igh ethercat程序汇川步进代码示例
时间: 2023-08-25 10:05:52 浏览: 321
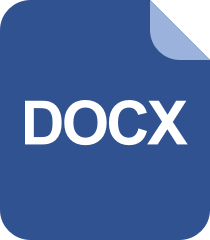
IGH ethercat 引用头文件进行了目录分类 ecrt文件.docx
以下是汇川步进电机使用EtherCAT通讯控制的示例代码:
```c
#include "ecrt.h"
#include <stdio.h>
#include <signal.h>
#include <unistd.h>
#define EC_TIMEOUTMON 500
#define VENDOR_ID 0x1d50
#define PRODUCT_CODE 0x60c0
#define POSITION_MODE 0x01
#define VELOCITY_MODE 0x03
#define PROFILE_POS_MODE 0x07
#define OPMODE_OFFSET 0x6060
#define OPMODE_SIZE 0x01
#define OPMODE_PROFILE_POS 0x01
#define OPMODE_HOMING 0x06
#define OPMODE_PROFILE_VELOCITY 0x03
#define OPMODE_INTERPOLATION_POS 0x0a
#define CONTROLWORD_OFFSET 0x6040
#define CONTROLWORD_SIZE 0x02
#define CONTROLWORD_SHUTDOWN 0x06
#define CONTROLWORD_SWITCH_ON 0x07
#define CONTROLWORD_DISABLE_VOLTAGE 0x00
#define CONTROLWORD_QUICK_STOP 0x02
#define CONTROLWORD_DISABLE_OPERATION 0x07
#define CONTROLWORD_ENABLE_OPERATION 0x0f
#define STATUSWORD_OFFSET 0x6041
#define STATUSWORD_SIZE 0x02
#define STATUSWORD_READY_TO_SWITCH_ON 0x0021
#define STATUSWORD_SWITCHED_ON 0x0023
#define STATUSWORD_OPERATION_ENABLED 0x0027
#define STATUSWORD_QUICK_STOP_ACTIVE 0x0007
#define STATUSWORD_FAULT 0x000f
#define TARGET_POS_OFFSET 0x607a
#define TARGET_POS_SIZE 0x04
#define TARGET_VELOCITY_OFFSET 0x60ff
#define TARGET_VELOCITY_SIZE 0x04
#define PROFILE_VELOCITY_OFFSET 0x6081
#define PROFILE_VELOCITY_SIZE 0x04
#define PROFILE_ACCELERATION_OFFSET 0x6083
#define PROFILE_ACCELERATION_SIZE 0x04
#define PROFILE_DECELERATION_OFFSET 0x6084
#define PROFILE_DECELERATION_SIZE 0x04
#define FOLLOWING_ERROR_OFFSET 0x60f4
#define FOLLOWING_ERROR_SIZE 0x04
#define DIGITAL_OUTPUT_OFFSET 0x2070
#define DIGITAL_OUTPUT_SIZE 0x01
#define DIGITAL_INPUT_OFFSET 0x2071
#define DIGITAL_INPUT_SIZE 0x01
#define MAXON_EPOS3_NAME "EPOS3"
#define MAXON_EPOS3_ALIAS "my_epos3"
#define MAXON_EPOS3_POSITION "position"
#define ECAT_CYCLE_TIME_NS 1000000
static volatile int run = 1;
static ec_master_t *master = NULL;
static ec_master_state_t master_state = {};
static ec_domain_t *domain1 = NULL;
static ec_domain_state_t domain1_state = {};
static ec_slave_config_t *maxon_epos3 = NULL;
static ec_slave_config_state_t maxon_epos3_state = {};
void signal_handler(int signum)
{
if (signum == SIGINT)
run = 0;
}
int main(int argc, char **argv)
{
if (ecrt_master_open(&master, "ETHERCAT_MASTER") != 0) {
printf("Failed to open EtherCAT master.\n");
return -1;
}
if (ecrt_master_get_state(master, &master_state) != 0) {
printf("Failed to get master state.\n");
return -1;
}
if (master_state.slaves_responding == 0) {
printf("No slaves found on EtherCAT bus.\n");
return -1;
}
domain1 = ecrt_master_create_domain(master, 1, 0);
if (domain1 == NULL) {
printf("Failed to create domain.\n");
return -1;
}
maxon_epos3 = ecrt_master_slave_config(master, VENDOR_ID, PRODUCT_CODE);
if (maxon_epos3 == NULL) {
printf("Failed to get slave config.\n");
return -1;
}
maxon_epos3->alias = MAXON_EPOS3_ALIAS;
maxon_epos3->position = MAXON_EPOS3_POSITION;
if (ecrt_slave_config_pdos(maxon_epos3, EC_END, maxon_epos3_syncs) != 0) {
printf("Failed to configure PDOs.\n");
return -1;
}
if (ecrt_domain_reg_pdo_entry_list(domain1, maxon_epos3_syncs)) {
printf("Failed to register PDO entry list.\n");
return -1;
}
if (ecrt_master_activate(master) != 0) {
printf("Failed to activate master.\n");
return -1;
}
printf("Initialization complete.\n");
// Set operation mode to profile position mode
uint8_t opmode = OPMODE_PROFILE_POS;
if (ecrt_sdo_write(maxon_epos3, OPMODE_OFFSET, 0x00, OPMODE_SIZE, &opmode) != 0) {
printf("Failed to set operation mode.\n");
return -1;
}
// Set control word to switch on
