igh ethercat程序汇川代码示例
时间: 2023-08-25 11:05:50 浏览: 86
以下是汇川的IGH EtherCAT程序示例:
```c++
#include "stdafx.h"
#include <Windows.h>
#include <tchar.h>
#include <ecrt.h>
#include <iostream>
#include <vector>
#define EC_TIMEOUTMON 500
#define VENDOR_ID 0x1A05 // Vendor ID of EtherCAT device
#define PRODUCT_CODE 0x0003 // Product code of EtherCAT device
#define NSEC_PER_SEC 1000000000
// EtherCAT domain
#define DOMAIN_INDEX 0 // Domain index
#define DOMAIN_SYNC0_PERIOD 1000000 // Sync0 period (ns)
#define DOMAIN_SYNC1_CYCLE 0 // Sync1 cycle (ns)
// EtherCAT slave
#define SLAVE_COUNT 1 // Number of EtherCAT slaves
#define SLAVE_POSITION 0 // Slave position in the EtherCAT network
#define SLAVE_VENDOR_ID 0x1A05 // Vendor ID of EtherCAT slave
#define SLAVE_PRODUCT_ID 0x0003 // Product code of EtherCAT slave
#define SLAVE_ALIAS 0 // Slave alias (0 for no alias)
// EtherCAT PDO mapping
#define PDO_MAPPING_COUNT 4 // Number of PDO mappings
#define PDO_MAPPING_RX_POS 0 // PDO mapping index for receive data
#define PDO_MAPPING_RX_COUNT 3 // Number of receive data PDO mapping
#define PDO_MAPPING_RX_OFFSET 0 // Offset of receive data PDO mapping
#define PDO_MAPPING_TX_POS 1 // PDO mapping index for transmit data
#define PDO_MAPPING_TX_COUNT 3 // Number of transmit data PDO mapping
#define PDO_MAPPING_TX_OFFSET 0 // Offset of transmit data PDO mapping
// EtherCAT slave registers
#define REG_OUTPUT 0x6000 // Output register address
#define REG_INPUT 0x7000 // Input register address
// EtherCAT slave PDO mapping
struct SlavePDO
{
uint8_t status;
uint16_t position;
int16_t velocity;
};
// EtherCAT domain and slave
static ec_master_t *master = NULL;
static ec_domain_t *domain = NULL;
static ec_slave_config_t *slave_config = NULL;
// EtherCAT slave PDO mapping
static SlavePDO pdo_rx;
static SlavePDO pdo_tx =
{
0,
0,
0
};
// EtherCAT domain and slave initialization
static bool InitEtherCAT()
{
// Initialize EtherCAT master
if (!ecrt_master_init())
{
std::cout << "Failed to initialize EtherCAT master." << std::endl;
return false;
}
// Get the first available EtherCAT master
if (!(master = ecrt_master_find_first()))
{
std::cout << "Failed to find EtherCAT master." << std::endl;
return false;
}
// Create an EtherCAT domain
if (!(domain = ecrt_domain_create()))
{
std::cout << "Failed to create EtherCAT domain." << std::endl;
return false;
}
// Set the EtherCAT domain synchronization
ecrt_domain_set_sync0_period(domain, DOMAIN_SYNC0_PERIOD);
ecrt_domain_set_sync1_cycle(domain, DOMAIN_SYNC1_CYCLE);
ecrt_master_set_send_interval(master, ecrt_domain_get_send_interval(domain));
// Create an EtherCAT slave configuration
slave_config = ecrt_master_slave_config_create(master, SLAVE_POSITION, SLAVE_VENDOR_ID, SLAVE_PRODUCT_ID);
// Set the EtherCAT slave alias
ecrt_slave_config_set_alias(slave_config, SLAVE_ALIAS);
// Set the EtherCAT slave PDO mapping
ec_pdo_entry_reg_t pdo_rx_entries[] =
{
{ REG_INPUT + 0, 0x0000, EC_SIZE_BYTE, &pdo_rx.status },
{ REG_INPUT + 1, 0x0000, EC_SIZE_WORD, &pdo_rx.position },
{ REG_INPUT + 3, 0x0000, EC_SIZE_WORD, &pdo_rx.velocity },
};
ec_pdo_entry_reg_t pdo_tx_entries[] =
{
{ REG_OUTPUT + 0, 0x0000, EC_SIZE_BYTE, &pdo_tx.status },
{ REG_OUTPUT + 1, 0x0000, EC_SIZE_WORD, &pdo_tx.position },
{ REG_OUTPUT + 3, 0x0000, EC_SIZE_WORD, &pdo_tx.velocity },
};
ec_pdo_info_t pdo_rx_info =
{
PDO_MAPPING_RX_POS,
PDO_MAPPING_RX_COUNT,
