igh ethercat步进例程
时间: 2023-06-12 13:07:35 浏览: 219
以下是使用 EtherCAT 协议控制步进电机的示例代码:
```c
#include "ecrt.h"
#define MOTOR_CHANNEL 0
#define MOTOR_POSITION 0x6064
#define MOTOR_PROFILE_VELOCITY 0x6081
#define MOTOR_TARGET_VELOCITY 0x60FF
#define MOTOR_MODE_OF_OPERATION 0x6060
#define MOTOR_CONTROL_WORD 0x6040
#define MOTOR_STATUS_WORD 0x6041
#define MOTOR_MAX_PROFILE_VELOCITY 3000
#define MOTOR_PROFILE_ACCELERATION 5000
#define MOTOR_PROFILE_DECELERATION 5000
#define MOTOR_TARGET_POSITION 10000
int main()
{
ec_master_t *master = ecrt_request_master(0);
ec_slave_config_t *sc_motor = ecrt_slave_config_create(0, MOTOR_CHANNEL);
ec_slave_config_pdos_clear(sc_motor);
// 配置输入对象字典
ec_pdo_entry_info_t motor_inputs[] = {
{MOTOR_STATUS_WORD, 0x10, EC_SIZE_UINT16},
{MOTOR_POSITION, 0x10, EC_SIZE_INT32},
{0}
};
ecrt_slave_config_pdos_add(sc_motor, EC_DIR_INPUT, motor_inputs);
// 配置输出对象字典
ec_pdo_entry_info_t motor_outputs[] = {
{MOTOR_CONTROL_WORD, 0x0f, EC_SIZE_UINT16},
{MOTOR_MODE_OF_OPERATION, 0x0f, EC_SIZE_UINT8},
{MOTOR_TARGET_VELOCITY, 0x0f, EC_SIZE_INT32},
{0}
};
ecrt_slave_config_pdos_add(sc_motor, EC_DIR_OUTPUT, motor_outputs);
if (ecrt_slave_config_reg_pdo_entry(sc_motor, MOTOR_CONTROL_WORD, 0, &motor_control_word) ||
ecrt_slave_config_reg_pdo_entry(sc_motor, MOTOR_MODE_OF_OPERATION, 0, &motor_mode_of_operation) ||
ecrt_slave_config_reg_pdo_entry(sc_motor, MOTOR_TARGET_VELOCITY, 0, &motor_target_velocity))
{
printf("Failed to register PDO entries for MOTOR\n");
return -1;
}
if (ecrt_master_activate(master))
{
printf("Failed to activate EtherCAT master\n");
return -1;
}
ec_slave_config_pdos_update(sc_motor);
// 进入电机操作模式
uint16_t motor_mode = 9;
if (ecrt_master_write(master, MOTOR_CHANNEL, MOTOR_MODE_OF_OPERATION, 0, &motor_mode, sizeof(motor_mode)))
{
printf("Failed to set motor mode of operation\n");
return -1;
}
// 设置电机最大速度
int32_t max_velocity = MOTOR_MAX_PROFILE_VELOCITY;
if (ecrt_master_write(master, MOTOR_CHANNEL, MOTOR_PROFILE_VELOCITY, 0, &max_velocity, sizeof(max_velocity)))
{
printf("Failed to set motor max profile velocity\n");
return -1;
}
// 设置加速度和减速度
int32_t profile_acceleration = MOTOR_PROFILE_ACCELERATION;
int32_t profile_deceleration = MOTOR_PROFILE_DECELERATION;
if (ecrt_master_write(master, MOTOR_CHANNEL, 0x6083, 0, &profile_acceleration, sizeof(profile_acceleration)) ||
ecrt_master_write(master, MOTOR_CHANNEL, 0x6084, 0, &profile_deceleration, sizeof(profile_deceleration)))
{
printf("Failed to set profile acceleration and deceleration\n");
return -1;
}
// 发送电机目标位置
int32_t target_position = MOTOR_TARGET_POSITION;
if (ecrt_master_write(master, MOTOR_CHANNEL, MOTOR_TARGET_POSITION, 0, &target_position, sizeof(target_position)))
{
printf("Failed to set motor target position\n");
return -1;
}
// 启动电机运动
uint16_t control_word = 0x0006;
if (ecrt_master_write(master, MOTOR_CHANNEL, MOTOR_CONTROL_WORD, 0, &control_word, sizeof(control_word)))
{
printf("Failed to start motor motion\n");
return -1;
}
// 循环读取电机状态
uint16_t status_word;
int32_t position;
while (1)
{
ecrt_master_read(master, MOTOR_CHANNEL, MOTOR_STATUS_WORD, 0, &status_word, sizeof(status_word));
ecrt_master_read(master, MOTOR_CHANNEL, MOTOR_POSITION, 0, &position, sizeof(position));
if (status_word & 0x100)
{
printf("Motor reached target position!\n");
break;
}
}
// 停止电机运动
control_word = 0x0000;
if (ecrt_master_write(master, MOTOR_CHANNEL, MOTOR_CONTROL_WORD, 0, &control_word, sizeof(control_word)))
{
printf("Failed to stop motor motion\n");
return -1;
}
ecrt_release_master(master);
return 0;
}
```
这段代码使用 EtherCAT 协议控制步进电机,实现了设置电机运动模式、最大速度、加速度、减速度和目标位置,并启动电机运动,等待电机到达目标位置后停止运动。这其中的具体实现和配置需要根据实际硬件设备和 EtherCAT 网络的具体情况进行调整和修改。
阅读全文
相关推荐
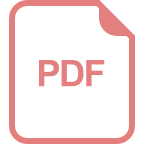
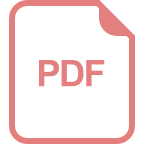


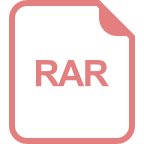
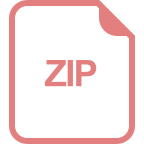
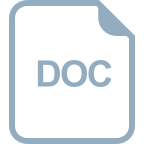



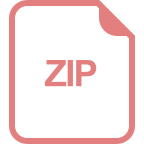
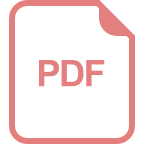
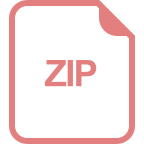