uint16_t controlword = CONTROLWORD_SWITCH_ON;
if (ecrt_sdo_write(maxon_epos3, CONTROLWORD_OFFSET, 0x00, CONTROLWORD_SIZE, &controlword) != 0) {
printf("Failed to set control word.\n");
return -1;
}
signal(SIGINT, signal_handler);
// Main loop
int64_t cycle_count = 0;
uint32_t target_pos = 100000;
uint32_t target_velocity = 2000;
uint32_t profile_velocity = 2000;
uint32_t profile_acceleration = 5000;
uint32_t profile_deceleration = 5000;
uint32_t following_error = 0;
uint8_t digital_output = 0;
uint8_t digital_input = 0;
while (run) {
ecrt_master_receive(master);
ecrt_domain_process(domain1);
cycle_count++;
if (cycle_count >= (1000000000 / ECAT_CYCLE_TIME_NS)) {
cycle_count = 0;
// Set target position
if (ecrt_sdo_write(maxon_epos3, TARGET_POS_OFFSET, 0x00, TARGET_POS_SIZE, &target_pos) != 0) {
printf("Failed to set target position.\n");
return -1;
}
// Set target velocity
if (ecrt_sdo_write(maxon_epos3, TARGET_VELOCITY_OFFSET, 0x00, TARGET_VELOCITY_SIZE, &target_velocity) != 0) {
printf("Failed to set target velocity.\n");
return -1;
}
// Set profile velocity
if (ecrt_sdo_write(maxon_epos3, PROFILE_VELOCITY_OFFSET, 0x00, PROFILE_VELOCITY_SIZE, &profile_velocity) != 0) {
printf("Failed to set profile velocity.\n");
return -1;
}
// Set profile acceleration
if (ecrt_sdo_write(maxon_epos3, PROFILE_ACCELERATION_OFFSET, 0x00, PROFILE_ACCELERATION_SIZE, &profile_acceleration) != 0) {
printf("Failed to set profile acceleration.\n");
return -1;
}
// Set profile deceleration
if (ecrt_sdo_write(maxon_epos3, PROFILE_DECELERATION_OFFSET, 0x00, PROFILE_DECELERATION_SIZE, &profile_deceleration) != 0) {
printf("Failed to set profile deceleration.\n");
return -1;
}
// Read following error
if (ecrt_sdo_read(maxon_epos3, FOLLOWING_ERROR_OFFSET, 0x00, FOLLOWING_ERROR_SIZE, &following_error) != 0) {
printf("Failed to read following error.\n");
return -1;
}
// Read digital output
if (ecrt_sdo_read(maxon_epos3, DIGITAL_OUTPUT_OFFSET, 0x00, DIGITAL_OUTPUT_SIZE, &digital_output) != 0) {
printf("Failed to read digital output.\n");
return -1;
}
// Write digital input
digital_input = !digital_output;
if (ecrt_sdo_write(maxon_epos3, DIGITAL_INPUT_OFFSET, 0x00, DIGITAL_INPUT_SIZE, &digital_input) != 0) {
printf("Failed to write digital input.\n");
return -1;
}
// Read status word
uint16_t statusword = 0;
if (ecrt_sdo_read(maxon_epos3, STATUSWORD_OFFSET, 0x00, STATUSWORD_SIZE, &statusword) != 0) {
printf("Failed to read status word.\n");
return -1;
}
// Check status word
if (statusword & STATUSWORD_FAULT) {
printf("Fault detected.\n");
run = 0;
}
else if ((statusword & STATUSWORD_SWITCHED_ON) && !(statusword & STATUSWORD_OPERATION_ENABLED)) {
// Set control word to enable operation
controlword = CONTROLWORD_ENABLE_OPERATION;
if (ecrt_sdo_write(maxon_epos3, CONTROLWORD_OFFSET, 0x00, CONTROLWORD_SIZE, &controlword) != 0) {
printf("Failed to set control word.\n");
return -1;
}
}
else if (statusword & STATUSWORD_OPERATION_ENABLED) {
printf("Target position: %d, Following error: %d\n", target_pos, following_error);
}
}
ecrt_domain_queue(domain1);
ecrt_master_send(master);
usleep(ECAT_CYCLE_TIME_NS / 1000);
}
// Set control word to shut down
controlword = CONTROLWORD_SHUTDOWN;
if (ecrt_sdo_write(maxon_epos3, CONTROLWORD_OFFSET, 0x00, CONTROLWORD_SIZE, &controlword) != 0) {
printf("Failed to set control word.\n");
return -1;
}
ecrt_master_deactivate(master);
ecrt_master_close(master);
return 0;
}
```
这是一个简单的示例程序,可以控制汇川步进电机进行位置控制。具体的功能和参数设置可以根据实际需要进行修改。
阅读全文
相关推荐
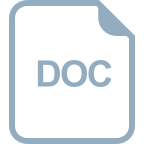
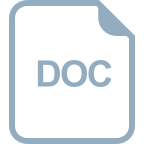















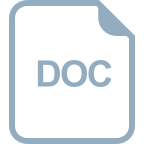