pdo_rx_entries,
};
ec_pdo_info_t pdo_tx_info =
{
PDO_MAPPING_TX_POS,
PDO_MAPPING_TX_COUNT,
pdo_tx_entries,
};
ec_sync_info_t sync_info[] =
{
{
EC_SYNC_INFO(0),
EC_DIR_INPUT,
1,
&pdo_rx_info,
EC_WD_DISABLE,
},
{
EC_SYNC_INFO(1),
EC_DIR_OUTPUT,
1,
&pdo_tx_info,
EC_WD_DISABLE,
},
{ 0 },
};
if (ecrt_slave_config_pdos(slave_config, EC_END, sync_info))
{
std::cout << "Failed to set EtherCAT slave PDO mapping." << std::endl;
return false;
}
// Register the EtherCAT slave configuration
if (ecrt_master_slave_config_add(master, slave_config))
{
std::cout << "Failed to add EtherCAT slave configuration." << std::endl;
return false;
}
// Register the EtherCAT domain for the EtherCAT slave configuration
if (ecrt_domain_reg_pdo_entry_list(domain, sync_info))
{
std::cout << "Failed to register EtherCAT domain." << std::endl;
return false;
}
// Activate the EtherCAT master
if (ecrt_master_activate(master))
{
std::cout << "Failed to activate EtherCAT master." << std::endl;
return false;
}
// Initialize the EtherCAT slave
if (ecrt_slave_config_sdo8(slave_config, 0x60FF, 0x00, 0x01))
{
std::cout << "Failed to initialize EtherCAT slave." << std::endl;
return false;
}
return true;
}
// EtherCAT domain and slave deinitialization
static void DeinitEtherCAT()
{
// Deactivate the EtherCAT master
ecrt_master_deactivate(master);
// Unregister the EtherCAT domain for the EtherCAT slave configuration
ecrt_domain_unreg_pdo_entry_list(domain, ecrt_domain_reg_pdo_entry_list(domain, NULL));
// Unregister the EtherCAT slave configuration
ecrt_master_slave_config_remove(master, slave_config);
// Delete the EtherCAT slave configuration
ecrt_master_slave_config_delete(slave_config);
// Delete the EtherCAT domain
ecrt_domain_delete(domain);
// Deinitialize the EtherCAT master
ecrt_master_deinit(master);
}
// EtherCAT slave read
static void ReadSlave()
{
// Read the EtherCAT slave PDO mapping
ecrt_master_receive(master);
ecrt_domain_process(domain);
ecrt_master_send(master);
// Get the EtherCAT slave PDO mapping
pdo_rx.status = ecrt_slave_config_reg_read_u8(slave_config, REG_INPUT + 0);
pdo_rx.position = ecrt_slave_config_reg_read_u16(slave_config, REG_INPUT + 1);
pdo_rx.velocity = ecrt_slave_config_reg_read_s16(slave_config, REG_INPUT + 3);
}
// EtherCAT slave write
static void WriteSlave()
{
// Set the EtherCAT slave PDO mapping
pdo_tx.status = 0x01;
pdo_tx.position = 0x0100;
pdo_tx.velocity = 0x0200;
ecrt_slave_config_reg_write_u8(slave_config, REG_OUTPUT + 0, pdo_tx.status);
ecrt_slave_config_reg_write_u16(slave_config, REG_OUTPUT + 1, pdo_tx.position);
ecrt_slave_config_reg_write_s16(slave_config, REG_OUTPUT + 3, pdo_tx.velocity);
// Write the EtherCAT slave PDO mapping
ecrt_master_receive(master);
ecrt_domain_process(domain);
ecrt_master_send(master);
}
// Main function
int _tmain(int argc, _TCHAR* argv[])
{
// Initialize EtherCAT domain and slave
if (!InitEtherCAT())
{
return 1;
}
// Loop until the program is terminated
while (true)
{
// Read the EtherCAT slave
ReadSlave();
// Write the EtherCAT slave
WriteSlave();
// Sleep for a short time
Sleep(1);
}
// Deinitialize EtherCAT domain and slave
DeinitEtherCAT();
return 0;
}
```
该示例初始化一个IGH EtherCAT主机,配置一个EtherCAT从机,设置PDO映射,读取和写入PDO数据,并在无限循环中重复此操作。注意,此示例仅适用于一个从机。如果您需要连接多个从机,请相应地调整代码。
相关推荐
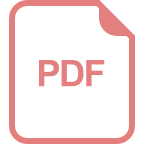
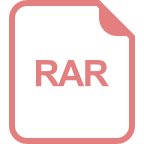














