在JavaScript中,什么是promise、怎么使用promise、怎么手写promise
时间: 2023-10-14 22:13:31 浏览: 45
1. 什么是promise?
Promise是一种异步编程的解决方案,它是ES6新增的特性,用于解决回调地狱的问题。Promise代表一个异步操作的最终完成状态(成功或失败)以及返回的结果值。
2. 怎么使用promise?
使用Promise需要以下几个步骤:
(1)创建Promise对象,将异步任务封装在Promise对象中。
(2)使用then方法处理成功状态的结果。
(3)使用catch方法处理失败状态的结果。
例如:
```javascript
let promise = new Promise(function(resolve, reject) {
setTimeout(() => {
if (Math.random() < 0.5) {
resolve('success');
} else {
reject('fail');
}
}, 1000);
});
promise.then(function(result) {
console.log(result); // 'success'
}).catch(function(error) {
console.log(error); // 'fail'
});
```
3. 怎么手写promise?
手写Promise需要实现以下几个步骤:
(1)创建Promise类,定义构造函数,初始化Promise状态。
(2)定义resolve方法和reject方法,分别用于改变Promise状态为成功和失败。
(3)定义then方法和catch方法,分别用于处理成功状态和失败状态。
(4)实现链式调用,即then方法可以无限调用。
例如:
```javascript
class MyPromise {
constructor(executor) {
this.status = 'pending';
this.value = undefined;
this.reason = undefined;
let resolve = (value) => {
if (this.status === 'pending') {
this.status = 'fulfilled';
this.value = value;
}
};
let reject = (reason) => {
if (this.status === 'pending') {
this.status = 'rejected';
this.reason = reason;
}
};
try {
executor(resolve, reject);
} catch (error) {
reject(error);
}
}
then(onFulfilled, onRejected) {
if (this.status === 'fulfilled') {
onFulfilled(this.value);
} else if (this.status === 'rejected') {
onRejected(this.reason);
}
return this;
}
catch(onRejected) {
if (this.status === 'rejected') {
onRejected(this.reason);
}
return this;
}
}
```
使用手写的Promise:
```javascript
let promise = new MyPromise(function(resolve, reject) {
setTimeout(() => {
if (Math.random() < 0.5) {
resolve('success');
} else {
reject('fail');
}
}, 1000);
});
promise.then(function(result) {
console.log(result); // 'success'
}).catch(function(error) {
console.log(error); // 'fail'
});
```
相关推荐
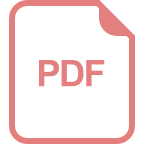
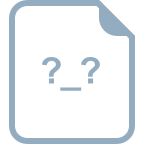














