pygame 写贪吃蛇
时间: 2023-07-10 14:38:24 浏览: 47
下面是一个简单的 Pygame 贪吃蛇示例代码:
```python
import pygame
import random
# 初始化 Pygame
pygame.init()
# 设置游戏窗口尺寸
WINDOW_WIDTH = 640
WINDOW_HEIGHT = 480
WINDOW_SIZE = (WINDOW_WIDTH, WINDOW_HEIGHT)
# 设置游戏标题
pygame.display.set_caption("Snake Game")
# 定义游戏颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
GREEN = (0, 255, 0)
RED = (255, 0, 0)
# 设置游戏时钟
clock = pygame.time.Clock()
# 初始化游戏对象
class Snake:
def __init__(self):
self.size = 20
self.x = 0
self.y = 0
self.speed = self.size
self.direction = 'right'
self.body = []
def move(self):
if self.direction == 'right':
self.x += self.speed
elif self.direction == 'left':
self.x -= self.speed
elif self.direction == 'up':
self.y -= self.speed
elif self.direction == 'down':
self.y += self.speed
self.body.insert(0, (self.x, self.y))
def change_direction(self, direction):
if direction == 'right' and self.direction != 'left':
self.direction = 'right'
elif direction == 'left' and self.direction != 'right':
self.direction = 'left'
elif direction == 'up' and self.direction != 'down':
self.direction = 'up'
elif direction == 'down' and self.direction != 'up':
self.direction = 'down'
def draw(self, surface):
for x, y in self.body:
pygame.draw.rect(surface, GREEN, (x, y, self.size, self.size))
def check_collision(self, apple):
if self.x == apple.x and self.y == apple.y:
return True
else:
return False
class Apple:
def __init__(self):
self.size = 20
self.x = random.randint(0, WINDOW_WIDTH - self.size)
self.y = random.randint(0, WINDOW_HEIGHT - self.size)
def draw(self, surface):
pygame.draw.rect(surface, RED, (self.x, self.y, self.size, self.size))
# 创建游戏窗口
screen = pygame.display.set_mode(WINDOW_SIZE)
# 初始化游戏对象
snake = Snake()
apple = Apple()
# 游戏循环
while True:
# 处理游戏事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
quit()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_RIGHT:
snake.change_direction('right')
elif event.key == pygame.K_LEFT:
snake.change_direction('left')
elif event.key == pygame.K_UP:
snake.change_direction('up')
elif event.key == pygame.K_DOWN:
snake.change_direction('down')
# 移动贪吃蛇
snake.move()
# 检查贪吃蛇是否吃到苹果
if snake.check_collision(apple):
apple = Apple()
# 绘制游戏场景
screen.fill(BLACK)
snake.draw(screen)
apple.draw(screen)
# 更新屏幕显示
pygame.display.update()
# 控制游戏帧率
clock.tick(10)
```
这个示例代码创建了一个简单的 Pygame 贪吃蛇游戏,玩家可以使用方向键来控制贪吃蛇的移动方向,同时贪吃蛇会不停地移动,直到吃到苹果为止。如果贪吃蛇吃到了苹果,就会重新生成一个新的苹果。这个示例代码可以帮助你了解 Pygame 的基本用法,同时也可以作为你创建自己的 Pygame 贪吃蛇游戏的基础。
相关推荐
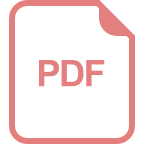
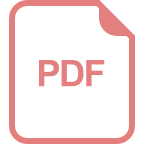














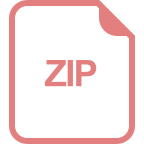