋值和输入输出操作。
阅读全文
相关推荐
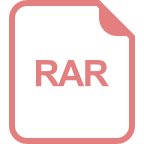
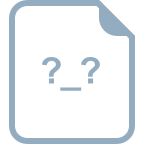














