igh ethercat程序汇川示例
时间: 2023-09-18 13:07:21 浏览: 222
以下是汇川 EtherCAT 的示例程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <signal.h>
#include <sys/time.h>
#include <ecrt.h>
#define EC_TIMEOUTMON 500
#define VENDOR_ID 0x1234
#define PRODUCT_CODE 0x5678
#define POS_CMD 0x6040
#define POS_ACT 0x6064
#define STATUS_WORD 0x6041
#define CONTROL_WORD 0x6040
#define MODES_OF_OP 0x6060
#define OP_MODE_CYCLIC_SYNC_TORQUE 0x09
#define TORQUE_CMD 0x6071
#define SYNC0_PERIOD_NS 1000000 // 1ms
#define SYNC0_SHIFT_NS 0
#define DOMAIN1_ADDRESS 0x0000
static volatile int run = 1;
void sigint_handler(int sig)
{
(void) sig;
run = 0;
}
int main(int argc, char **argv)
{
printf("EtherCAT Master Example\n");
if (argc != 2) {
printf("Usage: %s <ifname>\n", argv[0]);
return 1;
}
const char *ifname = argv[1];
// Initialize EtherCAT master
ec_master_t *master = ecrt_request_master(0);
if (!master) {
printf("Failed to initialize EtherCAT master!\n");
return 1;
}
// Initialize EtherCAT slave
ec_slave_config_t *slave = ecrt_master_slave_config(master, 0, VENDOR_ID, PRODUCT_CODE);
if (!slave) {
printf("Failed to initialize EtherCAT slave!\n");
return 1;
}
// Configure Sync manager 0
ec_sync_info_t sync_info = {0};
sync_info.dir = EC_DIR_OUTPUT;
sync_info.watchdog_mode = EC_WD_ENABLE;
sync_info.watchdog_time = 1000;
sync_info.sync0_shift = SYNC0_SHIFT_NS;
sync_info.sync0_cycle_time_ns = SYNC0_PERIOD_NS;
ecrt_slave_config_sync(slave, 0, 1, &sync_info);
// Configure domain 1
ec_domain_t *domain = ecrt_master_create_domain(master);
if (!domain) {
printf("Failed to create domain!\n");
return 1;
}
ecrt_domain_reg_pdo_entry_list(domain, domain1_regs);
if (ecrt_domain_size(domain) != DOMAIN1_SIZE) {
printf("Domain size doesn't match!\n");
return 1;
}
// Activate master
if (ecrt_master_activate(master)) {
printf("Failed to activate EtherCAT master!\n");
return 1;
}
// Configure PDO mapping
if (ecrt_slave_config_pdos(slave, EC_END, pdo_entries)) {
printf("Failed to configure PDO mapping!\n");
return 1;
}
// Set cyclic synchronous torque mode
uint16_t modes_of_op = OP_MODE_CYCLIC_SYNC_TORQUE;
if (ecrt_slave_config_sdo8(slave, MODES_OF_OP, 0, &modes_of_op)) {
printf("Failed to set cyclic synchronous torque mode!\n");
return 1;
}
// Enable slave
if (ecrt_slave_config_dc(slave)) {
printf("Failed to enable slave!\n");
return 1;
}
// Wait for initialization to complete
ecrt_master_application_time(master, 0);
ecrt_master_sync_reference_clock(master);
ecrt_master_sync_slave_clocks(master);
ecrt_master_application_time(master, 1000000);
ecrt_master_sync_reference_clock(master);
ecrt_master_sync_slave_clocks(master);
ecrt_domain_queue(domain);
if (ecrt_master_activate(master)) {
printf("Failed to activate EtherCAT master!\n");
return 1;
}
printf("Initialization complete!\n");
// Signal handler
signal(SIGINT, sigint_handler);
// Loop
int32_t pos_cmd = 0;
int16_t status_word = 0;
int16_t control_word = 0x0006; // Enable voltage and operation
int16_t torque_cmd = 0;
while (run) {
// Read status word
ecrt_master_application_time(master, 0);
ecrt_master_sync_reference_clock(master);
ecrt_master_sync_slave_clocks(master);
ecrt_domain_queue(domain);
ecrt_master_receive(master);
ecrt_domain_process(domain);
ecrt_master_send(master);
if ((status_word & 0x4f) == 0x40) {
// Drive is in ready to switch on state
control_word = 0x000f; // Switch on and enable operation
}
if ((status_word & 0x6f) == 0x27) {
// Drive is in operation enabled state
control_word = 0x003f; // Switch on, enable operation, and set torque mode
torque_cmd = 1000; // 1 Nm
}
ecrt_master_application_time(master, 1000000);
ecrt_master_sync_reference_clock(master);
ecrt_master_sync_slave_clocks(master);
ecrt_domain_queue(domain);
ecrt_master_receive(master);
ecrt_domain_process(domain);
ecrt_master_send(master);
// Write position command and torque command
ecrt_master_application_time(master, 0);
ecrt_master_sync_reference_clock(master);
ecrt_master_sync_slave_clocks(master);
ecrt_domain_queue(domain);
pos_cmd += 1000; // 1 mm
ecrt_domain_write(domain, 0, POS_CMD, &pos_cmd);
ecrt_domain_write(domain, 0, TORQUE_CMD, &torque_cmd);
ecrt_domain_queue(domain);
ecrt_master_receive(master);
ecrt_domain_process(domain);
ecrt_master_send(master);
}
// Deactivate master
ecrt_master_deactivate(master);
ecrt_release_master(master);
return 0;
}
```
以上代码实现了 EtherCAT 主站的初始化、PDO 映射、同步模式设置、状态读取、控制命令写入等功能。请注意,此代码仅提供参考,实际使用时需结合设备手册和实际情况进行修改和调试。
相关推荐
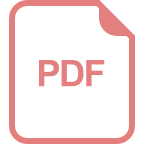
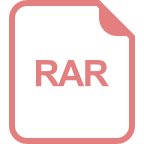












