igh ethercat雷赛步进例程
时间: 2023-09-11 10:05:03 浏览: 287
以下是使用EtherCAT总线控制雷赛步进电机的简单示例代码:
```
#include <ethercat.h>
#include <stdio.h>
#define NUM_OF_SLAVES 1
#define POS_MODE 0x08 // Set to position mode
#define MAX_POS 200000 // Maximum position
uint8_t inbuf[32*NUM_OF_SLAVES];
uint8_t outbuf[32*NUM_OF_SLAVES];
int main(int argc, char **argv) {
int expected_wkc;
int slave;
uint16_t status;
uint32_t position;
int32_t target_pos;
// Initialize EtherCAT master
if (ec_init("enp0s31f6") > 0) {
printf("ec_init on enp0s31f6 succeeded.\n");
} else {
printf("ec_init on enp0s31f6 failed.\n");
return -1;
}
// Set number of slaves
if (ec_config_init(FALSE) > 0) {
printf("%d slaves found and configured.\n", ec_slavecount);
} else {
printf("No slaves found.\n");
ec_close();
return -1;
}
// Check if there is only one slave
if (ec_slavecount != NUM_OF_SLAVES) {
printf("Error: Expected %d slaves but found %d.\n", NUM_OF_SLAVES, ec_slavecount);
ec_close();
return -1;
}
// Configure slave
for (slave = 1; slave <= ec_slavecount; slave++) {
// Set slave to operational state
ec_slave[slave].state = EC_STATE_OPERATIONAL;
ec_slave[slave].ALstatuscode = 0;
// Request state transition
ec_slave[slave].state_request = EC_STATE_OPERATIONAL;
ec_writestate(slave);
// Wait for state transition
ec_statecheck(slave, EC_STATE_OPERATIONAL, EC_TIMEOUTSTATE);
if (ec_slave[slave].state != EC_STATE_OPERATIONAL) {
printf("Slave %d did not reach operational state.\n", slave);
ec_close();
return -1;
}
// Read slave status
ec_readstate();
status = ec_slave[slave].state;
printf("Slave %d status: %d\n", slave, status);
// Set slave to position mode
outbuf[0] = POS_MODE;
expected_wkc = ec_SDOwrite(slave, 0x6060, 0x00, FALSE, 1, outbuf, EC_TIMEOUTRXM);
// Set maximum position
position = MAX_POS;
outbuf[0] = (uint8_t)(position & 0x000000FF);
outbuf[1] = (uint8_t)((position >> 8) & 0x000000FF);
outbuf[2] = (uint8_t)((position >> 16) & 0x000000FF);
outbuf[3] = (uint8_t)((position >> 24) & 0x000000FF);
expected_wkc += ec_SDOwrite(slave, 0x607A, 0x00, FALSE, 4, outbuf, EC_TIMEOUTRXM);
// Enable slave
outbuf[0] = 0x0F;
expected_wkc += ec_SDOwrite(slave, 0x6040, 0x00, FALSE, 1, outbuf, EC_TIMEOUTRXM);
if (expected_wkc != (3 * NUM_OF_SLAVES)) {
printf("Error: Expected %d write operations but %d were executed.\n", (3 * NUM_OF_SLAVES), expected_wkc);
ec_close();
return -1;
}
}
// Set target position to 0
target_pos = 0;
// Loop until target position is reached
while (1) {
// Read current position
ec_readstate();
position = (ec_slave[1].inputs[0]) | (ec_slave[1].inputs[1] << 8) | (ec_slave[1].inputs[2] << 16) | (ec_slave[1].inputs[3] << 24);
// Print current position
printf("Current position: %d\n", position);
// Check if target position is reached
if (position == target_pos) {
printf("Target position reached.\n");
break;
}
// Set target position
outbuf[0] = (uint8_t)(target_pos & 0x000000FF);
outbuf[1] = (uint8_t)((target_pos >> 8) & 0x000000FF);
outbuf[2] = (uint8_t)((target_pos >> 16) & 0x000000FF);
outbuf[3] = (uint8_t)((target_pos >> 24) & 0x000000FF);
expected_wkc = ec_SDOwrite(slave, 0x607A, 0x00, FALSE, 4, outbuf, EC_TIMEOUTRXM);
// Wait for position update
ec_send_processdata();
ec_receive_processdata(EC_TIMEOUTRET);
// Increment target position
target_pos += 1000;
if (target_pos > MAX_POS) {
target_pos = 0;
}
}
// Set slave to pre-operational state
for (slave = 1; slave <= ec_slavecount; slave++) {
ec_slave[slave].state_request = EC_STATE_PRE_OPERATIONAL;
ec_writestate(slave);
}
// Close EtherCAT master
ec_close();
return 0;
}
```
这个代码示例假定只有一个从站连接到EtherCAT总线上,并且该从站是雷赛步进电机。在代码中,我们使用了EtherCAT库中的函数来初始化EtherCAT主站、配置从站和读写从站的寄存器。我们还使用了从站状态机来将从站设置为操作状态,并使用SDO(服务数据对象)来设置从站的工作模式和最大位置。我们还使用了一个循环来将电机移动到不同的位置,并在移动过程中打印当前位置。
请注意,这只是一个简单的示例代码,实际的应用程序可能需要更多的功能和错误处理。
阅读全文
相关推荐
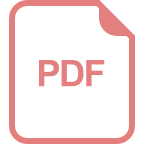
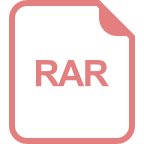






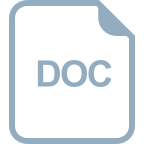


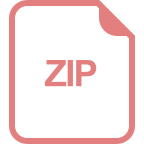
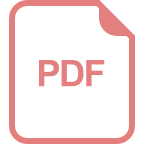
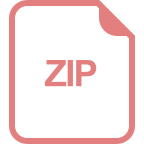
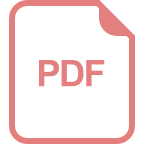
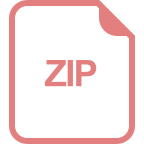

