igh ethercat程序代码示例
时间: 2023-08-24 17:09:58 浏览: 110
以下是一个简单的 EtherCAT 程序代码示例,以使用 Beckhoff TwinCAT 为例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <conio.h>
#include "ecatappl.h"
#define VENDOR_ID 0x0002
#define PRODUCT_CODE 0x0001
#define REVISION_NUMBER 0x0001
int main(int argc, char* argv[])
{
int result = 0;
ECAT_APP_MASTER_CONFIG masterConfig = { 0 };
ECAT_APP_SLAVE_CONFIG slaveConfig = { 0 };
ECAT_APP_SLAVE_INFO slaveInfo = { 0 };
ECAT_APP_SLAVE_IO_MAP ioMap = { 0 };
// 初始化 EtherCAT 应用程序
result = ecatAppInit();
if (result != ECAT_APP_RESULT_SUCCESS)
{
printf("Failed to initialize EtherCAT application.\n");
return -1;
}
// 配置 EtherCAT 主站
masterConfig.vendorId = VENDOR_ID;
masterConfig.productCode = PRODUCT_CODE;
masterConfig.revisionNumber = REVISION_NUMBER;
result = ecatAppConfigureMaster(&masterConfig);
if (result != ECAT_APP_RESULT_SUCCESS)
{
printf("Failed to configure EtherCAT master.\n");
ecatAppDeinit();
return -2;
}
// 启动 EtherCAT 主站
result = ecatAppStartMaster();
if (result != ECAT_APP_RESULT_SUCCESS)
{
printf("Failed to start EtherCAT master.\n");
ecatAppDeinit();
return -3;
}
// 配置 EtherCAT 从站
slaveConfig.vendorId = VENDOR_ID;
slaveConfig.productCode = PRODUCT_CODE;
slaveConfig.revisionNumber = REVISION_NUMBER;
result = ecatAppConfigureSlave(&slaveConfig, &slaveInfo);
if (result != ECAT_APP_RESULT_SUCCESS)
{
printf("Failed to configure EtherCAT slave.\n");
ecatAppStopMaster();
ecatAppDeinit();
return -4;
}
// 映射 EtherCAT 从站 I/O
ioMap.inputSize = slaveInfo.inputSize;
ioMap.outputSize = slaveInfo.outputSize;
ioMap.inputData = malloc(slaveInfo.inputSize);
ioMap.outputData = malloc(slaveInfo.outputSize);
result = ecatAppMapSlaveIO(&ioMap);
if (result != ECAT_APP_RESULT_SUCCESS)
{
printf("Failed to map EtherCAT slave I/O.\n");
free(ioMap.inputData);
free(ioMap.outputData);
ecatAppStopMaster();
ecatAppDeinit();
return -5;
}
// 读取 EtherCAT 从站输入
result = ecatAppReadSlaveInput(ioMap.inputData, ioMap.inputSize);
if (result != ECAT_APP_RESULT_SUCCESS)
{
printf("Failed to read EtherCAT slave input.\n");
free(ioMap.inputData);
free(ioMap.outputData);
ecatAppStopMaster();
ecatAppDeinit();
return -6;
}
// 写入 EtherCAT 从站输出
result = ecatAppWriteSlaveOutput(ioMap.outputData, ioMap.outputSize);
if (result != ECAT_APP_RESULT_SUCCESS)
{
printf("Failed to write EtherCAT slave output.\n");
free(ioMap.inputData);
free(ioMap.outputData);
ecatAppStopMaster();
ecatAppDeinit();
return -7;
}
// 释放 EtherCAT 从站 I/O 映射
free(ioMap.inputData);
free(ioMap.outputData);
// 停止 EtherCAT 主站
ecatAppStopMaster();
// 释放 EtherCAT 应用程序
ecatAppDeinit();
return 0;
}
```
注意,以上代码仅为示例,实际情况下需要根据具体的 EtherCAT 系统配置和要求进行修改。
阅读全文
相关推荐
















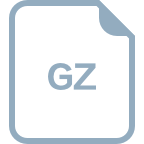